Question
/* This class encapsulates a list of user-defined items that should be done- a TODO list. * Each item on the list is represented by
/* This class encapsulates a list of user-defined items that should be done- a "TODO" list. * Each item on the list is represented by a String object. * The list is implemented by a String array. The array is initialized in the constructor to an * initial length that is passed to the constructor. The initial array contains only NULL values. * A user adds an item (a String) to the list by calling the addItem method, passing in a String * that represents the to-do item. * Thus, the array may have fewer items (Strings) than its length. * * For example, assume the list has an initial length of 5. It looks like this: * NULL, NULL, NULL, NULL, NULL * * Then, a user adds three items. It looks like this: * "eat lunch", "walk dog", "study Java", NULL, NULL * * The length of the list is 5, the number of items is 3. The NULL values are unoccupied cells. * The capacity of the array is its length. The size of the data stored in the array is less than * or equal to its capacity. * * If a user wants to add more items to a list that has no more NULL values, i.e. no more room, * the expandArray method is called to double the length of the toDoList. The original Strings * are in the same positions, but the new array has double the capacity- more room for adding items. */ public class TODOList { /* YOUR Instance variable declarations here. */ /* Constructor that initializes the initialLength variable to the value passed in. * It also initializes the toDoList with the initial length. * Any other instance variables may be initialized as well. */ public TODOList(int initialLen){ } /* Add the item passed in to the end of the list. * For example, if the toDoList list contained: "eat lunch", "walk dog", * the next item added, "study Java", would result in this list: * "eat lunch", "walk dog", "study Java" * Suggestion: use instance variable to keep track of the index of the next available cell. */ public void addItem(String itemStr){ } /* Overwrite the item at "position" to be the parameter "itemStr". * Note: position is a positive integer > 0 that has to be a valid position * in the toDoList. A valid position corresponds to an item stored in the * toDoList. For example, if this was the list: 1 walk the cat 2 order doughnuts 3 go to the gym 4 wash dishes * valid positions would be 1, 2, 3, 4. All other integers are invalid. * This method returns true if a valid position was passed in, false otherwise. */ public boolean replaceItemAt(String itemStr, int position){ return false; } /* Remove the last item in the toDoList. * For example, if the toDoList list contained: "eat lunch", "walk dog", "study Java", * removing the last item would result in this list: * "eat lunch", "walk dog". * This method returns true if there is at least one item in the list, * false otherwise. */ public boolean removeLastItem(){ return false; } /* * Return a list that contains only the items that have been added. * This list does not contain any NULL values. * For example, if the toDoList list contained: "eat lunch", "walk dog", "study Java", NULL, NULL * This method would return this array: "eat lunch", "walk dog", "study Java" * If the toDoList does not contain any items, this method returns a String array with zero length. */ public String[] getToDoList(){ return null; } /* Remove all items from the list, resulting in an empty list. * The capacity of the list returns to the initial length. */ public void clearToDoList(){ } /* Returns a String representation of the current item list according to * these specifications: * Each item is on one line, position number first followed by one blank, * followed by the item String. * For example: 1 walk the cat 2 order doughnuts 3 go to the gym 4 wash dishes * If no items are on the list the String returned is: "no items". */ public String getToDoListAsString(){ return null; } /* Returns the number of items stored in the item list. */ public int getNumberOfItems(){ return 5000; } /* Returns true if the item list contains no items, false otherwise. */ public boolean isEmpty(){ return false; } /****** Private, "helper" method section ******/ /* Creates a new array that is double the size of the array passed in, copies the data * from that array to the new array, and returns the new array. * Note that the new array will contain the items from the previous array followed by NULL values. */ private String[] expandList(String[] inputList){ return null; } /* A full item list is an array where all cells contain an item. That * means there is no cell that contains NULL. * This method returns true if all cells in the array contain a String * object, false otherwise. */ private boolean isFull(){ return true; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
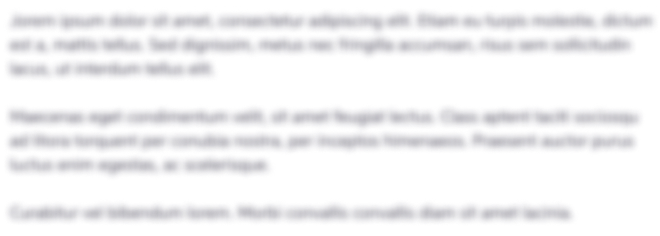
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started