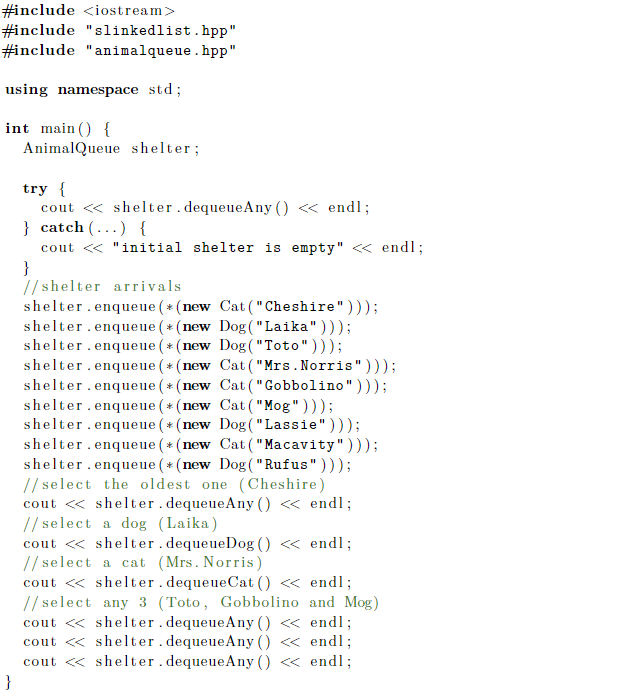
^^The test driver
This was the slinkedlist.hpp
#ifndef SLINKEDLIST_HPP #define SLINKEDLIST_HPP #include template class SLinkedList; template std::ostream& operator&); template class SLinkedList { private: class SNode { public: T elem; SNode* next; }; private: SNode* head; public: SLinkedList(); ~SLinkedList(); bool empty() const; int len() const; const T& front() const; T greatestValue() const; void addFront(const T& e); void addBack(const T& e); void addSorted(const T& e); T removeFront(); bool remove(T v); void reverse(); void clear(); friend std::ostream& operator(std::ostream& out, const SLinkedList& sl); }; template SLinkedList::SLinkedList() : head(NULL) {} template SLinkedList::~SLinkedList() { clear(); } template bool SLinkedList::empty() const { return head == NULL; } template int SLinkedList::len() const {
SLinkedList::SNode* p = head; int i = 0; while(p != NULL) { i++; p = p->next; } return i; } template const T& SLinkedList::front() const { return head->elem; } template T SLinkedList::greatestValue() const { SLinkedList::SNode* p = head; T v = head->elem; while(p != NULL) { if(p->elem > v) v = p->elem; p = p->next; } return v; } template void SLinkedList::addFront(const T& e) { SLinkedList::SNode* v = new SNode; v->elem = e; v->next = head; head = v; } template void SLinkedList::addBack(const T& e) { SLinkedList::SNode* v = new SNode; v->elem = e; v->next = NULL; if(empty()) head = v; else { SNode* p = head; while(p->next != NULL) p = p->next; p->next = v; } } //insertion sort template void SLinkedList::addSorted(const T& e) { SLinkedList::SNode* v = new SNode; v->elem = e;
v->next = NULL; if(empty()) head = v; else if(v->elem elem) { v->next = head; head = v; } else { SLinkedList::SNode* p1 = head; SLinkedList::SNode* p2 = p1; while(p1 != NULL && v->elem > p1->elem) { p2 = p1; p1 = p1->next; } p2->next = v; v->next = p1; } } template T SLinkedList::removeFront() { SNode* p = head; T v = head->elem; head = p->next; delete p; return v; } template bool SLinkedList::remove(T v) { SLinkedList::SNode* p1 = head; SLinkedList::SNode* p2 = p1; if(head->elem == v) return removeFront(); while(p1 != NULL) { if(p1->elem == v) { p2->next = p1->next; delete p1; return true; } p2 = p1; p1 = p1->next; } return false; } template void SLinkedList::reverse() { SLinkedList::SNode* p1 = head; SLinkedList::SNode* p2 = NULL; while(head != NULL) { head = p1->next; p1->next = p2; p2 = p1; p1 = head; }
head = p2; } template void SLinkedList::clear() { while(!empty()) removeFront(); } //global template std::ostream& operator& sl) { typename SLinkedList::SNode* p = sl.head; out "; while(p != NULL) { out elem "; p = p->next; } out An animal shelter, which holds only dogs and cats, operates on a strictly FIFO (first-in, first-out) basis. People must adopt either the oldest (based on arrival time) of all animals at the shelter, or they can select whether they would prefer a dog or a cat (and will receive the oldest animal of that type). i.e. They cannot select which specific animal they would like. Implement an Animalqueue class capable of maintaining this system structure. In particular, your Animalqueue class must implement the following member functions: enqueue, dequeueAny, dequeueDog, and dequeueCat. You must use the singly linked list (SLinkedList) implementation seen in class without modifying it, so you need to think carefully how to handle the order of arrival and how to use it to determine which animal is selected (Hint: use a counter instead of an actual timestamp). You can use the following code as a test driver for your implementation (see below). Please note that you must provide a simple Cat and Dog class definition to properly use the test driver, and that the Animalqueue is a simple wrapper around the Slinkedlist class. Finally, you are free to choose to implement only one queue or use two separate queues