Question
The Requirement (What you need to do) You are asked to write a program that reads in a sequence of expenditure record, stores them, sorts
The Requirement (What you need to do)
You are asked to write a program that reads in a sequence of expenditure record, stores them, sorts the records based upon the dollar amount in increasing order, and then reports the total and median expenditure amount.
Use case (Scenario)
$RecordKeeper Enter the expenditure record (e.g., $1.95, coffee, enter 0.0 to end):$8.45 Enter the expenditure record (e.g., $1.95, coffee, enter 0.0 to end):$45.03 Enter the expenditure record (e.g., $1.95, coffee, enter 0.0 to end):$2.03 Enter the expenditure record (e.g., $1.95, coffee, enter 0.0 to end):$0.0 Sorted list of expenditure: $2.03 $8.45 $45.03 The total is $55.51 (Fifty five and 51/100). The median is $8.45. Bye!
Error handling: You are required to handle the following error inputs. If the input is any of the following case, your program should display appropriate error message, and ask the user to try again.
- The dollar amount cannot be greater than 9999.99
- The dollar amount cannot be negative
- There are at most two decimal digitals in the input
- Handle non-digit input, such as ten, for the dollar amount
Details
The following are more detailed requirement/hints with regards to how to implement the program:
- (If you haven't done so in lab1) Design DollarAmount class
- What are the attributes of the object? What operations do you need to provide for object of this type?
- Write the class declaraion (i.e., class header file).
- Implement the class.
- Test the class
- overload input operator (>>) (if you haven't done so in LAB1) (this includes your previous code that handles errors in input).
- overload output operator (<<) (if you haven't done so in LAB1), which displays the amount in the format of $234.54.
- overload addition operator +, to be used to calculate the total amount
- overload greater-than operator > (so that we can use the template sort function, which uses >)
- In the driver program main() function, dynamically allocate an array of DollarAmount (or SpendingRecord if you work on the above extra credit version) of a certain initial size, enter a loop to read input (until 0.0 is entered). If the array is filled up, you need to grow the array (by allocating a new array of larger size, copying existing elements into the new array).
const int INIT_SIZE=10; int main() { ... DollarAmount * arr = new DollarAmount[INIT_SIZE]; DollarAmount * ptr=NULL; int arr_size=INIT_SIZE: int arr_len=0; // actual number of objects stored in it , also the index // of next free slot in arr bool lastInput = false; do { if (arr_len==arr_size) { // the array is full, need to grow! //allocate an array double the cur_size ptr = new DollarAmount[arr_size*2]; //copy each element in arr into the new array that ptr points to... // Todo: please figure out how to do this...(hint: use a for loop) //now we delete the current arr delete [] arr; arr = ptr; arr_size = arr_size*2; } cout <<"Enter the dollar amount:$"; cin >> arr[arr_len]; //read in a dollar amount from cin //We will allow input such as 0.0 //If the last read dollar amount is 0.0, then it's the end if (arr[arr_len].getDollar()==0 && arr[arr_len].getCent()==0 ) lastInput = true; else //we only increement arr_len for input that's not 0.0 arr_len++; } while (!lastInput); //A loopt to add all DollarAmount up, and display the total //Call Sort function to sort the array of DollarAmount //Display the sorted array, and the median }
After the user finishes with the input, the progaram calculates and displays the total dollar amount, then sorts the array, displays the sorted array, and the median dollar amount. - In this sample code, the bubble sorting algorithm is illustrated. Based upon the sort function inside, write a template sort function that can be used to sort array of objects. The function can be put in the driver program. dynamically allocate the array and enlarge the array if needed. -->
- [extra credit] Build a class named SpendingRecord to represent a single expenditure record, which includes both the dollar amount information and the description information. You should do so by either 1) inheriting from the class DollarAmount, or 2) including a member variable of type DollarAmount in the class. For the SpendingRecord class, implement the following:
- constructor
- overload greater-than operator > if needed (Do you?)
- input operator, output operator
$RecordKeeper Enter the expenditure record (e.g., $1.95, coffee, enter -1 to end):$8.45 lunch Enter the expenditure record (e.g., $1.95, coffee, enter -1 to end):$45.03 Data Structure Book Enter the expenditure record (e.g., $1.95, coffee, enter -1 to end):$2.03 coffee Enter the expenditure record (e.g., $1.95, coffee, enter -1 to end):$-1 ...
Step by Step Solution
There are 3 Steps involved in it
Step: 1
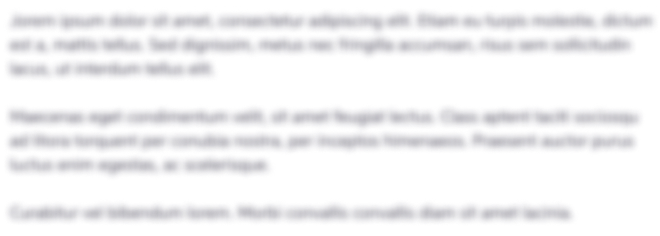
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started