Answered step by step
Verified Expert Solution
Question
1 Approved Answer
/** * This class contains methods to parse text, specifically intended to eventually parse CSV (comma separated values) * files. * * Definitions of Terms:
/** * This class contains methods to parse text, specifically intended to eventually parse CSV (comma separated values) * files. * * Definitions of Terms: * delimiter: The character used to specify the boundary between fields in a sequence of characters. * for example, the comma would be the delimiter between the fields: * name, id, section * Note the field values include the spaces. In general, the field value is every character except * for the delimiter. * * qualifier: If a field value contains a delimiter such as a comma with the name field: * John Doe, MD, 1234567, 321 * then a qualifier, such as ", is placed around a field value to indicate it is the same field. * for example: * "John Doe, MD", 1234567, 321 * If the qualifier itself is a part of the field value then the qualifier is duplicated. * for example for the field value: * H. Jackson Brown, Jr. said "The best preparation for tomorrow is doing your best today." * would be enclosed with " and internal quotes duplicated resulting in the qualified field value: * "H. Jackson Brown, Jr. said ""The best preparation for tomorrow is doing your best today.""" * * A example with , as delimiter and " as qualifier with a newline in a field value should be * processed as follows: * "This is a line of text.",bbb,ccc * would have 3 fields * "This is a line of text." * bbb * ccc * * This project is derived from RFC 4180: https://tools.ietf.org/html/rfc4180 * */ public class CSVParser { /** * This method returns the array of string containing the field values. * The field values in text are identified using , as the delimiter. * Qualifiers are not considered at all in the parsing of text. * * Example: * aaa,bbb,ccc * should result in an array with 3 fields * aaa * bbb * ccc * * aaa,bbb, * should also result in an array with 3 fields with the 3rd field containing either whitespace or no spaces * depending on the actual characters after the last ,. * * If text is null then a zero length array is returned. If text is non-null * but no delimiters are found then a single length array is returned with the text as the field value. * * @param text A sequence of characters that may include , as a delimiter. * @return An array of String containing each field value. The size of the * array is the number of fields found. */ public static String[] separate(String text) { //algorithm: //determine the number of fields by counting the number of , in the text string. //create an array of String for the number of fields //add each field value to the corresponding array index. //return the array of field values return null; //FIXME in Part1 } /** * This method is the same as separate(String text) except that any character can be used as the delimiter * specified as a parameter. In this method, qualifiers are not considered at all in the parsing of text. * * Example: * aaa,bbb,ccc ddd,eee,fff * with as the delimiter should result in an array with 2 fields * aaa,bbb,ccc * ddd,eee,fff * * @param text A sequence of characters. * @param delimiter The character that separates field values in text. * @return An array of String containing each field value. The size of the * array is the number of fields found. * */ public static String[] separate(String text, char delimiter) { //revise your code from the previous method to use the character passed in the delimiter //parameter rather than using ','. return null; //FIXME in Part1 } /** * This method extends the previous separate methods in that qualifiers are now considered. See the class * header comment for examples of qualifier. Qualifiers are kept as a part of the field values. * * Example: * "H. Jackson Brown, Jr. said ""The best preparation for tomorrow is doing your best today.""",bbb * With delimiter , and qualifier " would result in the following field values: * "H. Jackson Brown, Jr. said ""The best preparation for tomorrow is doing your best today.""" * bbb * * @param text A sequence of characters. * @param delimiter The character that separates field values in text. * @param qualifier The character indicating the beginning and ending of the field value such * that delimiters could be within a field value. * @return An array of String containing each field value. The size of the * array is the number of fields found. * */ public static String[] separate(String text, char delimiter, char qualifier) { return null; //FIXME in Part2 } /** * This method extends the previous by adding the parameter keepQualifiers. If keepQualifiers is true * the returned field values include the qualifier characters. If keepQualifiers is false then the * returned field values have the qualifier characters removed. * * Example: * "H. Jackson Brown, Jr. said ""The best preparation for tomorrow is doing your best today.""", bbb * With delimiter , and qualifier " and keepQualifiers false would result in the following field values: * H. Jackson Brown, Jr. said "The best preparation for tomorrow is doing your best today." * bbb * * @param text A sequence of characters. * @param delimiter The character that separates field values in text. * @param qualifier The character indicating the beginning and ending of the field value such * that delimiters could be within a field value. * @param keepQualifiers true to keep qualifiers, false to remove qualifiers * @return An array of String containing each field value. The size of the * array is the number of fields found. */ public static String[] separate(String text, char delimiter, char qualifier, boolean keepQualifiers) { return null; //FIXME in Part3 } }
*****Here are the test cases, if they work, it shall mean that the program is working. Test cases are all made and they are perfect.
/** * This file contains testing methods for the CSVParser project. * These methods are intended to serve several purposes: * 1) provide an example of a way to incrementally test your code * 2) provide example method calls for the various methods in CSVParser * 3) provide examples of creating and accessing arrays */ import java.util.Arrays; /** * This class contains methods for testing CSVParser methods as they are developed or modified. * These methods are all private as they are only intended for use within this class. * * @author Jim Williams * @author FIXME add your name here if you modify or extend the tests * */ public class TestCSVParser { /** * This is the main method that runs the various tests. Uncomment the tests * when you are ready for them to run. * * @param args (unused) */ public static void main(String[] args) { testParserPart1(); // testParserPart2(); // testParserPart3(); } /** * A testing method for part 1 of CSV Parser project * Part 1 implementation separates based on comma and then generalizes to any character, but does not consider " * or any qualifier characters. */ private static void testParserPart1() { boolean error = false; String input = null; String[] expectedResult = new String[0]; String[] actualResult = CSVParser.separate(input); if (checkForError("testParserPart1.1", expectedResult, actualResult)) { error = true; } input = ""; expectedResult = new String[] {""}; actualResult = CSVParser.separate(input); if (checkForError("testParserPart1.2", expectedResult, actualResult)) { error = true; } input = " a "; expectedResult = new String[] {" a "}; actualResult = CSVParser.separate(input); if (checkForError("testParserPart1.3", expectedResult, actualResult)) { error = true; } input = "aaa,bbb,ccc"; expectedResult = new String[] {"aaa", "bbb", "ccc"}; actualResult = CSVParser.separate(input); if (checkForError("testParserPart1.4", expectedResult, actualResult)) { error = true; } input = "aaa,bbb,"; expectedResult = new String[] {"aaa", "bbb", ""}; actualResult = CSVParser.separate(input); if (checkForError("testParserPart1.5", expectedResult, actualResult)) { error = true; } input = " aa a, bbb , cc c "; expectedResult = new String[] {" aa a", " bbb ", " cc c "}; actualResult = CSVParser.separate(input); if (checkForError("testParserPart1.6", expectedResult, actualResult)) { error = true; } input = "aaa,bbb,ccc zzz,yyy,xxx"; expectedResult = new String[] {"aaa,bbb,ccc", "zzz,yyy,xxx"}; actualResult = CSVParser.separate(input, ' '); if (checkForError("testParserPart1.7", expectedResult, actualResult)) { error = true; } if (error) { System.err.println("testParserPart1 failed"); } else { System.out.println("testParserPart1 passed"); } } /** * A testing method for part 2 of CSV Parser project * Part 2 methods add a qualifier, such as ", so that delimiter characters can be within field values. * */ private static void testParserPart2() { boolean error = false; String input = null; String[] expectedResult = new String[0]; String[] actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.1", expectedResult, actualResult)) { error = true; } input = ""; expectedResult = new String[] {""}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.2", expectedResult, actualResult)) { error = true; } input = " a "; expectedResult = new String[] {" a "}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.3", expectedResult, actualResult)) { error = true; } input = "aaa,bbb,ccc"; expectedResult = new String[] {"aaa", "bbb", "ccc"}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.4", expectedResult, actualResult)) { error = true; } input = "aaa,bbb,"; expectedResult = new String[] {"aaa", "bbb", ""}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.5", expectedResult, actualResult)) { error = true; } input = " aa a, bbb , cc c "; expectedResult = new String[] {" aa a", " bbb ", " cc c "}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.6", expectedResult, actualResult)) { error = true; } input = "aaa,bbb,ccc zzz,yyy,xxx"; expectedResult = new String[] {"aaa,bbb,ccc", "zzz,yyy,xxx"}; actualResult = CSVParser.separate(input, ' ', '"'); if (checkForError("testParserPart2.7", expectedResult, actualResult)) { error = true; } input = "field_name,field_name,field_name aaa,bbb,ccc zzz,yyy,xxx"; expectedResult = new String[] {"field_name,field_name,field_name", "aaa,bbb,ccc", "zzz,yyy,xxx"}; actualResult = CSVParser.separate(input, ' ', '"'); if (checkForError("testParserPart2.8", expectedResult, actualResult)) { error = true; } input = "\"aaa\",\"bbb\",\"ccc\""; // recall in Java, to have " within String literals we // have to precede with \ expectedResult = new String[] {"\"aaa\"", "\"bbb\"", "\"ccc\""}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.9", expectedResult, actualResult)) { error = true; } input = "\"a, aa\",\"b ,bb\",\"cc , c\""; expectedResult = new String[] {"\"a, aa\"", "\"b ,bb\"", "\"cc , c\""}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.10", expectedResult, actualResult)) { error = true; } input = "\"a\"\",aa\",\"b ,\"\"bb\",\"cc , c\""; expectedResult = new String[] {"\"a\"\",aa\"", "\"b ,\"\"bb\"", "\"cc , c\""}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.11", expectedResult, actualResult)) { error = true; } input = "\"aaa\",\"b bb\",\"ccc\""; expectedResult = new String[] {"\"aaa\"", "\"b bb\"", "\"ccc\""}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.12", expectedResult, actualResult)) { error = true; } input = "\"John Doe, MD\", 1234567, 321"; expectedResult = new String[] {"\"John Doe, MD\"", " 1234567", " 321"}; actualResult = CSVParser.separate(input, ',', '"'); if (checkForError("testParserPart2.13", expectedResult, actualResult)) { error = true; } if (error) { System.err.println("testParserPart2 failed"); } else { System.out.println("testParserPart2 passed"); } } /** * A testing method for part 3 of CSV Parser project * Part 3 methods add a field keepQualifiers that specify whether the field values should * contain the qualifier characters or not. * */ private static void testParserPart3() { boolean error = false; String input = null; String[] expectedResult = new String[0]; String[] actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.1", expectedResult, actualResult)) { error = true; } input = ""; expectedResult = new String[] {""}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.2", expectedResult, actualResult)) { error = true; } input = " a "; expectedResult = new String[] {" a "}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.3", expectedResult, actualResult)) { error = true; } input = "aaa,bbb,ccc"; expectedResult = new String[] {"aaa", "bbb", "ccc"}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.4", expectedResult, actualResult)) { error = true; } input = "aaa,bbb,"; expectedResult = new String[] {"aaa", "bbb", ""}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.5", expectedResult, actualResult)) { error = true; } input = " aa a, bbb , cc c "; expectedResult = new String[] {" aa a", " bbb ", " cc c "}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.6", expectedResult, actualResult)) { error = true; } input = "aaa,bbb,ccc zzz,yyy,xxx"; expectedResult = new String[] {"aaa,bbb,ccc", "zzz,yyy,xxx"}; actualResult = CSVParser.separate(input, ' ', '"', false); if (checkForError("testParserPart3.7", expectedResult, actualResult)) { error = true; } input = "field_name,field_name,field_name aaa,bbb,ccc zzz,yyy,xxx"; expectedResult = new String[] {"field_name,field_name,field_name", "aaa,bbb,ccc", "zzz,yyy,xxx"}; actualResult = CSVParser.separate(input, ' ', '"', false); if (checkForError("testParserPart3.8", expectedResult, actualResult)) { error = true; } input = "\"aaa\",\"bbb\",\"ccc\""; // recall in Java, to have " within String literals we // have to precede with \ expectedResult = new String[] {"\"aaa\"", "\"bbb\"", "\"ccc\""}; actualResult = CSVParser.separate(input, ',', '"', true); if (checkForError("testParserPart3.9T", expectedResult, actualResult)) { error = true; } input = "\"aaa\",\"bbb\",\"ccc\""; // recall in Java, to have " within String literals we // have to precede with \ expectedResult = new String[] {"aaa", "bbb", "ccc"}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.9F", expectedResult, actualResult)) { error = true; } input = "\"a, aa\",\"b ,bb\",\"cc , c\""; expectedResult = new String[] {"\"a, aa\"", "\"b ,bb\"", "\"cc , c\""}; actualResult = CSVParser.separate(input, ',', '"', true); if (checkForError("testParserPart3.10T", expectedResult, actualResult)) { error = true; } input = "\"a, aa\",\"b ,bb\",\"cc , c\""; expectedResult = new String[] {"a, aa", "b ,bb", "cc , c"}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.10F", expectedResult, actualResult)) { error = true; } input = "\"a\"\",aa\",\"b ,\"\"bb\",\"cc , c\""; expectedResult = new String[] {"\"a\"\",aa\"", "\"b ,\"\"bb\"", "\"cc , c\""}; actualResult = CSVParser.separate(input, ',', '"', true); if (checkForError("testParserPart3.11T", expectedResult, actualResult)) { error = true; } input = "\"a\"\",aa\",\"b ,\"\"bb\",\"cc , c\""; expectedResult = new String[] {"a\",aa", "b ,\"bb", "cc , c"}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.11F", expectedResult, actualResult)) { error = true; } input = "\"aaa\",\"b bb\",\"ccc\""; expectedResult = new String[] {"\"aaa\"", "\"b bb\"", "\"ccc\""}; actualResult = CSVParser.separate(input, ',', '"', true); if (checkForError("testParserPart3.12T", expectedResult, actualResult)) { error = true; } input = "\"aaa\",\"b bb\",\"ccc\""; expectedResult = new String[] {"aaa", "b bb", "ccc"}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.12F", expectedResult, actualResult)) { error = true; } input = "\"John Doe, MD\", 1234567, 321"; expectedResult = new String[] {"\"John Doe, MD\"", " 1234567", " 321"}; actualResult = CSVParser.separate(input, ',', '"', true); if (checkForError("testParserPart3.13T", expectedResult, actualResult)) { error = true; } input = "\"John Doe, MD\", 1234567, 321"; expectedResult = new String[] {"John Doe, MD", " 1234567", " 321"}; actualResult = CSVParser.separate(input, ',', '"', false); if (checkForError("testParserPart3.13F", expectedResult, actualResult)) { error = true; } if (error) { System.err.println("testParserPart3 failed"); } else { System.out.println("testParserPart3 passed"); } } /** * A supporting test method that compares the expected and actual results of calling a method. * This method returns true if a test fails and false if the test case succeeds. * * @param testName The name of the test to include in the output * @param expected The expected return value from the separate method. * @param actual The actual return value from the separate method. * @return true if error, false if no error detected. */ private static boolean checkForError( String testName, String[] expected, String[] actual) { boolean error = false; if (expected == null || actual == null) { System.err.println( testName + "a: actual=" + (actual == null ? "null" : Arrays.toString(actual)) + " expected=" + (expected == null ? "null" : Arrays.toString(expected))); error = true; } else if (expected.length != actual.length) { System.err.println(testName + "b: actual=" + Arrays.toString(actual) + " expected=" + Arrays.toString(expected)); error = true; } for (int i = 0; !error && i < expected.length; i++) { if (!expected[i].equals(actual[i])) { error = true; System.err.println(testName + "c: actual[" + i + "]=" + actual[i] + " expected[" + i + "]=" + expected[i]); } } return error; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
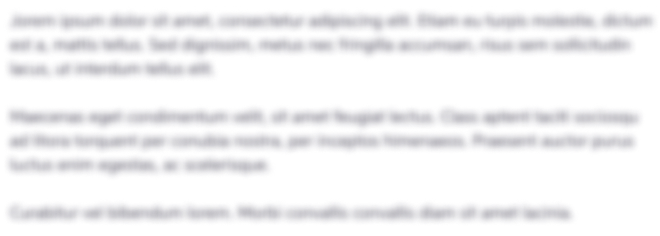
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started