Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
/ * * This class represents immutable rectangles in the plane. We say that an object is immutable if you cannot change it after it
This class represents "immutable" rectangles in the plane. We say that an object is "immutable" if you cannot change it after it is created. Whenever you wish to modify some aspect of a Rectangle, you actually get a new Rectangle in which the modification has been done. The fields of this class are both private and final. Private means they cannot be seen by any code outside of this class. The final modifier means that the values of those variables cannot be change by any code, even code in this class except that they can be initialized by the constructor public class Rectangle private final double x; xy is the upper lefthand corner of this rectangle private final double y; private final double width; private final double height; @param x xcoordinate of this Rectangle's upper lefthand corner @param y ycoordinate of this Rectangle's upper lefthand corner @param width width of this Rectangle, must be strictly positive @param height height of this Rectangle, must be strictly positive @throws IllegalArgumentException when width is not strictly positive @throws IllegalArgumentException when height is not strictly positive public Rectangledouble x double y double width, double height implementation @return the xcoordinate of this Rectangle's upper lefthand corner public double getX implementation @return the ycoordinate of this Rectangle's upper lefthand corner public double getY implementation @return the value of this Rectangle's width public double getWidth implementation @return the value of this Rectangle's height public double getHeight implementation @return the value of this Rectangle's area public double getArea implementation @return the value of this Rectangle's perimeter public double getPerimeter implementation Return a Rectangle object that has the same upper lefthand corner and height as this Rectangle but with the given width. @param width the width for the new rectangle @return a Rectangle with the given width and the same upper lefthand corner and height as this rectangle public Rectangle setWidthdouble width implementation Return a Rectangle object that has the same upper lefthand corner and width as this Rectangle but with the given height. @param height the height for the new rectangle @return a Rectangle with the given height and the same upper lefthand corner and width as this rectangle public Rectangle setHeightdouble height implementation Return a Rectangle object that has the same width and height as this Rectangle but the new Rectangle should have its upper lefthand corner at the given x and y values. @param x xcoordinate for the new Rectangle's upper lefthand corner @param y ycoordinate for the new Rectangle's upper lefthand corner @return a Rectangle with the same dimensions but a different upper lefthand corner public Rectangle moveTodouble x double y implementation Return a Rectangle object that has the same width and height as this Rectangle but the new Rectangle should have its upper lefthand corner translated from where this Rectangle's upper lefthand corner is @param dx amount by which to change the xcoordinate from this Rectangle @param dy amount by which to change the ycoordinate from this Rectangle @return a Rectangle with the same dimensions but a different upper lefthand corner public Rectangle translateBydouble dx double dy implementation Return a Rectangle object that has the same upper lefthand corner as this Rectangle, but the new Rectangle should have the width and height from this Rectangle flipped. @return a Rectangle with the same upper lefthand corner but with the dimensions flipped public Rectangle flip implementation Return a Rectangle object with the same upper lefthand corner as this Rectangle, a width that is the maximum of the widths from the two input Rectangles, and with a height that is the maximum of the heights from the two input Rectangles. @param r the first input Rectangle @param r the second input Rectangle @ret
This class represents "immutable" rectangles in the plane.
We say that an object is "immutable" if you cannot change
it after it is created.
Whenever you wish to modify some aspect of a Rectangle, you
actually get a new Rectangle in which the modification has
been done.
The fields of this class are both private and final. Private
means they cannot be seen by any code outside of this class.
The final modifier means that the values of those variables
cannot be change by any code, even code in this class except
that they can be initialized by the constructor
public class Rectangle
private final double x; xy is the upper lefthand corner of this rectangle
private final double y;
private final double width;
private final double height;
@param x xcoordinate of this Rectangle's upper lefthand corner
@param y ycoordinate of this Rectangle's upper lefthand corner
@param width width of this Rectangle, must be strictly positive
@param height height of this Rectangle, must be strictly positive
@throws IllegalArgumentException when width is not strictly positive
@throws IllegalArgumentException when height is not strictly positive
public Rectangledouble x double y double width, double height
implementation
@return the xcoordinate of this Rectangle's upper lefthand corner
public double getX
implementation
@return the ycoordinate of this Rectangle's upper lefthand corner
public double getY
implementation
@return the value of this Rectangle's width
public double getWidth
implementation
@return the value of this Rectangle's height
public double getHeight
implementation
@return the value of this Rectangle's area
public double getArea
implementation
@return the value of this Rectangle's perimeter
public double getPerimeter
implementation
Return a Rectangle object that has the same upper lefthand
corner and height as this Rectangle but with the given width.
@param width the width for the new rectangle
@return a Rectangle with the given width and the same upper lefthand corner and height as this rectangle
public Rectangle setWidthdouble width
implementation
Return a Rectangle object that has the same upper lefthand
corner and width as this Rectangle but with the given height.
@param height the height for the new rectangle
@return a Rectangle with the given height and the same upper lefthand corner and width as this rectangle
public Rectangle setHeightdouble height
implementation
Return a Rectangle object that has the same width and height
as this Rectangle but the new Rectangle should have its upper
lefthand corner at the given x and y values.
@param x xcoordinate for the new Rectangle's upper lefthand corner
@param y ycoordinate for the new Rectangle's upper lefthand corner
@return a Rectangle with the same dimensions but a different upper lefthand corner
public Rectangle moveTodouble x double y
implementation
Return a Rectangle object that has the same width and height
as this Rectangle but the new Rectangle should have its upper
lefthand corner translated from where this Rectangle's upper
lefthand corner is
@param dx amount by which to change the xcoordinate from this Rectangle
@param dy amount by which to change the ycoordinate from this Rectangle
@return a Rectangle with the same dimensions but a different upper lefthand corner
public Rectangle translateBydouble dx double dy
implementation
Return a Rectangle object that has the same upper lefthand
corner as this Rectangle, but the new Rectangle should have
the width and height from this Rectangle flipped.
@return a Rectangle with the same upper lefthand corner but with the dimensions flipped
public Rectangle flip
implementation
Return a Rectangle object with the same upper lefthand
corner as this Rectangle, a width that is the maximum
of the widths from the two input Rectangles, and with
a height that is the maximum of the heights from the
two input Rectangles.
@param r the first input Rectangle
@param r the second input Rectangle
@ret
Step by Step Solution
There are 3 Steps involved in it
Step: 1
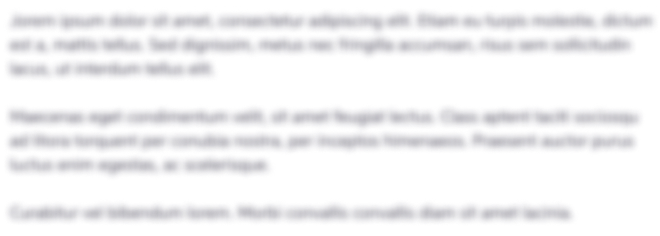
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started