Question
This has to be done in LC-3 The assignment can be broken down into the following tasks: 1. Your main code block should call a
This has to be done in LC-3
The assignment can be broken down into the following tasks: 1. Your main code block should call a MENU subroutine, which prints out a fancy looking menu with numerical options, allows the user to input a choice, and returns in R1 the choice (i.e. 1, 2, 3, 4, 5, 6, 7) that the user made (if the user inputs a non-existent option, output an error message and re-print the menu until a valid entry is obtained). Here is the menu that you will be using for the assignment. It is given to you in the template at memory address x6000 *********************** * The Busyness Server * *********************** 1. Check to see whether all machines are busy 2. Check to see whether all machines are free 3. Report the number of busy machines 4. Report the number of free machines 5. Report the status of machine n 6. Report the number of the first available machine 7. Quit 2. Write the following subroutines: A. MENU B. ALL_MACHINES_BUSY C. ALL_MACHINES_FREE D. NUM_BUSY_MACHINES E. NUM_FREE_MACHINES F. MACHINE_STATUS G. FIRST_FREE
this is the code template given that includes subroutine headers
.ORIG x3000 ; Program begins here ;------------- ;Instructions ;------------- ;------------------------------- ;INSERT CODE STARTING FROM HERE ;--------------------------------
HALT ;--------------- ;Data ;--------------- ;Add address for subroutines
;Other data
;Strings for options Goodbye .STRINGZ "Goodbye! " ALLNOTBUSY .STRINGZ "Not all machines are busy " ALLBUSY .STRINGZ "All machines are busy " FREE .STRINGZ "All machines are free " NOTFREE .STRINGZ "Not all machines are free " BUSYMACHINE1 .STRINGZ "There are " BUSYMACHINE2 .STRINGZ " busy machines " FREEMACHINE1 .STRINGZ "There are " FREEMACHINE2 .STRINGZ " free machines " STATUS1 .STRINGZ "Machine " STATUS2 .STRINGZ " is busy " STATUS3 .STRINGZ " is free " FIRSTFREE1 .STRINGZ "The first available machine is number " FIRSTFREE2 .STRINGZ "No machines are free " NEWLINE .FILL ' '
;----------------------------------------------------------------------------------------------------------------- ; Subroutine: MENU ; Inputs: None ; Postcondition: The subroutine has printed out a menu with numerical options, allowed the ; user to select an option, and returned the selected option. ; Return Value (R1): The option selected: #1, #2, #3, #4, #5, #6 or #7 ; no other return value is possible ;----------------------------------------------------------------------------------------------------------------- ;------------------------------- ;INSERT CODE For Subroutine MENU ;-------------------------------- ;HINT back up
;HINT Restore
;-------------------------------- ;Data for subroutine MENU ;-------------------------------- Error_msg_1 .STRINGZ "INVALID INPUT " Menu_string_addr .FILL x6000
;----------------------------------------------------------------------------------------------------------------- ; Subroutine: ALL_MACHINES_BUSY ; Inputs: None ; Postcondition: The subroutine has returned a value indicating whether all machines are busy ; Return value (R2): 1 if all machines are busy, 0 otherwise ;----------------------------------------------------------------------------------------------------------------- ;------------------------------- ;INSERT CODE For Subroutine ALL_MACHINES_BUSY ;-------------------------------- ;HINT back up
;HINT Restore
;-------------------------------- ;Data for subroutine ALL_MACHINES_BUSY ;-------------------------------- BUSYNESS_ADDR_ALL_MACHINES_BUSY .Fill xA400
;----------------------------------------------------------------------------------------------------------------- ; Subroutine: ALL_MACHINES_FREE ; Inputs: None ; Postcondition: The subroutine has returned a value indicating whether all machines are free ; Return value (R2): 1 if all machines are free, 0 otherwise ;----------------------------------------------------------------------------------------------------------------- ;------------------------------- ;INSERT CODE For Subroutine ALL_MACHINES_FREE ;-------------------------------- ;HINT back up
;HINT Restore
;-------------------------------- ;Data for subroutine ALL_MACHINES_FREE ;-------------------------------- BUSYNESS_ADDR_ALL_MACHINES_FREE .Fill xA400
;----------------------------------------------------------------------------------------------------------------- ; Subroutine: NUM_BUSY_MACHINES ; Inputs: None ; Postcondition: The subroutine has returned the number of busy machines. ; Return Value (R2): The number of machines that are busy ;----------------------------------------------------------------------------------------------------------------- ;------------------------------- ;INSERT CODE For Subroutine NUM_BUSY_MACHINES ;-------------------------------- ;HINT back up
;HINT Restore
;-------------------------------- ;Data for subroutine NUM_BUSY_MACHINES ;-------------------------------- BUSYNESS_ADDR_NUM_BUSY_MACHINES .Fill xA400
;----------------------------------------------------------------------------------------------------------------- ; Subroutine: NUM_FREE_MACHINES ; Inputs: None ; Postcondition: The subroutine has returned the number of free machines ; Return Value (R2): The number of machines that are free ;----------------------------------------------------------------------------------------------------------------- ;------------------------------- ;INSERT CODE For Subroutine NUM_FREE_MACHINES ;-------------------------------- ;HINT back up
;HINT Restore
;-------------------------------- ;Data for subroutine NUM_FREE_MACHINES ;-------------------------------- BUSYNESS_ADDR_NUM_FREE_MACHINES .Fill xA400
;----------------------------------------------------------------------------------------------------------------- ; Subroutine: MACHINE_STATUS ; Input (R1): Which machine to check ; Postcondition: The subroutine has returned a value indicating whether the machine indicated ; by (R1) is busy or not. ; Return Value (R2): 0 if machine (R1) is busy, 1 if it is free ;----------------------------------------------------------------------------------------------------------------- ;------------------------------- ;INSERT CODE For Subroutine MACHINE_STATUS ;-------------------------------- ;HINT back up
;HINT Restore
;-------------------------------- ;Data for subroutine MACHINE_STATUS ;-------------------------------- BUSYNESS_ADDR_MACHINE_STATUS.Fill xA400
;----------------------------------------------------------------------------------------------------------------- ; Subroutine: FIRST_FREE ; Inputs: None ; Postcondition: ; The subroutine has returned a value indicating the lowest numbered free machine ; Return Value (R2): the number of the free machine ;----------------------------------------------------------------------------------------------------------------- ;------------------------------- ;INSERT CODE For Subroutine FIRST_FREE ;-------------------------------- ;HINT back up
;HINT Restore
;-------------------------------- ;Data for subroutine FIRST_FREE ;-------------------------------- BUSYNESS_ADDR_FIRST_FREE .Fill xA400
;----------------------------------------------------------------------------------------------------------------- ; Subroutine: Get input ; Inputs: None ; Postcondition: ; The subroutine get up to a 5 digit input from the user within the range [-32768,32767] ; Return Value (R1): The value of the contructed input ; NOTE: This subroutine should be the same as the one that you did in assignment 5 ; to get input from the user, except the prompt is different. ;----------------------------------------------------------------------------------------------------------------- ;------------------------------- ;INSERT CODE For Subroutine ;--------------------------------
;-------------------------------- ;Data for subroutine Get input ;-------------------------------- prompt .STRINGZ "Enter which machine you want the status of (0 - 15), followed by ENTER: " Error_msg_2 .STRINGZ "ERROR INVALID INPUT " ;----------------------------------------------------------------------------------------------------------------- ; Subroutine: print number ; Inputs: ; Postcondition: ; The subroutine prints the number that is in ; Return Value : ; NOTE: This subroutine should print the number to the user WITHOUT ; leading 0's and DOES NOT output the '+' for positive numbers. ;----------------------------------------------------------------------------------------------------------------- ;------------------------------- ;INSERT CODE For Subroutine ;--------------------------------
;-------------------------------- ;Data for subroutine print number ;--------------------------------
.ORIG x6000 MENUSTRING .STRINGZ "********************** * The Busyness Server * ********************** 1. Check to see whether all machines are busy 2. Check to see whether all machines are free 3. Report the number of busy machines 4. Report the number of free machines 5. Report the status of machine n 6. Report the number of the first available machine 7. Quit "
.ORIG xA400 ; Remote data BUSYNESS .FILL xABCD ; <----!!!VALUE FOR BUSYNESS VECTOR!!!
;--------------- ;END of PROGRAM ;--------------- .END
Step by Step Solution
There are 3 Steps involved in it
Step: 1
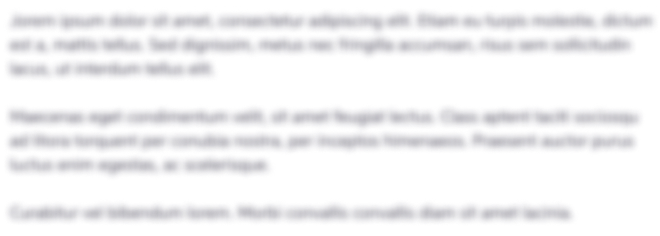
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started