This is a BASH shell script using Linux A document (txt, doc/docx, pdf) containing your BASH shell script A document (txt, doc/docx, pdf) containing the
This is a BASH shell script using Linux
A document (txt, doc/docx, pdf) containing your BASH shell script
A document (txt, doc/docx, pdf) containing the results of your BASH shell script
To
create a BASH shell script that accepts one or more arguments and calculates the wind-chill temperature given the air temperature and wind speed.
Arguments
The BASH script must test for and process the following arguments.
Argument | Optional | Description | Notes |
--airtemp= | No | The outside air temperature (in Fahrenheit by default) as a integer. | Use --cin argument for Celsius input. |
-c or --cout | Yes | Display wind-chill in Celsius rather than Fahrenheit. | Fahrenheit output is default. |
--cin | Yes | The --airtemp value is in Celsius rather than Fahrenheit. | |
--file= | Yes | Write all output to the specified file rather than the command line. | Only store answer if -q or --quiet is used. |
-h or --help | Yes | Display the help message. | See example. |
-q or --quiet | Yes | Do not display anything except the answer in the output. | See example. |
-v or --version | Yes | Display the script name and version information. | See example. |
--velocity= | No | The wind speed as a integer. |
Exit Codes
The BASH script should exit with one of the following Exit Codes (use the command echo $? on the command line to view the exit code).
Code | Description | Notes |
0 | No errors. | |
1 | Unknown argument(s). | |
2 | Invalid argument list. | Short and long arguments (-c and --cout) cannot be combined. The help and version arguments cannot be combined with any other argument. |
3 | Missing required arguments. | --airtemp, --velocity or |
4 | Value is out of range. | Outside Air Temperature or Wind Speed |
Important Notes
Refer to the Example Output section for examples to help develop the BASH script.
Program Logic
Test if any errors exist with the arguments Note: If an error exists, exit the script immediately using the appropriate Exit Code.
Unknown arguments
Invalid arguments
Missing required arguments
If the help or version argument is used
Help (-h or --help): Display the help information
Version (-v or --version): Display the version information
Display the name of the script (i.e., Wind-Chill Calculator) Note: Do not display if the -q or --quiet argument is used.
Accept the two (2) integer values (Outside Air Temperature and Wind Speed) provided by the --airtemp and --velocity argumentsIf the --cin argument is used, convert the --airtemp value from Celsius to Fahrenheit (see the Required Equations section)
The wind-chill equation expects Fahrenheit temperatures
Test if the --airtemp value is out of range Note: If value is out of range, display the appropriate error message and exit the script immediately using the appropriate Exit Code.
Valid range: -58 to 41 (inclusive)
Test if the --velocity value is out of range Note: If value is out of range, display the appropriate error message and exit the script immediately using the appropriate Exit Code.
Valid range: 2 to 50 (inclusive)
Calculate the wind-chill (see the Required Equations section)
If the -c or --cout argument is used, convert the wind-chill value to Celsius (see the Required Equations section)
Display the output (see the Example Output section) Note: If the -q or --quiet argument is used, only display the wind-chill value. Note: If the --file argument is used, write all the output for this section to the specified file
Indicate whether the value is in Fahrenheit or Celsius
Display the Wind Speed value
Display the Wind-Chill value
Indicate whether the value is in Fahrenheit or Celsius
Display the wind-chill value to three (3) decimal places
Required Equations
Convert Celsius to Fahrenheit
tf = tc * 9 / 5 + 32
Convert Fahrenheit to Celsius
tc = (tf - 32) * 5 / 9
Wind-Chill
Outside Air Temperature is represented by to
Wind Speed is represented by v Note: Use the statment `echo 'v^0.16' | bc` to calculate the exponent used in the Wind-Chill equation.
twc = 35.74 + 0.6215to - 35.75v0.16 + 0.4275tov0.16
Example Output
For each of these examples, the name of the script is windchill.
Simple Example
$ windchill --airtemp=32 --velocity=10 Wind-Chill Calculator Outside Air Temperature (F): 32 Wind Speed: 10 Wind-Chill (F): 23.727 $ echo $? # View the exit code $ 0
Celsius Input Example
$ windchill --airtemp=0 --velocity=10 --cin Wind-Chill Calculator Outside Air Temperature (C): 0 Wind Speed: 10 Wind-Chill (F): 23.727 $ echo $? # View the exit code $ 0
Celsius Output (-c or --cout) Example
$ windchill --airtemp=32 --velocity=10 -c Wind-Chill Calculator Outside Air Temperature (F): 32 Wind Speed: 10 Wind-Chill (C): -4.596 $ echo $? # View the exit code $ 0
Quiet (-q or --quiet) Example
$ windchill --airtemp=32 --velocity=10 -q 23.727 $ echo $? # View the exit code $ 0
File Output Example
$ windchill --airtemp=32 --velocity=10 --file=windchill.txt Wind-Chill Calculator $ echo $? # View the exit code $ 0 $ cat windchill.txt Outside Air Temperature (F): 32 Wind Speed: 10 Wind-Chill (F): 23.727
File Output with Quiet (-q or --quiet) Example
$ windchill --airtemp=32 --velocity=10 --file=windchill.txt -q $ echo $? # View the exit code $ 0 $ cat windchill.txt 23.727
Help (-h or --help) Example
$ windchill -h Wind-Chill Calculator Usage: windchill --airtemp=--velocity= [-c | --cout] [--cin] [--file= ] [-h | --help] [-q | --quiet] [-v | --version] Arguments --airtemp= The outside air temperature (in Fahrenheit by default) --velocity= The wind speed -c | --cout Display the wind-chill value in Celsius rather than Fahrenheit (Fahrenheit output is default) --cin The --airtemp value is in Celsius rather than Fahrenheit --file= Write all output to the specified file rather than the command line -h | --help Display this message -q | --quiet Do not display anything except the answer in the output -v | --version Display the version information $ echo $? # View the exit code $ 0
Version (-v or --version) Example
$ windchill -v Wind-Chill Calculator Version: 1.0 Author: John Doe Date: March 17, 2018 Copyright (C) 2018 by John Doe Enterprises, Inc. $ echo $? # View the exit code $ 0
Unknown Argument Example
$ windchill -d Illegal Argument(s) Usage: windchill --airtemp=--velocity= [-c | --cout] [--cin] [--file= ] [-h | --help] [-q | --quiet] [-v | --version] Try 'windchill --help' for more information. $ echo $? # View the exit code $ 1
Invalid Argument List Example
$ windchill --airtemp=32 --velocity=10 -c --count Invalid Arguments Usage: windchill --airtemp=--velocity= [-c | --cout] [--cin] [--file= ] [-h | --help] [-q | --quiet] [-v | --version] Try 'windchill --help' for more information. $ echo $? # View the exit code $ 2
Missing Required Argument Example 1
$ windchill --airtemp=32 Missing Wind Speed Argument Usage: windchill --airtemp=--velocity= [-c | --cout] [--cin] [--file= ] [-h | --help] [-q | --quiet] [-v | --version] Try 'windchill --help' for more information. $ echo $? # View the exit code $ 3
Missing Required Argument Example 2
$ windchill --velocity=10 Missing Outside Air Temperature Argument Usage: windchill --airtemp=--velocity= [-c | --cout] [--cin] [--file= ] [-h | --help] [-q | --quiet] [-v | --version] Try 'windchill --help' for more information. $ echo $? # View the exit code $ 3
Missing Required Argument Example 3
$ windchill --airtemp=32 --velocity=10 --file Missing Filename Argument Usage: windchill --airtemp=--velocity= [-c | --cout] [--cin] [--file= ] [-h | --help] [-q | --quiet] [-v | --version] Try 'windchill --help' for more information. $ echo $? # View the exit code $ 3
Outside Air Temperature (--airtemp) Out of Range Example
$ windchill --airtemp=50 --velocity=10 -c --count Outside Air Temperature (50) is Out of Range [-58 to 41] $ echo $? # View the exit code $ 4
Wind Speed (--velocity) Out of Range Example
$ windchill --airtemp=32 --velocity=0 -c --count Wind Speed (0) is Out of Range [2 to 50] $ echo $? # View the exit code $ 4
Step by Step Solution
There are 3 Steps involved in it
Step: 1
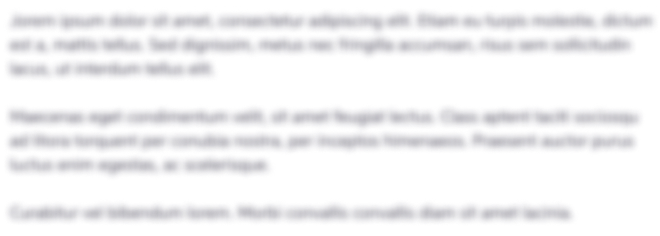
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started