Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The requirements are in the files First File import java.util.ArrayList; import java.util.LinkedList; import java.util.function.BiFunction; // ------------------------------------------------------- /** * * An exception to throw when attempting
The requirements are in the files
First File
import java.util.ArrayList; import java.util.LinkedList; import java.util.function.BiFunction; // ------------------------------------------------------- /** * * An exception to throw when attempting to insert elements into a * full hash table. * */ class TableFullE extends Exception {} // ------------------------------------------------------- /** * * The abstract class for the four hash tables we will implement. The * file HashTableTest has four test cases that should produce the same * information as Figures 5.5, 5.11, 5.13, and 5.18 in the book. You * should implement many more test cases!!! * */ abstract class HashTable { abstract void insert (int key) throws TableFullE; abstract void delete (int key); abstract boolean search (int key); } // ------------------------------------------------------- /** * * An implementation of a hash table that uses separate chaining. The * constructor should take two arguments: an argument 'size' of type * int, and an argument 'hf' of type HashFunction. The constructor * should create an ArrayList of the given size and initialize each * entry in that ArrayList to an empty linked list. The three methods * should be implemented as described in the book. You should also * implement a toString method that returns a comprehensive string * showing the entire contents of the hash table for debugging and * testing purposes. * */ class HashSeparateChaining extends HashTable { private int size; private HashFunction hf; ArrayListchains; HashSeparateChaining(int size, HashFunction hf){ this.size = size; this.hf = hf; chains = new ArrayList<>(size); for(int i = 0; i< size; i++){ chains.add(i, new LinkedList()); } } @Override void insert(int key){ int h = hf.apply(key); LinkedList list = chains.get(h); if(!list.contains(h)){ list.add(key); } } @Override void delete(int key) { int h = hf.apply(key); chains.get(h).remove(Integer.valueOf(key)); } @Override boolean search(int key){ int h = hf.apply(key); return chains.contains(h); } } // ------------------------------------------------------- /** * * Before implementing the next three variants of hash tables, we will * implement a small class hierarchy of hash tables entries. There are * three kinds of entries: an entry that contains an 'int' value, an * entry that is empty and hence available, and an entry that is * marked as deleted. A deleted entry is available for insertions but * cannot be marked as empty. See the book for details. * */ abstract class Entry { abstract boolean available (); } /** * * An instance of this class represents an entry that is * available. Don't forget to also implement a toString method. * */ class Empty extends Entry { public String toString () { return ""; } } /** * * An instance of this class represents an entry that is * available. Don't forget to also implement a toString method. * */ class Deleted extends Entry { public String toString () { return "X"; } } } /** * * A constructor of this class takes an 'int' representing a value * stored in the hash table. That entry is not available. Also don't * forget to implement a toString method. * */ class Value extends Entry { private int i; public String toString () { return String.valueOf(this.i); } } // ------------------------------------------------------- /** * * This class, although abstract, will have a constructor and an * implementation of each of the three methods: insert, delete, and * search. (Also don't forget a toString method.) The constructor * should take three arguments: an argument 'size' of type int, an * argument 'hf' of type HashFunction, and an argument 'f' of type * BiFunction ,Integer,Integer>. The constructor should create * an ArrayList of the given size and initialize each slot in that * array with an Empty entry. The construct should also define a * BiFunction (key))){ return true; } } return false; } public String toString () { String result = ""; for (int i=0; i,Integer,Integer> called 'dhf' as follows: * * this.dhf = (key,index) -> (hf.apply(key) + f.apply(key,index)) % size; * * This auxiliary hash function applies the regular hash function 'hf' * and then modifies the result using the BiFunction 'f' that depends * on the current index in the hash table. The subclasses for linear * probing, quadratic probing, and double hashing, will each pass an * appropriate function 'f' to control the auxiliary function. * * Each of the methods insert, delete, and search takes a value 'key' * to process. Each of the methods will involve a loop that iterates * over all the positions in the ArrayList. At iteration 'i', an * integer position is calculated using: * * int h = dhf.apply(key,i) * * For insert: if the position 'h' is available then the value 'key' * is stored. * * For delete: if position 'h' has an entry of kind 'Value' and if the * stored integer is identical to 'key' then the entry is marked as * deleted. * * For search: if position 'h' is Empty then the item is not found. If * position 'h' has an entry of kind 'Value' and if the stored value * is identical to 'key' then the item is found. * */ abstract class HashTableAux extends HashTable { int size; HashFunction hf; BiFunction (key))){ aux.add(h,new Deleted()); } } } public boolean search(int key) { for(int i =0; if; BiFunction dhf; ArrayList aux; HashTableAux(int size, HashFunction hf, BiFunction f){ this.size =size; this.hf =hf; this.f =f; dhf =(key,index)->(hf.apply(key)+f.apply(key,index))%size; aux = new ArrayList<>(size); for(int i = 0; i valueOf valueOf /** * * The only code in this class should be a constructor that takes two * arguments: an argument 'size' of type int and an argument 'hf' of * type HashFunction. * */ class HashLinearProbing extends HashTableAux { } // ------------------------------------------------------- /** * * The only code in this class should be a constructor that takes two * arguments: an argument 'size' of type int and an argument 'hf' of * type HashFunction. * */ class HashQuadProbing extends HashTableAux { } // ------------------------------------------------------- /** * * The only code in this class should be a constructor that takes * three arguments: an argument 'size' of type int, an argument 'hf' * of type HashFunction, and a third argument 'hf2' represents the * secondary hash function. * */ class HashDouble extends HashTableAux { } // -------------------------------------------------------
test file
import org.junit.Test; import java.util.Random; import static org.junit.Assert.assertFalse; import static org.junit.Assert.assertTrue; public class HashTableTest { @Test public void fig55() throws TableFullE { HashFunction hf = new HashMod(10); HashTable ht = new HashSeparateChaining(10, hf); ht.insert(0); ht.insert(81); ht.insert(64); ht.insert(49); ht.insert(9); ht.insert(36); ht.insert(25); ht.insert(16); ht.insert(4); ht.insert(1); System.out.println("Fig. 5.5"); System.out.println(ht); } @Test public void fig511 () throws TableFullE { HashFunction hf = new HashMod(10); HashTable ht = new HashLinearProbing(10, hf); ht.insert(89); ht.insert(18); ht.insert(49); ht.insert(58); ht.insert(69); System.out.println("Fig. 5.11"); System.out.println(ht); } @Test public void fig513 () throws TableFullE { HashFunction hf = new HashMod(10); HashTable ht = new HashQuadProbing (10, hf); ht.insert(89); ht.insert(18); ht.insert(49); ht.insert(58); ht.insert(69); System.out.println("Fig. 5.13"); System.out.println(ht); } @Test public void fig518 () throws TableFullE { HashFunction hf = new HashMod(10); HashFunction hf2 = new HashModThen(7, h -> 7 - h); HashTable ht = new HashDouble (10, hf, hf2); ht.insert(89); ht.insert(18); ht.insert(49); ht.insert(58); ht.insert(69); System.out.println("Fig. 5.18"); System.out.println(ht); } }
plz help me solve this problem in java
Step by Step Solution
There are 3 Steps involved in it
Step: 1
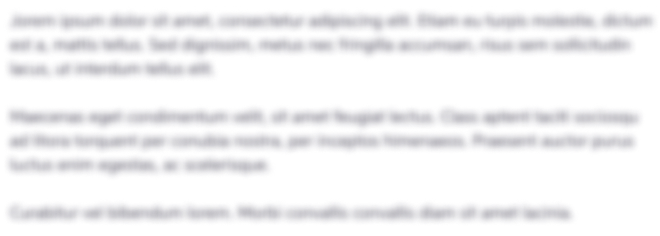
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started