Answered step by step
Verified Expert Solution
Question
1 Approved Answer
This assignment involves coding the game 2048 using 2-D arrays. I really just need help with the second and third methods. I need help with
This assignment involves coding the game 2048 using 2-D arrays. I really just need help with the second and third methods.



I need help with the addRandomTile and swipeLeft here is the template code for Board.Java:
package game;
import java.util.ArrayList;
/**
* 2048 Board
* Methods to complete:
* updateOpenSpaces(), addRandomTile(), swipeLeft(), mergeLeft(),
* transpose(), flipRows(), makeMove(char letter)
*
**/
public class Board {
private int[][] gameBoard; // the game board array
private ArrayList openSpaces; // the ArrayList of open spots: board cells without numbers.
/**
* Zero-argument Constructor: initializes a 4x4 game board.
**/
public Board() {
gameBoard = new int[4][4];
openSpaces = new ArrayList();
}
/**
* One-argument Constructor: initializes a game board based on a given array.
*
* @param board the board array with values to be passed through
**/
public Board ( int[][] board ) {
gameBoard = new int[board.length][board[0].length];
for ( int r = 0; r
for ( int c = 0; c
gameBoard[r][c] = board[r][c];
}
}
openSpaces = new ArrayList();
}
/**
* 1. Initializes the instance variable openSpaces (open board spaces) with an empty array.
* 2. Adds open spots to openSpaces (the ArrayList of open BoardSpots).
*
* Note: A spot (i, j) is open when gameBoard[i][j] = 0.
*
* Assume that gameBoard has been initialized.
**/
public void updateOpenSpaces() {
// WRITE YOUR CODE HERE
}
}
/**
* Adds a random tile to an open spot with a 90% chance of a 2 value and a 10% chance of a 4 value.
* Requires separate uses of StdRandom.uniform() to find a random open space and determine probability of a 4 or 2 tile.
*
* 1. Select a tile t by picking a random open space from openSpaces
* 2. Pick a value v by picking a double from 0 to 1 (not inclusive of 1);
* 3. Update the tile t on gameBoard with the value v
*
* Note: On the driver updateOpenStapes() is called before this method to ensure that openSpaces is up to date.
**/
public void addRandomTile() {
// WRITE YOUR CODE HERE
}
/**
* Swipes the entire board left, shifting all nonzero tiles as far left as possible.
* Maintains the same number and order of tiles.
* After swiping left, no zero tiles should be in between nonzero tiles.
* (ex: 0 4 0 4 becomes 4 4 0 0).
**/
public void swipeLeft() {
// WRITE YOUR CODE HERE
}
/**
* Find and merge all identical left pairs in the board. Ex: "2 2 2 2" will become "2 0 2 0".
* The leftmost value takes on double its own value, and the rightmost empties and becomes 0.
**/
public void mergeLeft() {
// WRITE YOUR CODE HERE
}
/**
* Rotates 90 degrees clockwise by taking the transpose of the board and then reversing rows.
* (complete transpose and flipRows).
* Provided method. Do not edit.
**/
public void rotateBoard() {
transpose();
flipRows();
}
/**
* Updates the instance variable gameBoard to be its transpose.
* Transposing flips the board along its main diagonal (top left to bottom right).
*
* To transpose the gameBoard interchange rows and columns.
* Col 1 becomes Row 1, Col 2 becomes Row 2, etc.
*
**/
public void transpose() {
// WRITE YOUR CODE HERE
}
/**
* Updates the instance variable gameBoard to reverse its rows.
*
* Reverses all rows. Columns 1, 2, 3, and 4 become 4, 3, 2, and 1.
*
**/
public void flipRows() {
// WRITE YOUR CODE HERE
}
/**
* Calls previous methods to make right, left, up and down moves.
* Swipe, merge neighbors, and swipe. Rotate to achieve this goal as needed.
*
* @param letter the first letter of the action to take, either 'L' for left, 'U' for up, 'R' for right, or 'D' for down
* NOTE: if "letter" is not one of the above characters, do nothing.
**/
public void makeMove(char letter) {
// WRITE YOUR CODE HERE
}
/**
* Returns true when the game is lost and no empty spaces are available. Ignored
* when testing methods in isolation.
*
* @return the status of the game -- lost or not lost
**/
public boolean isGameLost() {
return openSpaces.size() == 0;
}
/**
* Shows a final score when the game is lost. Do not edit.
**/
public int showScore() {
int score = 0;
for ( int r = 0; r
for ( int c = 0; c
score += gameBoard[r][c];
}
}
return score;
}
/**
* Prints the board as integer values in the text window. Do not edit.
**/
public void print() {
for ( int r = 0; r
for ( int c = 0; c
String g = Integer.toString(gameBoard[r][c]);
StdOut.print((g.equals("0")) ? "-" : g);
for ( int o = 0; o
StdOut.print(" ");
}
}
StdOut.println();
}
}
/**
* Prints the board as integer values in the text window, with open spaces denoted by "**"". Used by TextDriver.
**/
public void printOpenSpaces() {
for ( int r = 0; r
for ( int c = 0; c
Overview of files provided We provide two divers (text and graphic) to help you test the indididual mothods. We suggest that you start with the text driver but once you have a good understanding of the assignment then you may use the graphio diver. - Board: The Board elass contains al methods needed to construct a funefioning 2048 game. Edt the omply methads with you folution, but 00 NOT edit the provided ones or the methods signatures of any method. This is the file you submi. - BoardSpot: An object used to represent a location on the board. It houses a row and a column, along with getters and setters. Don' edit or submit to Autolab. - TexiDriver: A tool to tost your 2048 board interactively using only text-based boards. This drwer is equivalent to Arimatadoriver and tunctions in the same way - you are free to use this driver to test all of your methods. Feel tree to edt this class, as it is providec only to help you test. It is not submitted andor graded. - To use this driver. pick whether you want to test individual methods or play the full game this option requires all methads to be eompitadf by solecting the number that appears for each option. - To test individual methods, first type in the ful flee name for an input lie. Then. select a method to test by trping in the number that appeara for oach method in the ist. Additionally, for makeMove, moves are represented by using the WASD keys ike arrow keys (w is up, a is left, s is down, and d is righit. Type in the letter corresponding to the move you want to test. Playing the full game makes use of the WASD keys live arrow keys (W is up, A is toft. S is down, and D is right). Press "Q" to quat. - AnimatedDriver: A tool to test your 2048 board interactively through rendered images. This driver is equivalent to Textbriver and tunctions in the same way - you are free to use this driver to lest all of your methods. Foel tree to edit this class, as it is provided only to help you tent. It is not submitted and/or graded. 3. To use this diver, pick whether you want to test individual mothods or play the full game (ihis cotion requires all methods to be comploted) by selecting the number that appears for each option. - To test individual methods, solect an input fle name trom the list. Then, solect a mothod to best by typing in the number that appears for each method in the list. You wil then see the board stored in the input the. For all methods besides make Move, press ary key to teat yeur method. Press any key again to exit testing that method. "For makeMove, moves are represented by using the WaSD keys like arrow keys (w is up, a is lott, s is down, and dis night, Type in the letter corresponding to the move you want to test when you see the board stored in the input fle. Once you type that lother, the move you wart to that will be made. Press any key aftenward to exit testing that method. Playing the full game makes uso of the WASD keys Exe arrow keys (w is up, a is loth, s is down, and dis right). Press "qu" to quit. - Stdin and 5tdOut: libraries to handle input and output. Do not edit these classes. - StdAandom: Provides mothods for generating random numbers. Don't edit or submit to Autolab. - Colliage, Picture, StdDraw: Helper loraries to support AnimatedDriver. Don't edit or submit to Autolab. - Input Files: Preset boards youre able to use to test your 2048 board in the divers (intput1 in, inputr in. ). You can use all input tiles to test all methods. Each input file contains 4 ines, each with 4 space separatod numbers. Each number is ailher o (representa an amply opsco) or a powor of 2 (represents a normal the). Feel free to make your own input files, as they will not be submitted to Autolab. 2. addPandomTile Note: wadateopenspaces must be completed betore starting this method. This method adds a random tie into the board, following these fules: iss is to generate a random integer betweon a lower bound "a' up to (but not including) an upper bound b ", To randomly pick a board spot the lower bound is 0 and the upper bound is the number of itwems in the ArrayLis. - Next, assign a value to the tio. There is a 00 rs chance that a 2 tile will be insented and a 10% chance that a 4 tie sill be inserted. Use - To randomly pick a number between 0 and 1 the lower bound is 0 and the upper bound is 1 . - If this value is less than, but not equai to, 10 percent (0.1), the tle will have a 4 value. Otherwise, the tie wal have a 2 value. - Be sure to update the correct tile in the gameBoard aray (as detined by the Boandspot's row and column values) with the proper value. - Nole: On the diver updateOpenSpacese is calod before this method to ensure that openSpacos is up lo date. You do not need to remove the board spot from the openSpaces ArrayLiet! - Nole: On the driver, the seed is set so you will gen the same ourputs every time when testing indvidual methods. Autolab uses the sene seed as ne idiver Io lest this method. However, whon playing the tull game, the diver will not use seed values it will use random values to gonerase different bosids and tiles. Here is an example of testing this method using input1 in in both drivers. 3. swipeleft Move all tiles as far lett as possible while maintaining the same order and number of tiles. - There should be no empty spaces to the left of any tile. - Remember that 0 (gamesoard(rou) (co11 - 0) represents an empty space, - Watch this video to learn more about this method. Here is an example of testing this method using input1. in in both diversString g = Integer.toString(gameBoard[r][c]);
for ( BoardSpot bs : getOpenSpaces() ) {
if (r == bs.getRow() && c == bs.getCol()) {
g = "**";
}
}
StdOut.print((g.equals("0")) ? "-" : g);
for ( int o = 0; o
StdOut.print(" ");
}
}
StdOut.println();
}
}
/**
* Seed Constructor: Allows students to set seeds to debug random tile cases.
*
* @param seed the long seed value
**/
public Board(long seed) {
StdRandom.setSeed(seed);
gameBoard = new int[4][4];
}
/**
* Gets the open board spaces.
*
* @return the ArrayList of BoardSpots containing open spaces
**/
public ArrayList getOpenSpaces() {
return openSpaces;
}
/**
* Gets the board 2D array values.
*
* @return the 2D array game board
**/
public int[][] getBoard() {
return gameBoard;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
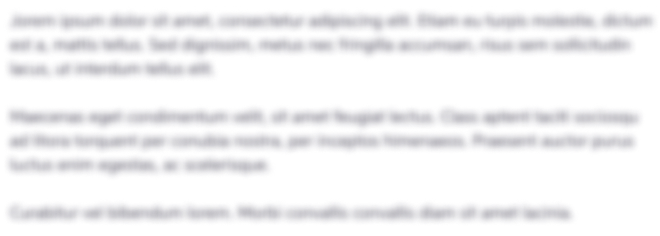
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started