Question
This exercise asks you to implement a small class that will later serve as part of larger programs. You will implement a new kind of
This exercise asks you to implement a small class that will later serve as part of larger programs. You will implement a new kind of number, a BoundedInteger.
When we write programs to solve problems in the world, we often need to model particular kinds of values, and those values don't match up with the primitive types in our program language. For example, if we were writing a program to implement a clock or a calendar, then we would need numbers to represent minutes or days. But these numbers aren't Java integers, which range from -231to 231-1; they range from 0 to 59 and 1 to 31 (or less!), respectively. Java's base types are useful building blocks, but at the level of atoms, not molecules.
Tasks
Write a class for representing bounded integers.
A bounded integer takes integer values within a given range. For example, the minutes part of a time takes values in the range [0..59]. The next minute after 59 is 0. The minute before 0 is 59.
Object creation
We want to be able to create bounded integers in two ways.
We specify the object's lower bound, its upper bound, and its initial value.
We specify only the object's lower bound and its upper bound. The object's
initial value is the same as its lower bound.
Of course, this means that you need to write two constructors.
We would like to be able to use bounded integers in much the same way we use regular ints.
Arithmetic operations
We would like to be able to:
add an int to a bounded integer.
subtract an int from it.
increment and decrement it.
A bounded integer "wraps around" when it does such arithmetic. For example, suppose that we have a minutes bounded integer whose value is 52. Adding 10 to this object gives it a value of 2.
Value operations
We would like to be able to:
ask a bounded integer for its value as an int.
change its value to an arbitrary int.
print its value out. The way to do this in Java is for the object to respond
to toString() message by returning a String that can be printed. If we try to set a bounded integer to a value outside of its range, the object should
keep its current value and print an error message to System.err. or System.out. Comparison operations We would like to be able to:
ask one bounded integer if it is equal to another.
ask one bounded integer if it is less than another.
ask a bounded integer if a particular int is a legal value for the bounded integer. For example, 23 is a legal value for the minutes bounded integer, but 67 is not.
The answer to each of these questions is true or false.
You may implement these requirements in any order you choose, though you'll need at least one constructor before you can test any of the others. Write a class to test your code and submit that test class as well.
Some Suggestions for Coding:
To write your program, first write a test that expresses what you would like your code to do, and then write the code to do it. Take small steps, and your tests will give you feedback as soon as possible.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
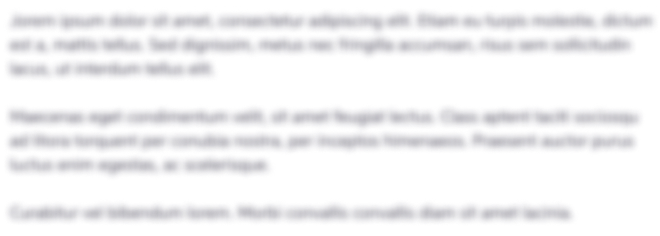
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started