Question
The ten digits or numbers that we learn early in life influence our daily life in far more ways we could ever imagine. These digits
The ten digits or numbers that we learn early in life influence our daily life in far more ways we could ever imagine.
These digits and the infinite array of other numbers they create represent age, height, license plate number, PIN numbers, bank account numbers, sequence/series of numbers, factors, squares, Fibonacci numbers, perfect numbers, and the list goes on. Over time, many of the infinite arrays, or patterns, of numbers derivable from the basic ten digits have been classified as a variety of number types.
Below are some basic definitions of terms. We will create a class (NumberProcessor) with static methods, which can be called to compute the result of some of the definitions; these methods are described below.
Number Methods:
1) public static boolean isComposite(int input) A composite number is defined to be the one that is positive integer and has at least one divisor other than 1 and itself. Negative numbers are not composite by definition. A composite number can be formed by multiplying two smaller positive integers. Write a method named isComposite that returns true if its integer argument is composite, otherwise it returns false.
input | return |
---|---|
0 | false |
1 | false |
2 | false |
3 | false |
4 | true (because 2 is a divisor) |
5 | false |
6 | true (3 is a divisor) |
7 | false |
8 | true (2,4 are divisors) |
9 | true (3 is a divisor) |
10 | true (2,5 are divisors) |
11 | false |
12 | true (2,3,4,6 are divisors) |
13 | false |
-14 | false |
15 | true (3, 5 are are divisors) |
2) public static boolean isFriendly(int num1, int num2)
Friendly numbers are two non-zero integers, each of which is the sum of the other's aliquot divisors. Aliquot divisors are all positive divisors of the number other than itself. For example, 1184 and 1210 are friendly numbers because all the aliquot divisors of 1184, i.e., 592, 296, 148, 74, 37,32, 16,8, 4, 2, 1 add up to 1210 and all the aliquot divisors of 1210, i.e., 605, 242, 121,110,55,22,11,10,5,2, 1 add up to 1184.
3) public static boolean isEquivalent(int num1, int num2)
Equivalent integers are non-zero integers where the sum of the aliquot divisors (refer to the previous question) are equal. For instance, 159 and 703 are equivalent numbers, since f(159) = 1, 3, 53, and 159 where 1 + 3 + 53 = 57 f(703) = 1, 19, 37, and 703 where 1 + 19 + 37 = 57
4) public static int reverseNum(int num)
Write a method named reverseNum that accepts an integer and returns the reverse of the integer. For example, if num=456 the method returns 654 (six hundred fifty four). Similarly if num=100000 , it returns 1 (one) and for -57 it returns -75 (negative seventy five).
5) public static boolean isReverseEquivalent(int num1, int num2)
Two integers are said to be ReverseEquivalent if the reverse of one of the numbers is equal to the other number. Write a method named isReverseEquivalent that accepts two integers and returns true if the two ints are ReverseEquivalent, otherwise it returns false. (Hint: you can use the reverseNum method defined for the previous question).
input numbers | return |
---|---|
255, 552 | true |
552, 255 | true |
333, 333 | true |
-637, -736 | true |
444, 325 | false |
Array Methods:
1) public static boolean isListEven(int[] array)
An array is said to be ListEven if it is non-empty, its elements are in ascending order, and each element appears two or more times. Write a method named isListEven that accepts an array of integers and returns true if the array is ListEven, otherwise it returns false.
input array | return |
---|---|
{0, 1, 2, 3} | fasle (elements appear only once) |
{1, 1, 2, 2, 3, 3} | true |
{3, 3, 2, 2} | false(elements are not in ascending order) |
{1} | false |
{ } | false |
2) public static double evaluate(double x, int[ ] coefficient)
Write a method named evaluate that returns the solution of the polynomial anxn + an-1xn-1 + ... + a1x1 + a0
x input | array input | Polynomial representation | evaluate returns |
---|---|---|---|
1.0 | {0,1, 2, 3,4} | 4x4+3x3+2x2+x+0 | 10.0 |
3.0 | {3, 2, 1} | x2 + 2x + 3 | 18.0 |
2.0 | {3, -2, -1} | -x2 - 2x + 3 | -5.0 |
-3.0 | {3, 2, 1} | x2 + 2x + 3 | 6.0 |
2.0 | {3, 2} | 2x + 3 | 7.0 |
2.0 | {4, 0, 9} | 9x2 + 4 | 40.0 |
2.0 | {10} | 10 | 10.0 |
10.0 | {0, 1} | x | 10.0 |
3) public static boolean isIDA(int array[])
An array is called isIDA if it has the following properties
- The value of the first element equals the sum of the next two elements, which equals to the next three elements, which equals to the sum of the next four elements, etc.
- It has a size of x*(x+1)/2 for some positive integer x .
Write a method named isIDA() that returns true if the array is isIDA false otherwise
input array | output |
---|---|
{6, 2, 4, 2, 2, 2, 1, 5, 0, 0} | true (6=2+4=2+2+2=1+5+0+0) |
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, -2, -1} | true |
{2, 1, 2, 3, 5, 6} | false (2 !=1+2 != 3+5+6) |
{} | false |
{0,0} | false (does not meet the second criterion) |
{1,0,1,0,1,0,1,0} | false |
4) public static boolean isDual(int array[])
- An array is called Dual if it can be divided into two non-empty sub arrays, where the sum of elements of the first sub array equals the sum of elements of the second sub array. The order of elements in the sub arrays should be the same as the original array. Define a method isDual that returns true if the array is dual, false otherwise. Use the following signature for your method.
input array output {6, 2, 4, 2, 2, 2, 1, 5, 0, 0} true ( {6,2,4} and {2,2,2,1,5,0,0} ) {0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, -2, -1} true ( {0,0,0,0,0,0,0,0,0,0} and {1,1,1,-2,-1}) {2, 1, 2, 3, 5, 6} false {} false {0,0} true ({0} and {0}) {1,0,1,0,1,0,0,0} false
5) public static int [ ] representASCII(char [ ] input)
- Write a java method named representASCII that accepts an array of characters and returns an array of integers that contains the decimal representation (ASCII) of each character in the input array.
input array output array { '6', 'k', 'R', '}' } {54, 107, 82, 125} { '0', '.', '7', '^', '*' } {48, 46, 55, 94, 42} {} {}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
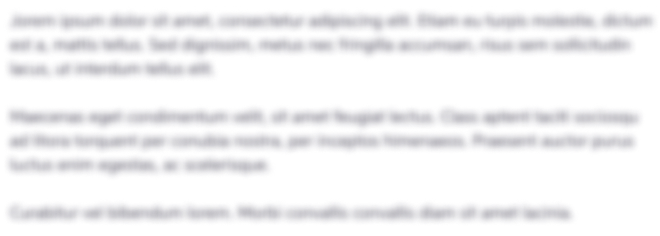
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started