Question
This code is wrong I need some help with it Instructions The following program takes a string and builds an index of it. The index
This code is wrong I need some help with it
Instructions
The following program takes a string and builds an index of it. The index indicates the:
Start of each line in the string,
Start of each word in the string,
Start of each number in the string.
#pragma once
#ifndef STRINGHELP_H
#define STRINGHELP_H
#define MAX_STRING_SIZE 511
#define MAX_INDEX_SIZE 100
#define MAX_WORD_SIZE 23
struct StringIndex
{
char str[MAX_STRING_SIZE + 1];
int wordStarts[MAX_INDEX_SIZE];
int lineStarts[MAX_INDEX_SIZE];
int numberStarts[MAX_INDEX_SIZE];
int numWords, numLines, numNumbers;
};
int nextWhite(const char* str);
int isNumber(const char* str, const int len);
struct StringIndex indexString(const char* str);
int getNumberLines(const struct StringIndex* idx);
int getNumberWords(const struct StringIndex* idx);
int getNumberNumbers(const struct StringIndex* idx);
void getWord(char word[], const struct StringIndex* idx, int wordNum);
void getNumber(char word[], const struct StringIndex* idx, int numberNum);
char* getLine(struct StringIndex* idx, int lineNum);
void printUntil(const char* s, const int start, const char terminator);
void printUntilSpace(const char* s, const int start);
#endif
stringhelp.c
#define _CRT_SECURE_NO_WARNINGS
#include "stringhelp.h"
#include
#include
int nextWhite(const char* str)
{ int i, result = -1;
for (i = 0; result < 0 && str[i] != '\0'; i++) {
if (isspace(str[i]))
{ result = i;
} }
return (result < 0) ? i : result; }
int isNumber(const char* str, const int len)
{ int i, result = 1;
for (i = 0; i < len && result; i++)
{ result = result && isdigit(str[i]);
}
return result; }
struct StringIndex indexString(const char* str)
{ struct StringIndex result = { {0}, {0}, {0}, 0, 0, 0 }; int i, sp;
strcpy(result.str, str); if (str[0] != '\0')
{ result.lineStarts[0] = 0;
result.numLines = 1; }
for (i = 0; str[i] != '\0'; i++)
{ while (str[i] != '\0' && isspace(str[i]))
{ if (str[i] == ' ')
{ result.lineStarts[result.numLines] = i + 1;
}
i++; }
sp = nextWhite(str + i);
if (isNumber(str + i, sp - i + 1))
{ result.numberStarts[result.numNumbers++] = i;
}
else
{ result.wordStarts[result.numWords++] = i;
}
i += sp - 1; }
return result; }
int getNumberLines(const struct StringIndex* idx)
{ return idx->numLines;
}
int getNumberWords(const struct StringIndex* idx)
{ return idx->numWords;
}
int getNumberNumbers(const struct StringIndex* idx)
{ return idx->numNumbers;
}
void getWord(char word[], const struct StringIndex* idx, int wordNum)
{ int i, sp, start;
word[0] = '\0'; if (wordNum < idx->numWords && wordNum >= 0)
{ start = idx->wordStarts[wordNum]; sp = nextWhite(idx->str + start); for (i = 0; i < sp; i++) {
word[i] = idx->str[i]; }
word[sp] = '\0';
((struct StringIndex*)idx)->numWords--; }
}
void getNumber(char word[], const struct StringIndex* idx, int numberNum)
{ int i, sp, start;
word[0] = '\0'; if (numberNum < idx->numNumbers && numberNum >= 0) {
start = idx->numberStarts[numberNum]; sp = nextWhite(idx->str + start); for (i = 0; i < sp; i++) {
word[i] = idx->str[start + i]; }
word[sp] = '\0'; }
}
char* getLine(struct StringIndex* idx, int lineNum)
{ char* result = NULL;
if (lineNum < idx->numLines && lineNum >= 0)
{ result = idx->str + idx->lineStarts[lineNum];
}
return result; }
void printUntil(const char* s, const int start, const char terminator)
{ int i;
for (i = start; s[i] != '\0' && s[i] != terminator; i++) printf("%c", s[i]);
}
void printUntilSpace(const char* s, const int start)
{ int i;
for (i = start; s[i] != '\0' && !isspace(s[i]); i++) printf("%c", s[i]);
}
main.c
#define _CRT_SECURE_NO_WARNINGS
#include
#include
#include
int main(void)
{ char testStr[] = { "This is a string with embedded newline
characters and 12345 numbers inside it as well 7890" };
struct StringIndex index = indexString(testStr); int i;
printf("LINES "); for (i = 0; i < index.numLines; i++)
{ printUntil(index.str, index.lineStarts[i], ' ');
printf(" "); }
printf(" WORDS "); for (i = 0; i < index.numWords; i++)
{ printUntilSpace(index.str, index.wordStarts[i]);
printf(" "); }
printf(" NUMBERS "); for (i = 0; i < index.numNumbers; i++)
{ printUntilSpace(index.str, index.numberStarts[i]);
printf(" ");
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
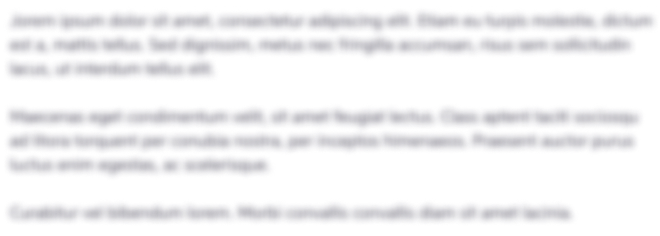
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started