Question
There is no method for removing a ConcertTicket from the wallet! Your job is to write that method. The method should be called remove. It
There is no method for removing a ConcertTicket from the wallet! Your job is to write that method. The method should be called remove. It should take no parameters, and it should return the most recently added ConcertTicket.
******************************************************************************************************************************************************************
package ConcertTicket;
import java.util.Arrays;
/** * This class is a wallet which can store any number of concert tickets. * * @author manuel a perez-quinones * @version Fall 2019 */ public class Wallet {
/** * Array of ConcertTicket objects */ protected ConcertTicket[] tickets = new ConcertTicket[10]; private int size = 0;
/** * Add a concert ticket into the array * * @param ct a concert ticket being added to the wallet */ public void add(ConcertTicket ct) { if (this.size == this.tickets.length) { this.resize(); } this.tickets[this.size] = ct; this.size++; }
/** * Resizes the wallet so that you can fit more tickets. * */ public void resize() { this.tickets = Arrays.copyOf(this.tickets, 2 * this.tickets.length); }
/** * Converts the wallet into a string representation for easy reading. * * @return a string representation of the wallet */ @Override public String toString() { return Arrays.toString(Arrays.copyOf(this.tickets, size)); }
/** * Returns how many tickets are in the wallet. * * @return the size of the wallet */ public int getSize() { return this.size; } /** * Returns the size of the wallet. * * @return the size of the wallet */ public int getLength() { return this.tickets.length; } /** * Removes the most recently added concert ticket from the wallet * * @return the concert ticket removed from the wallet */ public ConcertTicket remove() {
// Create a ConcertTicket reference variable (DO NOT create a // new ConcertTicket JUST create the reference variable)
if (this.size > 0) { // TODO Use the size variable //(which always points at the next empty // slot) to get the last added ConcertTicket from the array: // TODO Set that array slot to null: // TODO Decrement the size variable: } // Return the ConcertTicket: return null; }
}
*********************************************************************************************************************************************************
package ConcertTicket;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * This it the class ConcertTicket that stores the name of a concert, the price, * and the date. * * @author clatulip, mapq * @version Fall 2019 */ public class ConcertTicket implements Comparable {
private String name; private String date; private double price;
/** * Constructor with default value for all the fields. * * @param name of band * @param date of the concert * @param price of the ticket */ public ConcertTicket(String name, String date, double price) { this.name = name; this.date = date; this.price = price; }
/** * Default constructor for this class. */ public ConcertTicket() { name = ""; date = new String(); price = 0.0; }
/** * Getter returning the name of the band performing concert. * @return name of the band */ public String getName() { return name; }
/** * Setter storing the name of the band. * * @param name to be stored for the band */ public void setName(String name) { this.name = name; }
/** * Getter returning the date of the concert. * * @return the date of the concert */ public String getString() { return date; }
/** * Setter storing the date of the concert. * * @param date the date of the concert */ public void setString(String date) { this.date = date; }
/** * Getter returning the price for the ticket to the concert. * * @return the price */ public double getPrice() { return price; }
/** * Setter to set the price of the ticket of the concert. * * @param price the price of the ticket */ public void setPrice(double price) { this.price = price; }
/** * Method from Comparable that compares two concert ticket objects by * looking at ticket price. * * @param o * @return -1,0,1 */ @Override public int compareTo(Object o) { ConcertTicket ct = (ConcertTicket) o; int result = 0;
// compare by price if (this.price ct.price) { result = 1; } return result; }
/** * Returns a string version of this object. * * @return string representation of this object */ @Override public String toString() { return "ConcertTicket{" + "name=" + name + ", date=" + date + ", price=" + price + '}'; }
} ************************************************************************************************************************************************************
Test Driven Development 1. Here are the files in your project for the Cencurtitictat paralgy - 2 Get fariliar with these files and read through the commentst 3. 4. Onre you are dane, make sure there are no syntax enrors. You can do so by opening the problens view - by didaing on this button in the battom left - Which opers this tab terts - 6. If all terts have passed, hit subritit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
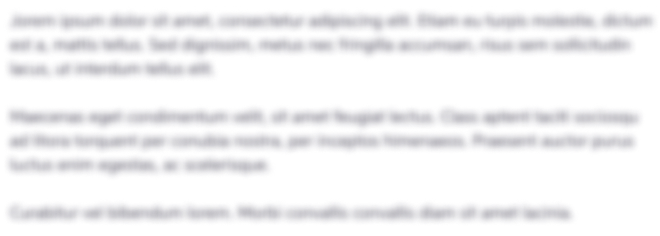
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started