Question
This assignment will give you experience working with classes, throwing exceptions, and JUnit testing. You will not have to write try catch blocks for this
This assignment will give you experience working with classes, throwing exceptions, and JUnit testing. You will not have to write try catch blocks for this assignment since you will only be throwing exceptions, not catching them. You just got a sweet gig working for a vending machine company called iVend. They specialize in designing high-tech vending machines. Youve been hired to program the logic for one of their machines. Your first job is to design a class that models the behavior of an iVend machine that dispenses snacks. An iVend machine works as follows. When a customer walks up to an iVend snack machine, he/she can either select an item or insert cents (selecting an item does not purchase the item, it simply informs the iVend machine which item the customer is interested in). This particular machine only sells three items: Snickers, Twix, and Reeses. (Snickers costs 100 cents, Twix costs 115 cents, and 1 Reeses costs 130 cents.) After the customer has inserted cents he/she can press a button to return the cents (if they decide not to purchase anything), or they can press a different button to purchase a selected item and have any unspent cents returned to them (given they inserted more cents than the cost of the purchased item). There are many ways in which a customer can misuse an iVend machine. For example, a customer can attempt to press the purchase button before selecting an item, or a customer can insert a number of cents that is not a multiple of 5 (only nickels, dimes, and quarters can be inserted). Because of this, youll need to throw exceptions when a user has misused the machine. This machine also has a feature that informs the owner how much money it has made in purchases. After you created the class for the iVend machine, your boss wants you to design some JUnit tests in order to catch any bugs that your class might have.
The details for the Vending Machine and VendingMachineTester classes are discussed below.
The Vending Machine Class Create a new class called VendingMachine in the Vending package. This class must correctly implement the VendingMachineInterface interface. Thus you should have public class Vending Machine implements VendingMachine Interface{ written at the top of the Vending Machine class. Please see slide 12 of our October 6th lecture to review what an interface is. You will notice that details for each public method are already written as a comment above each method heading in VendingMachineInterface. You simply need to write the code for these methods inside of VendingMachine. The comments inside of VendingMachineInterface.java also explain how exceptions should be thrown. Along with the methods listed in VendingMachineInterface.java, you must also write a constructor for VendingMachine whose header looks like this: public VendingMachine(int s, int t, int r) { As usual, this constructor initializes any private variables you have declared. Your VendingMachine must be initialized with s number of Snickers, t number of Twix, and r number of Reeses. You are allowed to use as many private methods and private variables as you want.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
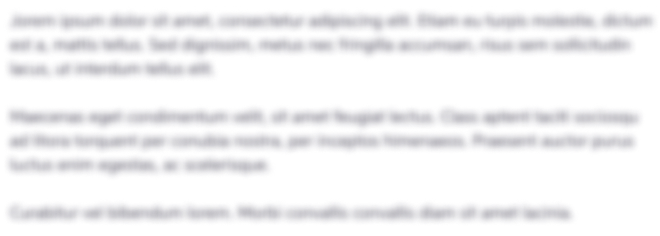
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started