Question
There are two sides which need to be coded, the Auction server and the seller/buyer client server In this project, you will implement socket-based programs
There are two sides which need to be coded, the Auction server and the seller/buyer client server
In this project, you will implement socket-based programs that will simulate the sealed-bidauctions. There will be 2 types of processes running the simulation: an"Auctioneer"serverforhostingtheauctions,anda"Seller/Buyer"clientboth for submitting auctionrequests to the auctioneer and for bidding for items in the auction. A client can by either aSeller,oraBuyer,butnotboth.
An auction starts with a Seller submitting an auction request to the Auctioneer, which specifiesthe type of auction (first-price or second-price), the lowest price acceptable to the Seller, theintended number of bids, and the good to be sold. The Auctioneer opens up the auction afterreceiving the request (if no other auction is on-going), and starts accepting bids from Buyers. ABuyer submits a bid to the Auctioneer by first connecting to the Auctioneer server, and thensubmitting its bid. Once a bid is received from every connected, the Auctioneer processes allbids,findsthehighest bid,andnotifies theSellerandtheBuyersstatusoftheauction.
"Auctioneer" Server
The server is continuously listening on a welcoming TCP port for clients to connect to it. Whenthe server detects that a new client is trying to connect to it, it creates a new TCP socket to talktothatspecificclient anddoesthefollowing:
- The server runs in two states: "Waiting for Seller" (status 0), and "Waiting for Buyer"(status1).Theserver startsinstatus0.
- If the server is running in status 0, and there has not been any client connected to it, thenext connected client assumes the Seller role. The server will inform the client to submitan auction request, and wait for it to submit its auction request. For any other incomingconnection before the server receives the auction request, the server replies "Serverbusy!"tothenewclientandclosestheconnection.
- To realize this, you make a new thread to handle the input from the Sellerclient, while the original thread is still accepting new in-coming clients, sendingoutabusymessageafter eachconnected,andclosingtheconnection.
- Displayamessage whenthenewthreadisspawned.
- Upon receiving a message from the Seller, the server decodes the message into fourfields:(1forfirst-price,and2forsecond-price), (positive integer), (positive integer < 10), and (chararrayofsize<=255).
- If the decoding fails, the server informs the Seller "Invalid auction request!", andwaitsfortheSeller tosendanotherrequest.
- Ifthedecoding succeeds,theserverinformstheSeller"Auctionrequestreceived:[message_received]".Theserverthenchangesstatus1.
- In status 1, the server will accept in-coming connections from up to clientsasBuyers.Whenaclientisconnected:
- If the current number of Buyers is smaller than , the serverinforms the client that the server is currently waiting for other Buyers, and waitsforotherconnections.
- If the current number of Buyers is equal to , the server tellseveryconnectedclient"Biddingstart!",andstartsthebiddingprocess.
- If the current number of Buyers exceeds , the server tells thenewclient"Biddingon-going!",andclosestheconnection.
- The server starts the bidding process by creating a new thread for handling the biddinginputs from Buyers, while the original thread is dedicated to rejecting newly comingclients as in Step 4 during this process. Display a message when the new thread isspawned.
- During bidding, the server will continuously receive data from any Buyer who has notsubmittedabidyet.Uponreceiving amessagefromaBuyer:
- Ifthemessage containsasingle positiveintegerasthebid,theserverrecordsthe bid with the sending Buyer, tells the Buyer "Bid received. Please wait...", anddoesnotacceptanyfurthermessages fromthisBuyer.
- If the message contains any other data, the server informs the Buyer "Invalid bid.Please submit a positive integer!", and attempts to receive another round ofmessages fromthisBuyer.
- Upon receiving all bids, the server will run through all Buyers and their bids, and identifytheBuyerwiththehighestbidb.Itthendecides theresultofthisaction asfollows:
- If the highest bid is no less than , the auction succeeds. Theserverdoesthefollowing:
- If the = 1 (first-price), the server tells the Seller that theitem is sold forbdollars, then tells the highest-bid Buyer that it won in thisauction and now has a payment due ofbdollars, and finally tells each oftheotherBuyersthatunfortunately,itdid not win in this auction. Theserverthencloses the connection to all clients. (We omit the actualpayment and good delivery in this project, and defer that to our nextproject.)
- If the = 2 (second-price), the server finds the secondhighest bidb1 among all bids including theSeller's . TheserverthentellstheSellerthattheitemissoldforb1dollars,thentellsthe highest-bid Buyer that it won in this auction and now has a paymentdueofb1dollars,andfinallytells eachoftheotherBuyersthatunfortunately it did not win in this auction. The server then closes theconnectiontoallclients.
- If the highest bid is no less than , the auction succeeds. Theserverdoesthefollowing:
- If the highest bid is less than , the server tells the Seller thatunfortunately its item was not sold in the auction, and tells all other clients thatunfortunately,theydidnotwinintheauction. Theserverthenclosesallconnections.
- After the auction is done and everyone is informed, the server cleans all the informationabout this auction including the list of Buyers, and then reset its status to0, to wait foranyotherSeller'sconnectionandauctionrequest.
"Seller/Buyer" Client
Whentheclientstarts,ittriestocontacttheserveronaspecified port.
SellerMode
- Iftheclientreceivesmessage fromtheserver tosubmitanauctionrequest afterconnection,itholdstheroleofaSeller.
- Toproceed,theclientsendsanauction request,intheformof"
",totheserver,andwaitsforserverreply.
- Iftheserver indicatesaninvalid request,theclient mustresendavalidrequest.
- Iftheserverindicates "Auction request received: [message_sent]", the client waits untiltheauctionfinishes toobtaintheauctionresult.
BuyerMode
- Iftheclientreceivesa messagefromtheservertowaitforanotherBuyerorstartbidding,itholdstheroleofaBuyer.
- Whenthebidding starts,theBuyersendsitsbid(apositiveinteger value)totheserver.
- Iftheserverindicatesaninvalidbid,theclient mustresendavalidbid.
Iftheserverindicates"Bidreceived",theclientwaits forthefinalresultoftheauction.
When starting the client, specify the IP address of theAuctionServer and the portnumberwheretheserverisrunning.Intheexamplebelow,theserverisatIPaddress
127.0.0.1runningonTCPport12345.Ifsuccessfullyconnected,amessageisdisplayed with relevant information, as in Steps 2 (client) and 3 (server), respectively.Step 4 is displayed when the client receives the Seller role prompt from the server.When a request is entered from the Seller side (Step 5), if it is invalid, the server shouldreturn an invalid prompt; if it is valid, the server should send a feedback to the Seller(Step 6) and set itself to wait for Buyers. The Seller displays the a message indicatingtheauctionhasstarted(Step 7). Here the request "2 10 3 WolfPackSword" is used asanexample(whichmeans"useauctiontype2,startingat$10minimum,allow3bids,forthe item name 'WolfPackSword'to be sold.")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
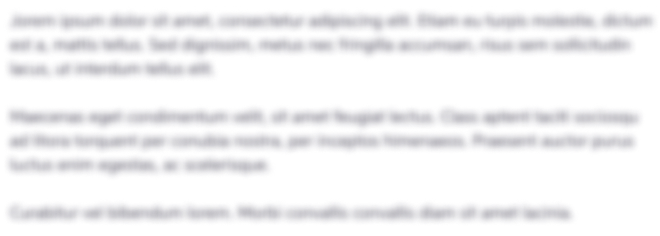
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started