Question
Third JAVA Assignment The Facebook Class Now well continue our project by writing a Facebook class that contains an ArrayList of Facebook user objects. The
Third JAVA Assignment The Facebook Class
Now well continue our project by writing a Facebook class that contains an ArrayList of
Facebook user objects. The Facebook class should have methods to list all of the users (i.e. print out their usernames), add a user, delete a user, and get the password hint for a user. You will also need to create a driver program. The driver should create an instance of the Facebook class and display a menu containing five options:
Menu
- List Users,
- Add a User,
- Delete a User,
- Get Password Hint
- Quit
Then it should read in the users choice and call the appropriate method on the Facebook object. This should continue until the user chooses to quit. You dont need to worry about adding and removing friends for this assignment well do that later in the project. Heres the catch: we want the contents of our Facebook object to persist between program executions. To do this, serialize your Facebook object before the program terminates. When the program starts up again, de-serialize the Facebook object rather than creating a new one.
Here are some details:
When adding a new FacebookUser, prompt the user for the username and check to see that if the users ArrayList already contains a FacebookUser object with that username. If it does, display an error message. If the username is unique, prompt for a password and password hint and create a new FacebookUser object with those values. Add this new user to the users ArrayList. When deleting a FacebookUser, prompt for the username and check to see that the users ArrayList contains a FacebookUser object with that username. If it doesnt display an error message. If it does, prompt for the password and check that the FacebookUser object with this username has the same password as the one that was entered. If it doesnt, display an error message. If the passwords match, delete this FacebookUser object from the users ArrayList When retrieving the password hint for a FacebookUser, ask for the username and display an error message if there is no user with that username. Obviously, if the user does exist you should display the password hint without requiring the users password.
You will be graded according to the following rubric (each item is worth one point):
- The Facebook class has the appropriate fields and methods
- The Facebook class can list the FacebookUser objects (i.e. their usernames)
- It is possible to add a user at runtime
- It is possible to delete a user at runtime
- It is possible to get the password hint for a user at runtime
- The driver program creates a Facebook object that is serialized before the program ends
- The driver program de-serializes the Facebook object when it starts executing, if a serialized version exists. If a serialized version of the Facebook object does not exist, the driver program creates a new Facebook object.
- The program compiles
- The program runs
- The program is clearly written and follows standard coding conventions
- Note: If your program does not compile, you will receive a score of 0 on the entire assignment
- Note: If you program compiles but does not run, you will receive a score of 0 on the entire assignment
- Note: If your Eclipse project is not exported and uploaded to the eLearn drop box correctly, you will receive a score of 0 on the entire assignment
- Note: If you do not submit code that solves the problem for this particular assignment, you will not receive any points for the programs compiling, the programs running, or following standard coding conventions.
Here is my code
DriverProgram.java
import java.util.ArrayList; import java.util.Collections;
public class DriverProgram {
public static void main(String[] args) { FacebookUser user1 = new FacebookUser("Walter White", "pa55"); FacebookUser user2 = new FacebookUser("jesse Pinkman", "password"); FacebookUser user3 = new FacebookUser("Daryl Dixon", "pas5"); FacebookUser user4 = new FacebookUser("rick Grimes", "12345"); System.out.println(" "); // create arrayList of FacebookUser then sort alphabetically ignoring case ArrayList
System.out.println("Users: "); for (FacebookUser faceBookUser : users) { System.out.println("\t" + faceBookUser); }
System.out.println(" Sorting Users... ");
Collections.sort(users);
System.out.println("Users: "); for (FacebookUser faceBookUser : users) { System.out.println("\t" + faceBookUser); }
System.out.println(" "); // test setPasswordHint and getPasswordHelp methods user1.setPasswordHint("Thou shalt not pass"); user1.getPasswordHelp(); System.out.println(" "); user1.setPasswordHint("pwerd"); user1.getPasswordHelp(); //test friend method System.out.print(" "); System.out.println("***Test friend method***"); user1.friend(user4); user1.friend(user3); user1.friend(user3); user1.friend(user2); //test defriend method System.out.print(" "); System.out.println("***Test defriend method***"); user1.defriend(user2); user1.defriend(user2);
//test getFriend method System.out.print(" "); System.out.println("***Test getFriend method***"); user1.getFriends();
}
}
FacebookUser.java
import java.util.ArrayList;
public class FacebookUser extends UserAccount implements Comparable
public FacebookUser(String username, String password) { super(username, password); friends = new ArrayList
public void setPasswordHint(String hint) { this.passwordHint = hint; }
public void friend(FacebookUser newFriend) { if (friends.contains(newFriend)) { System.out.println("That friend has already been added. "); } else { friends.add(newFriend); } }
public void defriend(FacebookUser formerFriend) { if (!friends.contains(formerFriend)) { System.out.println("That friend has not been added. "); } else { friends.remove(formerFriend); } }
public ArrayList
return friendsCopy; }
@Override public int compareTo(FacebookUser o) { if (this.username.compareToIgnoreCase(o.username) != 0) { return this.username.compareToIgnoreCase(o.username); }
return 0; }
@Override public void getPasswordHelp() { setPasswordHint(passwordHint); System.out.println("Password Hint: " + passwordHint);
} }
UserAccount.java
public abstract class UserAccount {
protected String username; protected String password; protected boolean active; // indicates whether or not the account is // currently active
public UserAccount(String username, String password) { this.username = username; this.password = password; active = true; }
public boolean checkPassword(String password) { boolean passwordMatch; if (password.equals(this.password)) { passwordMatch = true; } else { passwordMatch = false; } return passwordMatch; }
public void deactivateAccount() { active = false; }
@Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + ((username == null) ? 0 : username.hashCode()); return result; }
@Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; UserAccount other = (UserAccount) obj; if (username == null) { if (other.username != null) return false; } else if (!username.equals(other.username)) return false; return true; }
@Override public String toString() { return "Username: " + username + " "; }
public abstract void getPasswordHelp();
} // end UserAccount
Step by Step Solution
There are 3 Steps involved in it
Step: 1
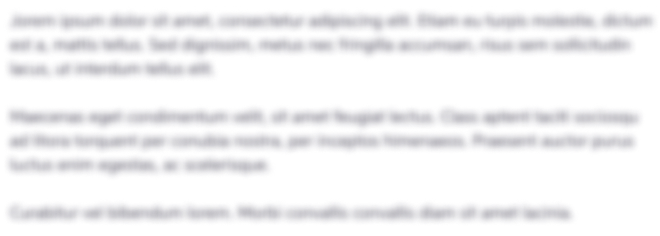
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started