Question
This exercise expands upon the discussion of Regular Expressions from Chapter 9 in the book. Java has a built in regex library for regular expressions.
This exercise expands upon the discussion of Regular Expressions from Chapter 9 in the book. Java has a built in "regex" library for regular expressions. For more information on how to use and create regular expressions, go to: http://docs.oracle.com/javase/tutorial/essential/regex/index.html This program should ask a user to enter a Phone number, the program will then check and respond with the "The phone number is valid" or "This isn't a valid phone number" A method called phoneFormatCheck has already been created for you. The method accepts a string as input, tests it to valate that it does or doesn't match returning a Boolean "true" if it does match and "false" if it doesn't match the phone number format. Based on the true or false value returned, you need to give the proper output. Example input 1 (575) 555-1234 Example output 1 The phone number is valid Example input 2 555.123.4567 Example output 2 This isn't a valid phone number
What I have already:
import java.util.*; import java.util.regex.*;
/** * Date Created: * @author Student Name * Filename: PhoneNumberTest.java */ public class PhoneNumberTest {
/** * @param args the command line arguments */ public static void main(String[] args) { //***** enter the program code here ******
} /** * This method takes a string and test to verify it is in the proper phone * number format. The proper format will be (575) 555-1234 or (575)555-1234 * * @param phoneNumber * @return true or false based on pattern matching */ public static boolean phoneFormatCheck(String phoneNumber) { Pattern p = Pattern.compile("[(][0-9]{3}[)] ?[0-9]{3}[-][0-9]{4}"); Matcher m = p.matcher(phoneNumber); return m.matches(); } // end phoneFormatCheck
} // end PhoneNumberTest class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
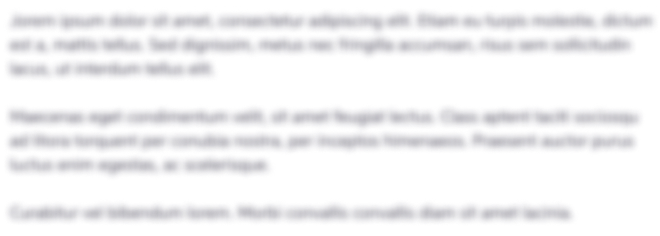
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started