Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The skeleton needs filled in A program skeleton (TicTacToeStarter.java) has been provided to help you write your program. The skeleton includes two class variables that
The skeleton needs filled in A program skeleton (TicTacToeStarter.java) has been provided to help you write your program. The skeleton includes two class variables that are visible to all of the programs methods: a Scanner used to read user input from the keyboard and a char array of size nine used to represent the tic tac toe board. In all, the program consists of eleven methods: main, playGame, initializeGameBoard, processComputerMove, processHumanMove, displayGameBoard, isDone, isComputerWin, isHumanWin, isPlayerWin, and isTie. A few of these already have some code in them, but most dont. All of them contain instructions on what you need to do in these methods import java.util.Random; import java.util.Scanner; public class TicTacToeStarter { private static Scanner keyboard = new Scanner(System.in); private static char[] board = new char[9]; // ******************************************************* public static void main(String[] args) { /* * Welcome the user to the game. Then ask the user if they would like to play a * game. * * As long as the user continues to answer yes, repeatedly do the following: (1) * call the playGame method (2) ask if they want to play again. * * When the user is done playing games, say goodbye to the user */ // <--- YOUR CODE HERE ---> } // ******************************************************* private static void playGame() { /* * Ask the player who should move first. Do this repeatedly until the user * responds with the letter c or the letter h. The user can enter either lower * or upper case, and you should handle converting the response to lower case. */ // <--- YOUR CODE HERE ---> /* * Set the following variable equal to 'c' or 'h' based on the user's response * above */ char currentPlayer = ' '; /* * Now call the initializeGameBoard method. */ // <--- YOUR CODE HERE ---> /* * The following code is the main loop for the game Don't change this code! */ while (!(isDone())) { if (currentPlayer == 'c') { processComputerMove(); currentPlayer = 'h'; } else { displayGameBoard(); processHumanMove(); currentPlayer = 'c'; } } /* * Display the game board one final time to tell the user the game is over. */ // <--- YOUR CODE HERE ---> } // ******************************************************* private static void initializeGameBoard() { /* * Set each cell of the board array to contain a space (i.e., the character ' * '). */ // <--- YOUR CODE HERE ---> } // ******************************************************* private static void processComputerMove() { /* * Create a loop that randomly selects a position on the board. This will be a * number from 0 to 8 which corresponds to a cell in the board array. * * If the selected cell is empty (i.e., contains a space), put an 'X' in the * cell (the computer always plays 'X'). Otherwise, randomly select another * number and try again. * * This is a good situation for a Do-While loop. */ // <--- YOUR CODE HERE ---> } // ******************************************************* private static void processHumanMove() { /* * Ask the user to enter the number of an empty position between 0 and 8. * * If the corresponding cell in the board array contains a space, place an 'O' * in that cell. * * Otherwise, the user has specified a bad position. Tell the user they have * entered an "invalid move" and call the displayGameBoard method to redisplay * the board. * * Use a loop to keep asking them to enter a position until they enter the * number of an empty cell. Also display the "invalid move" message if the user * enters a number less than 0 or greater than 8. */ // <--- YOUR CODE HERE ---> } // ******************************************************* private static void displayGameBoard() { /* * Display the board based on the contents of the appropriate cells in the board * array. * * Since the board array contains chars (' ' or 'X' or 'O'), you can concatenate * them with other chars and Strings to print them. * * A printed board should look something like it does in the project * instructions. */ // <--- YOUR CODE HERE ---> } // ******************************************************* private static boolean isDone() { /* * The game is over if one of the following situations occurs: (1) The computer * won (i.e., there are 3 X's in a row), (2) the human won (i.e., there are 3 * X's in a row), or (3) nobody won, but all nine cells contain an X or O. * * All that's needed for this method is an if-else structure that checks if the * various situations exist. If calling isComputerWin() or isHumanWin() or * isTie() returns true, display an appropriate message and return true for this * method. If all of these methods return false, the game is not over and you * should return false for this method. */ // <--- YOUR CODE HERE ---> return false; // this line is provided so the skeleton compiles -- // replace it with your own code } // ******************************************************* private static boolean isComputerWin() { /* Nothing more to do here... see the isPlayerWin method below. */ return isPlayerWin('X'); } // ******************************************************* private static boolean isHumanWin() { /* Nothing more to do here... see the isPlayerWin method below. */ return isPlayerWin('O'); } // ******************************************************* private static boolean isPlayerWin(char player) { /* * Checking to see if the computer has won consists of determining if there are * three 'X's in row. Similarly, checking to see if the human has won consists * of seeing if there are three 'O's in row. * * Rather than write two methods that are almost exactly the same, you will * write one method that looks for a character (passed in to this method as a * parameter called player) that occurs three times in a row. * * Remember, there are eight different ways to get three in a row. */ // <--- YOUR CODE HERE ---> return false; // this line is provided so the skeleton compiles -- // replace it with your own code } // ******************************************************* private static boolean isTie() { /* * The game is over (and a tie) if all of the cells have an 'X' or an 'O' but no * player has three in a row. Check to see if the game is a tie. */ // <--- YOUR CODE HERE ---> return false; // this line is provided so the skeleton compiles -- // replace it with your own code } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
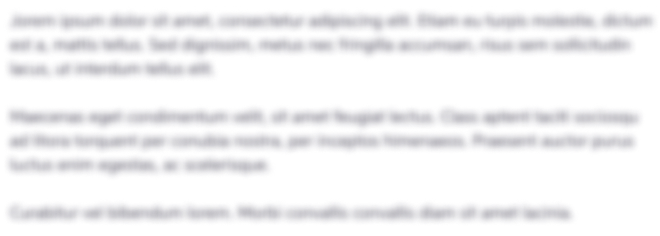
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started