Question
This assignment will give you practice using random numbers, functions, C-style arrays, array and vector classes, and different call passing mechanisms (by value, by reference
This assignment will give you practice using random numbers, functions, C-style arrays, array and vector classes, and different call passing mechanisms (by value, by reference using a reference, by reference using a pointer).
Write a C++ program that will create 3 collections (arrays). The size of each array should be 10 elements. Each array will store a random number in the range of 50 - 100. Use a ranged for loop to load each array with random numbers.
a c-style array | use the name array1 |
an array class object | use the name array2 |
a vector class object | use the name array3 |
After you have created and loaded the 3 arrays you will need to make copies of the three arrays. Give the copies the names array1_copy, array2_copy, and array3_copy.
Create 3 functions that will take a single integer and double it. The doubled value will be stored in the array being processed. Use loops to call the functions to double the values in the array elements.
1. The first function will have a return type of int and use call-by-value. | use on array1 function double1 |
2. The second function will have a return type of void and use call-be-reference using a reference. | use on array2 function double2 |
3. The third function will have a return type of void and use call-by-reference using a pointer. | use on array3 function double3 |
Create 3 functions that will take an integer array and double every element in the array. Use loops in the functions that are called to triple the values in the array elements.
1. The first function will have a return type of void and use call-by-reference using a c-style array. Use [ ] in the function header: void triple1( int array1[ ], int size ) | use on array1_copy function triple1 |
2. The second function will have a return type of void and use call-be-reference using a reference | use on array2_copy function triple2 |
3. The third function will have a return type of void and use call-by-reference using a pointer. | use on array3_copy function triple3 |
Here is the sequence you should follow:
1. Create the 3 arrays. Print out each array on a single line nicely formatted [hint - use the setw() stream manipulator]
2. Double the elements in the original 3 arrays using the first set of 3 functions. Print out each array on a single line nicely formatted
3. Triple the elements in the copied 3 arrays using the second set of 3 functions. Print out each array on a single line nicely formatted
Note: You may use traditional random number generation functions or use the new C++2011 more finely tuned random number generation functions.
Note: Do NOT use a loop to make the copies of the array and vector objects. Copies can be made using the assignment operator or using the copy constructor! Use the new C++2011 ranged for loop instead of the traditional for loop with 3 expressions for ALL loops except for loops working with a c-style (aka built-in) array. A ranged for loop won't work with a c-style array passed to a function.
Here are the function prototypes for the double and triple functions you may use in your program:
int double1 (int number); void double2 (int &number); void double3 (int *number); void triple1 (int array1[], int size); void triple2 (array &array2); void triple3 (vector *array3);
Arrays after loading them with random numbers in the range [50,100]: array1: arra array3: 1: 91 70 64 519388 63 845468 y2: 82 51 69 786075 76 84 65 72 3: 92 95 71 8687 84 69 72 7391 Arrays after calling a function element by element to double each element in the arrays. 1 182 140 128 102 186 176 126 168 108 136 arrayl: array2: 164102 138 156 120 150 152 168 130 144 arra y3 184 190 142 172174 168 138 144 146 182 Arrays after passing them to a function to triple each element in the arrays: 1: 273 210 192 153 279 264 189 252 162 204 arraVI: array2:246 153 207 234 180 225 228 252 195 216 array3: y3 276 285 213 258 261 252 207 216 219 273 Press any key to continueStep by Step Solution
There are 3 Steps involved in it
Step: 1
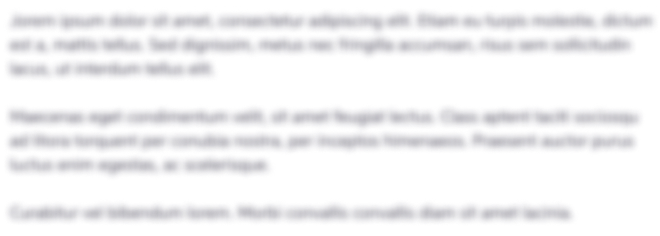
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started