this course called data structure and algorithm do as in the screenshot and use the following code for it and do part 1 2 3 4 and 5 everything you need to know is in the screenshots thanks
#include #include using namespace std; class CPUProcess{ private: string process_name; . . . public: CPUProcess(string pn,...){ process_name = pn; . . . } void printProcessInfo(){ .... } }; template class ProcessNode{ public: ..... } template class StackLL{ ProcessNode* head; ProcessNode* top; public: StackLL(){ head = NULL; top = NULL; } bool pushProcess(T process){ ProcessNode* newNode = new ProcessNode(process); .... } CPUProcess lateProcess(){ .... } . . . . }; template class QueueLL{ ProcessNode* head; ProcessNode* front; ProcessNode* rear; int capacity = 3; public: QueueLL(){ head = NULL; front = NULL; rear = NULL; } bool execute(T process){ ProcessNode* newNode = new ProcessNode(process); .... } void cpuSchedule(){ .... } void killProcess(){ // You need to create an object of type StackLL so that you can // call the pushProcess(T process) method here. .... } . . . . };
// If you will solve the bonus part (Binary Tree) your code will be below // // //
int main() { CPUProcess p1(1,"p1",10); CPUProcess p2(2,"p2",4); CPUProcess p3(3,"p3",1); CPUProcess p4(4,"p4",5); CPUProcess p5(5,"p5",15); QueueLL qll; qll.execute(p1); qll.execute(p2); qll.execute(p3); qll.execute(p4); qll.execute(p5); qll.cpuSchedule(); qll.killProcess(); StackLL sll; sll.stackOfProcesses(); sll.lateProcess().printProcessInfo(); return 0; }
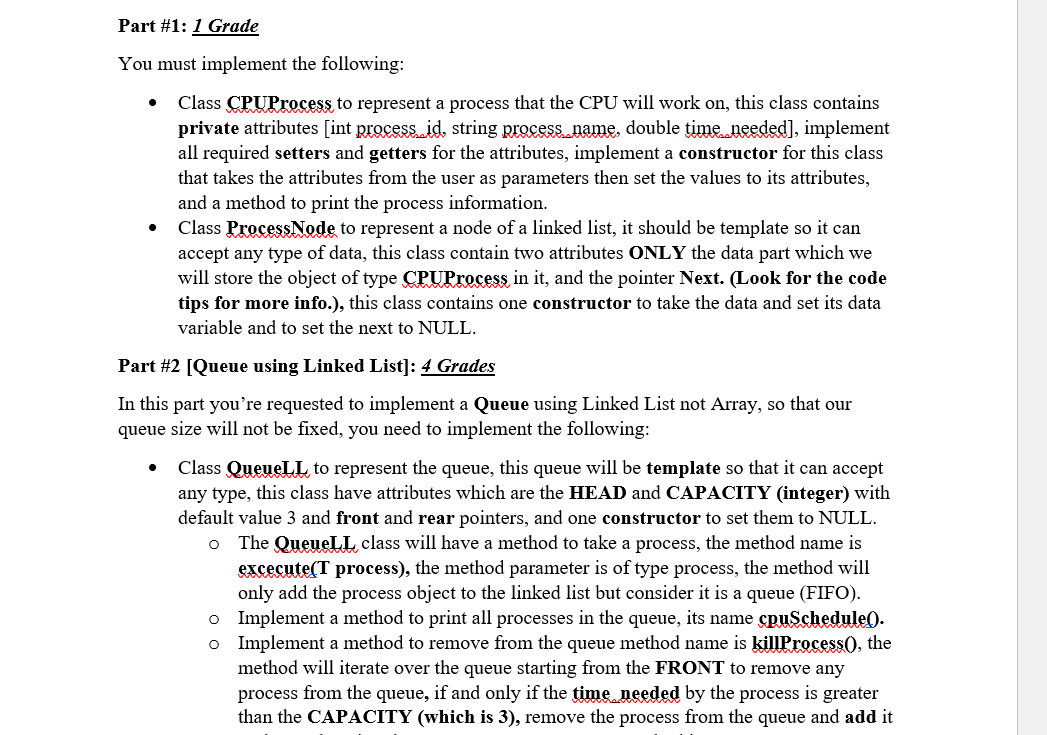
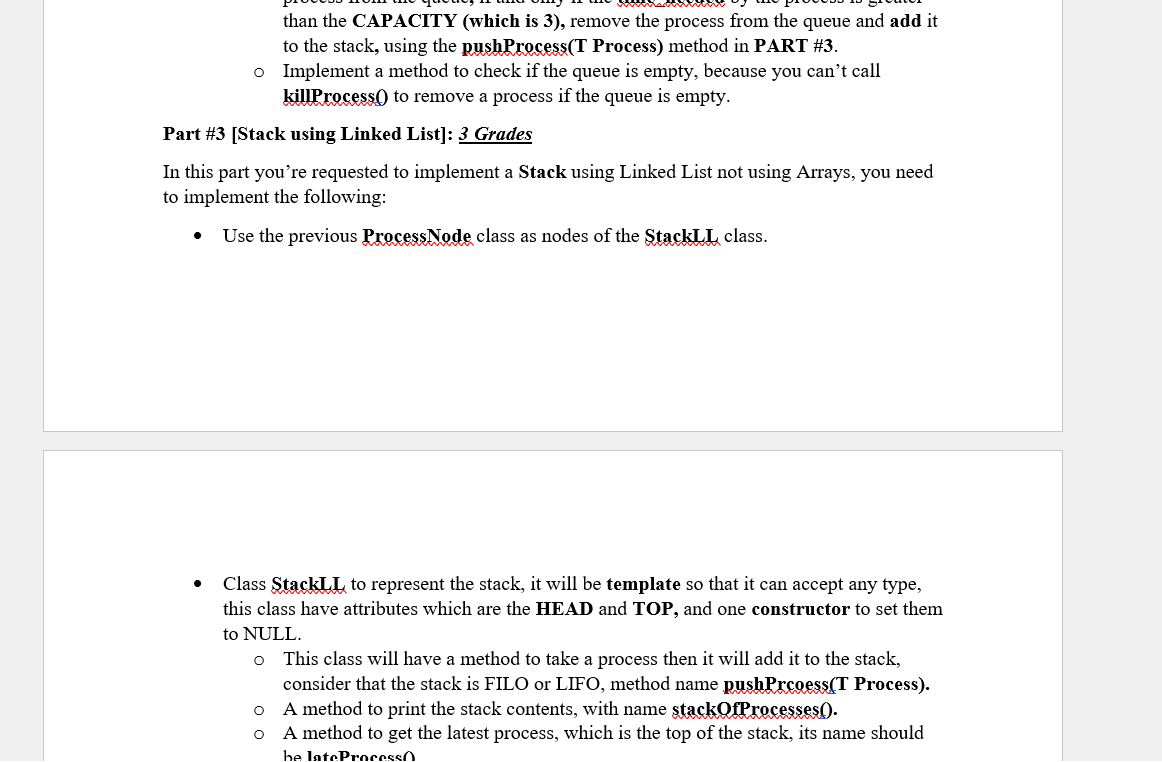
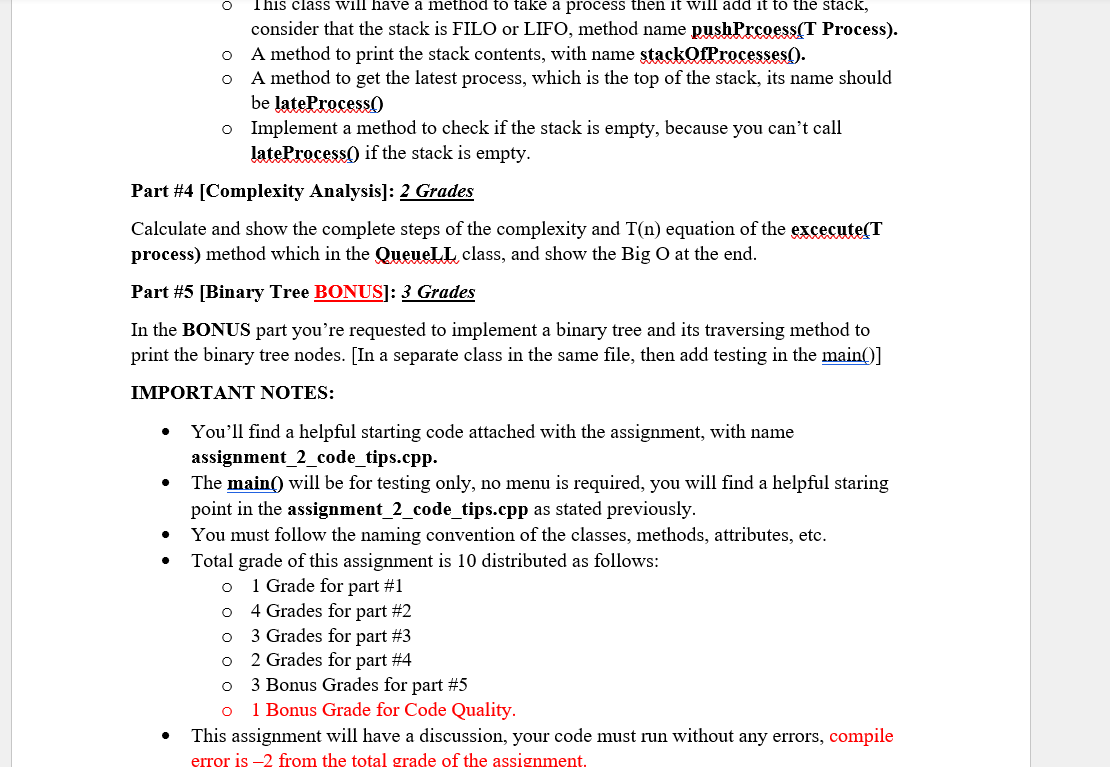
Part \#1: 1 Grade You must implement the following: - Class CPUProcess to represent a process that the CPU will work on, this class contains private attributes [int process id, string process name, double time needed], implement all required setters and getters for the attributes, implement a constructor for this class that takes the attributes from the user as parameters then set the values to its attributes, and a method to print the process information. - Class ProcessNode to represent a node of a linked list, it should be template so it can accept any type of data, this class contain two attributes ONLY the data part which we will store the object of type CPUProcess in it, and the pointer Next. (Look for the code tips for more info.), this class contains one constructor to take the data and set its data variable and to set the next to NULL. Part \#2 [Queue using Linked List]: 4 Grades In this part you're requested to implement a Queue using Linked List not Array, so that our queue size will not be fixed, you need to implement the following: - Class QueueLL to represent the queue, this queue will be template so that it can accept any type, this class have attributes which are the HEAD and CAPACITY (integer) with default value 3 and front and rear pointers, and one constructor to set them to NULL. - The QueueLL class will have a method to take a process, the method name is excecute(T process), the method parameter is of type process, the method will only add the process object to the linked list but consider it is a queue (FIFO). - Implement a method to print all processes in the queue, its name cpuSchedule 0 . - Implement a method to remove from the queue method name is killProcess0, the method will iterate over the queue starting from the FRONT to remove any process from the queue, if and only if the time needed by the process is greater than the CAPACITY (which is 3 ), remove the process from the queue and add it than the CAPACITY (which is 3 ), remove the process from the queue and add it to the stack, using the pushProcess(T Process) method in PART \#3. - Implement a method to check if the queue is empty, because you can't call killProcess) to remove a process if the queue is empty. Part \#3 [Stack using Linked List]: 3Grades In this part you're requested to implement a Stack using Linked List not using Arrays, you need to implement the following: - Use the previous ProcessNode class as nodes of the StackLL class. - Class StackLL to represent the stack, it will be template so that it can accept any type, this class have attributes which are the HEAD and TOP, and one constructor to set them to NULL. - This class will have a method to take a process then it will add it to the stack, consider that the stack is FILO or LIFO, method name pushPrcoess(T Process). - A method to print the stack contents, with name stackQfProcesses(). - A method to get the latest process, which is the top of the stack, its name should consider that the stack is FILO or LIFO, method name pushPrcoess(T Process). - A method to print the stack contents, with name stackQfProcesses(). - A method to get the latest process, which is the top of the stack, its name should be lateProcesso - Implement a method to check if the stack is empty, because you can't call lateProcess) if the stack is empty. Part \#4 [Complexity Analysis]: 2 Grades Calculate and show the complete steps of the complexity and T(n) equation of the excecute(T process) method which in the QueuelL class, and show the Big O at the end. Part \#5 [Binary Tree BONUS]: 3 Grades In the BONUS part you're requested to implement a binary tree and its traversing method to print the binary tree nodes. [In a separate class in the same file, then add testing in the main()] IMPORTANT NOTES: - You'll find a helpful starting code attached with the assignment, with name assignment_2_code_tips.cpp. - The main() will be for testing only, no menu is required, you will find a helpful staring point in the assignment_2_code_tips.cpp as stated previously. - You must follow the naming convention of the classes, methods, attributes, etc. - Total grade of this assignment is 10 distributed as follows: - 1 Grade for part \#1 - 4 Grades for part \#2 - 3 Grades for part \#3 - 2 Grades for part \#4 - 3 Bonus Grades for part \#5 - 1 Bonus Grade for Code Quality. - This assignment will have a discussion, your code must run without any errors, compile error is 2 from the total grade of the assignment