Question
The table is produced using a while loop that iterates through interest rates starting at 5% and incrementing 1/8 at a time to 8%. Each
The table is produced using a while loop that iterates through interest rates starting at 5% and incrementing 1/8 at a time to 8%. Each iteration of the while loop can compute a new monthly and total payment based on the incrementing annual interest rate.
Listing 2.8 ComputeLoan.py from Chapter 2 is a program that asks the user an annual interest rate, number of years and loan amount, and produces a monthly payment and total payment.
# Listing 2.8 ComputeLoan.py # Enter yearly interest rate annualInterestRate = float(input("Enter annual interest rate, e.g., 8.25: ")) monthlyInterestRate = annualInterestRate / 1200 # Enter number of years numberOfYears = int(input("Enter number of years as an integer, e.g., 5: ")) # Enter loan amount loanAmount = float(input("Enter loan amount, e.g., 120000.95: ")) # Calculate payment monthlyPayment = loanAmount * monthlyInterestRate / (1 - 1 / (1 + monthlyInterestRate) ** (numberOfYears * 12)) totalPayment = monthlyPayment * numberOfYears * 12 # Display results print("The monthly payment is", int(monthlyPayment * 100) / 100) print("The total payment is", int(totalPayment * 100) /100)
This code can be used inside the while loop producing the line by line table of payments by interest rate.
To create the graphical user interface, we can start by getting the WidgetsDemo.py program running:
from tkinter import * # Import tkinter class WidgetsDemo: def __init__(self): window = Tk() # Create a window window.title("Widgets Demo") # Set a title # Add a button, a check button, and a radio button to frame1 frame1 = Frame(window) # Create and add a frame to window frame1.pack() self.v1 = IntVar() cbtBold = Checkbutton(frame1, text = "Bold", variable = self.v1, command = self.processCheckbutton) self.v2 = IntVar() rbRed = Radiobutton(frame1, text = "Red", bg = "red", variable = self.v2, value = 1, command = self.processRadiobutton) rbYellow = Radiobutton(frame1, text = "Yellow", bg = "yellow", variable = self.v2, value = 2, command = self.processRadiobutton) cbtBold.grid(row = 1, column = 1) rbRed.grid(row = 1, column = 2) rbYellow.grid(row = 1, column = 3) # Add a label, an entry, a button and a message to frame2 frame2 = Frame(window) # Create and add a frame to window frame2.pack() label = Label(frame2, text = "Enter your name: ") self.name = StringVar() entryName = Entry(frame2, textvariable = self.name) btGetName = Button(frame2, text = "Get Name", command = self.processButton) message = Message(frame2, text = "It is a widgets demo") label.grid(row = 1, column = 1) entryName.grid(row = 1, column = 2) btGetName.grid(row = 1, column = 3) message.grid(row = 1, column = 4) # Add a text text = Text(window) # Create a text add to the window text.pack() text.insert(END, "Tip The best way to learn Tkinter is to read ") text.insert(END, "these carefully designed examples and use them ") text.insert(END, "to create your applications.") window.mainloop() # Create an event loop def processCheckbutton(self): cout = "check button is " + ("checked " if self.v1.get() == 1 else "unchecked") print(cout) def processRadiobutton(self): print(("Red" if self.v2.get() == 1 else "Yellow") + " is selected " ) def processButton(self): print("Your name is " + self.name.get()) WidgetsDemo() # Create GUI
The execution window looks as follows:
Modify the program so clicking the Get Name button also adds the Console output to the text box:
You basically accomplish this by adding the following line at the end of the processButton(self) method:
self.text.insert(END," Your name is " + self.name.get())
For this to work, you need to add the self. prefix to all the other references to the text object, starting with:
self.text = Text(window,height=30) # Create a text window self.text.pack()
Adding the self. prefix on the amount and years IntVar() objects will allow their access inside the event handlers.
Now, once that works, you can remove the Bold, Red, Yellow row. Modify the Enter your name row with Entry boxes for inputting the loan amount and number of years.
Inside the processButton(self) event handler, the loanAmount and numberOfYears is used to calculate a monthly and total payment for the interest rates defined by the while loop:
loanAmount = float(self.amount.get()) numberOfYears = self.years.get() annualInterestRate = 5.0 while annualInterestRateYour program should appear similar to the following image:
Add your name as a comment in the program and attach it below.
Python Please
Step by Step Solution
There are 3 Steps involved in it
Step: 1
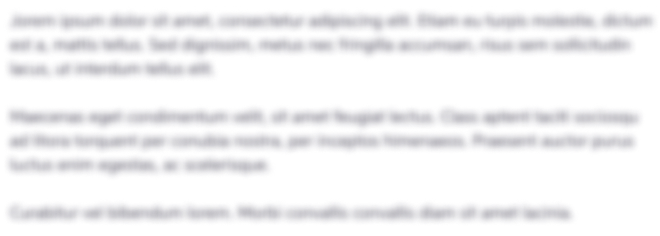
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started