Question
The Random class is in the java.util package. It implements a pseudo-random number generator. One of its methods is: nextInt(int num) which returns a random
The Random class is in the java.util package. It implements a pseudo-random number generator. One of its methods is: nextInt(int num) which returns a random integer (any one of the possible values 0..num-1). Look at pages 112-115 of the text. The following program simulates flipping a coin:
import java.util.*;
class Flip
{ public static void main ( String[] args )
{ Scanner scan = new Scanner( System.in );
Random coin = new Random();
int count = 1, heads = 0, tails = 0;
int numberFlips, flipResult;
System.out.print("Enter the number of flips:");
numberFlips = scan.nextInt();
while( count <= numberFlips )
{ flipResult = coin.nextInt(2) ;
if ( flipResult == 0 )
heads++ ;
else tails++ ;
count++ ; }
System.out.println("The number of heads:" + heads );
System.out.println("The number of tails:" + tails );
}
}
Your job: Modify the program so that it simulates an 8-sided die. Rather than heads and tails, use an array of 8 int. Be sure to initialize the array to zero. After each throw of the die, increment the correct cell. At the end of the program print the value in each cell.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
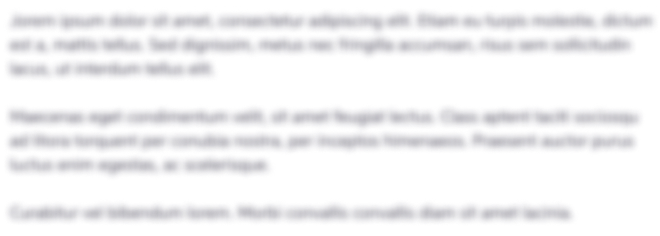
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started