Question
This is a c++ project dealing with sorting and operator overloding. Part 1 is the project description with inputs and expected outputs. Part 2 in
This is a c++ project dealing with sorting and operator overloding. Part 1 is the project description with inputs and expected outputs. Part 2 in the bottom is where the codes will go. Please help me with the correct codes, my codes are not working. Just give me the correct codes INTERMS OF PROJECT DESCRIPTION that will work will match with expected outputs. There are seven sections where my codes will go including OVER LOADED PHONE BILL, PRINT NAME, CHNAGE NAME then with FOUR DIFFERENT OPERATORS. You dont have to check or run as there are no real input files. Just arrange the codes the way i should put ON PART 2 from project description (SEE BOLD COLORED SECTIONS) in order to get same expected outputs. Just help me to fill up the codes sections on Part 2 in the bottom as best as you can. I really appreciate your kind help. Thanks.
PART 1
PROJECT 1 DESCRIPTION:
Write a C++ class definition called which will evaluate the student performance.
The prototype for the class is defined as follows:
class OVERLOADED_PHONE_BILL{
public: // public methods for this class
OVERLOADED_PHONE_BILL(char *, int); // example: g.OVERLOADED_PHONE_BILL("i_f_name",3); // first constructor reads information from in_file_name; // for first three customers; // returns nothing (void)
OVERLOADED_PHONE_BILL(void); // example: g.OVERLOADED_PHONE_BILL(); // second constructor; // initializes n to zero; // returns nothing (void) void PRINT_CUSTOMERS(int, int); // example: g.PRINT_CUSTOMERS(x, y); // method to print information; // where x is customer phone number, and y is the pin. // if x and y are -1 and -1, print all customers; // if x and y are -2 and -2, sort the customers // in alphabetical order based on last names // and then print them; sorted names are stored in // private data; // returns no values;
void CHANGE_NAME(int, int, char *, char *); // example: g.CHANGE_NAME(x, y, "FRED","LAKER"); // update the first and last names for phone number x // with pin y as FRED LAKER. // returns no values;
int operator > (OVERLOADED_PHONE_BILL &); // example: if (g1 > g2) // returns 1 if the maximum number of outgoing calls // in g1 is greater than the maximum in g2; // returns 0 otherwise;
int operator == (OVERLOADED_PHONE_BILL &); // example: if (g1 == g2) // returns 1 if the number of customers in g1 // and g2 are equal; // returns 0 otherwise;
void operator += (OVERLOADED_PHONE_BILL &); // example: g1 += g2; // append the customers in g2 to g1; // assume that all customers are unique; // returns no values;
void operator -= (OVERLOADED_PHONE_BILL &); // example: g1 -= g2; // delete the customers of g2 from g1 if they exist // in g1 (phone number, first and last names must match); // g2 does not change; // returns no values;
private: // private var to be used by this class only (not from main)
int n; // no of customers; int customer_phone_num[10]; // customers' phone numbers; int customer_pin[10]; // customers' pin numbers; int noof_incoming_calls[10]; // no of incoming calls to a customer; int noof_outgoing_calls[10]; // no of outgoing calls from a customer; char first_names[10][15]; // customers' first names (15 char max); char last_names[10][15]; // customers' last names (15 char max); char in_file_name[10]; // input file name; };
The objects of class will read the input values from a file called in.4 given with the following format:
last_name_0 first_name_0 phone_num_0 pin_0 incoming_calls_0 outgoing_calls_0 last_name_1 first_name_1 phone_num_1 pin_1 incoming_calls_1 outgoing_calls_1 last_name_2 first_name_2 phone_num_2 pin_2 incoming_calls_2 outgoing_calls_2 ... last_name_n-1 first_name_n-1 phone_num_n-1 pin_n-1 incoming_calls_n-1 outgoing_calls_n-1
where n (1<= n <= 10) is the number of customers, last_name_i is the last name, first_name_i is the first name, customer_phone_num_i (between 0 and 9999999 inclusive) is the phone number, pin_i (between 0 and 1000 inclusive) is the pin number, incoming_calls_i is the number of incoming calls and outgoing_calls_i is the number of outgoing calls for the ith customer, respectively.
\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_
An example of constructor is as follows:
OVERLOADED_PHONE_BILL g(char* i_f_name, int x); // for example: g.OVERLOADED_PHONE_BILL bill1("in.4", 3);
which instantiates an object g of class OVERLOADED_PHONE_BILL where i_f_name is the input file name and x is the number of customers. It should initialize all the variables to 0. And it should populate the private data elements. The output to out.4 file is:
++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM i_f_name FILE HAS n CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++
MAKE SURE THAT EVERY LINE TO out.4 ENDS WITH A endl.
\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_
An example of second constructor is as follows:
OVERLOADED_PHONE_BILL g(); // example: g.OVERLOADED_PHONE_BILL();
which instantiates an object g of class OVERLOADED_PHONE_BILL. The constructor sets n to 0. There is no output to out.4 file.
\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_
The definition of PRINT_CUSTOMERS method is as follows:
g.PRINT_CUSTOMERS(x, y);
where g is a object of class OVERLOADED_PHONE_BILL, x and y are the customer phone number and pin number, respectively.
If x and/or y are invalid, then this method should print the following into out.4:
++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS n CUSTOMERS. INPUT ERROR. ++++++++++++++++ END ++++++++++++++++++++++ If x and/or y are valid but are not in the private data, then this method should print the following into out.4:
++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS n CUSTOMERS. CUSTOMER NOT IN DATABASE. ++++++++++++++++ END ++++++++++++++++++++++
If x and y are valid and are in the private data, then this method should print the following into out.4:
++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS n CUSTOMERS. CUSTOMER NAME: last_name, first_name PHONE NUMBER: x PIN: y INCOMING: incoming_calls OUTGOING: outgoing_calls ++++++++++++++++ END ++++++++++++++++++++++
where last_name and first_name are the customer's last and first name having phone number x with pin y. And incoming_calls and outgoing_calls are the number of incoming calls and outgoing calls for this customer. Please make sure that all the customer information if printed in a single line into out.4 (see examples).
If x = -1 and y = -1, then all the customers are printed. This method should print the following into out.4:
++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS n CUSTOMERS. CUSTOMER NAME: last_name_0, first_name_0 PHONE NUMBER: num_0 PIN: pin_0 INCOMING: incoming_call_0 OUTGOING: outgoing_call_0 CUSTOMER NAME: last_name_1, first_name_1 PHONE NUMBER: num_1 PIN: pin_1 INCOMING: incoming_call_1 OUTGOING: outgoing_call_1 CUSTOMER NAME: last_name_2, first_name_2 PHONE NUMBER: num_2 PIN: pin_2 INCOMING: incoming_call_2 OUTGOING: outgoing_call_2 ... CUSTOMER NAME: last_name_n-1, first_name_n-1 PHONE NUMBER: num_n-1 PIN: pin_n-1 INCOMING: incoming_call_n-1 OUTGOING: outgoing_call_n-1 ++++++++++++++++ END ++++++++++++++++++++++
where last_name_i, first_name_i, num_i, pin_i, incoming_call_i and outgoing_call_i are the customer last name, first name, phone number, pin number, number of incoming calls and number of outgoing calls, respectively. Please make sure that all the customer information if printed in a single line into out.4 (see examples).
If x = -2 and y = -2, then all the customers are sorted in alphabetical order based on last names and then printed. Sorted names data are stored in private data. This method should print the following into out.4:
++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS n CUSTOMERS. CUSTOMER NAME: last_name_0, first_name_0 PHONE NUMBER: num_0 PIN: pin_0 INCOMING: incoming_call_0 OUTGOING: outgoing_call_0 CUSTOMER NAME: last_name_1, first_name_1 PHONE NUMBER: num_1 PIN: pin_1 INCOMING: incoming_call_1 OUTGOING: outgoing_call_1 CUSTOMER NAME: last_name_2, first_name_2 PHONE NUMBER: num_2 PIN: pin_2 INCOMING: incoming_call_2 OUTGOING: outgoing_call_2 ... CUSTOMER NAME: last_name_n-1, first_name_n-1 PHONE NUMBER: num_n-1 PIN: pin_n-1 INCOMING: incoming_call_n-1 OUTGOING: outgoing_call_n-1 ++++++++++++++++ END ++++++++++++++++++++++
where last_name_i, first_name_i, num_i, pin_i, incoming_call_i and outgoing_call_i are the customer last name, first name, phone number, pin number, number of incoming calls and number of outgoing calls, respectively. Please make sure that all the customer information if printed in a single line into out.4 (see examples).
MAKE SURE THAT EVERY LINE TO out.4 ENDS WITH A endl.
\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_
The definition of CHANGE_NAME method is as follows:
g.CHANGE_NAME(int x, int y, char *FIRST, char *LAST);
where g is a object of class OVERLOADED_PHONE_BILL and x and y are integers. FIRST and LAST are the names of the character strings which store the customer's first name and last name, respectively. x is the customer phone number and y is the pin number. This method replaces the old name with the new one based on its phone number and pin number.
If x and/or y are invalid, then this method should print the following into out.4:
++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM CHANGE_NAME INTERFACE: INPUT ERROR. ++++++++++++++++ END ++++++++++++++++++++++
If x and y are valid and are in the private data, then this method should change the first and last name for this customer and print the following into out.4:
++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM CHANGE_NAME INTERFACE: CUSTOMER NAME HAS BEEN CHANGED. ++++++++++++++++ END ++++++++++++++++++++++
If x and/or y are valid but are not in the private data, then this method should print the following into out.4:
++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM CHANGE_NAME INTERFACE: CUSTOMER x NOT IN DATABASE. ++++++++++++++++ END ++++++++++++++++++++++
MAKE SURE THAT EVERY LINE TO out.4 ENDS WITH A endl.
\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_
An example use of overloaded operator > method is as follows:
if(bill1 > bill2)
where bill1 and bill2 are objects of class OVERLOADED_PHONE_BILL. This overloaded operator checks if the maximum number of outgoing calls in bill1 is greater than of that in bill2.
If true, it returns 1 with the following output to out.4:
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR > INTERFACE: +++ RETURNS TRUE. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
Otherwise, the overloaded operator method returns 0 with the following output to out.4:
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR > INTERFACE: +++ RETURNS FALSE. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
MAKE SURE THAT EVERY LINE YOUR WRITE INTO out.4 ENDS WITH endl.
\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_
An example use of overloaded operator == method is as follows:
if(bill1 == bill2)
where bill1 and bill2 are objects of class OVERLOADED_PHONE_BILL. This overloaded operator checks if the number of customers in bill1 are equal to that in bill2.
If true, it returns 1 with the following output to out.4:
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR == INTERFACE: +++ RETURNS TRUE. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
Otherwise, the overloaded operator method returns 0 with the following output to out.4:
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR == INTERFACE: +++ RETURNS FALSE. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
MAKE SURE THAT EVERY LINE YOUR WRITE INTO out.4 ENDS WITH endl.
\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_
An example use of overloaded operator += method is as follows:
bill1 += bill2;
where bill1 and bill2 are objects of class OVERLOADED_PHONE_BILL. This overloaded operator appends the customers in bill 2 to bill1 (assume that all customers are unique).
If total number of customers after appending is less than 10, then it prints the following output to out.4:
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR += INTERFACE: +++ CUSTOMERS FROM in_file_name HAVE BEEN ADDED IN DATABASE. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ If total number of customers after appending is more than 10, then it prints the following output to out.4:
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR += INTERFACE: +++ INPUT ERROR. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
MAKE SURE THAT EVERY LINE YOUR WRITE INTO out.4 ENDS WITH endl.
\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_
An example use of overloaded operator -= method is as follows:
bill1 -= bill2;
where bill1 and bill2 are objects of class OVERLOADED_PHONE_BILL. This overloaded operator deletes the customers of bill2 if they exist in bill1 (phone number, first and last names must match). bill2 does not change.
If customers are deleted in bill1, then it prints the following output to out.4 file:
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR -= INTERFACE: +++ cnt CUSTOMERS DELETED. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ where cnt is an integer and denotes the number of customers deleted. If no customers are deleted in bill1, then this method prints the following output to out.4:
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR -= INTERFACE: +++ NO CUSTOMERS DELETED. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
MAKE SURE THAT EVERY LINE YOUR WRITE INTO out.4 ENDS WITH endl.
\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_\_ Your header file will be used by our staff as a header file called p4.h Each of the following examples will give you 10 points and there are zero points for compilation. 6 examples of main functions utilizing such a header are as follows: :::::::::::::: EXAMPLE 1 ::::::::::::::::::::: ---------CONTENTS OF main4_1.cc FILE:-----------
#include "p4.h" // example program: main4_1.cc int main () { OVERLOADED_PHONE_BILL bill1("in.4", 5); // instantiate an object bill1 OVERLOADED_PHONE_BILL bill2; // instantiate an object bill2
bill1.PRINT_CUSTOMERS(-1,-1); // Print the contents of in.1
return 0; } ---------CONTENTS OF in.4 FILE:--------------- Ajayi Aaba 1111111 111 10 11 Bjayi Baba 1111112 124 11 12 Djayi Daba 1111114 126 12 13 Cjayi Caba 1111113 125 13 14 Fjayi Faba 1111116 128 14 15 Ejayi Eaba 1111115 127 15 16 Jjayi Jaba 1111117 129 16 17 ---------EXPECTED CONTENTS OF out.4 FILE:----- ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 5 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS 5 CUSTOMERS. CUSTOMER NAME: Ajayi, Aaba PHONE NUMBER: 1111111 PIN: 111 INCOMING: 10 OUTGOING: 11 CUSTOMER NAME: Bjayi, Baba PHONE NUMBER: 1111112 PIN: 124 INCOMING: 11 OUTGOING: 12 CUSTOMER NAME: Djayi, Daba PHONE NUMBER: 1111114 PIN: 126 INCOMING: 12 OUTGOING: 13 CUSTOMER NAME: Cjayi, Caba PHONE NUMBER: 1111113 PIN: 125 INCOMING: 13 OUTGOING: 14 CUSTOMER NAME: Fjayi, Faba PHONE NUMBER: 1111116 PIN: 128 INCOMING: 14 OUTGOING: 15 ++++++++++++++++ END ++++++++++++++++++++++ :::::::::::::: EXAMPLE 2 ::::::::::::::::::::: ---------CONTENTS OF main4_2.cc FILE:----------- #include "p4.h"
// example program: main4_2.cc int main () { OVERLOADED_PHONE_BILL bill1("in.4", 5); // instantiate an object bill1 OVERLOADED_PHONE_BILL bill2("in.4", 2); // instantiate an object bill2 bill1.CHANGE_NAME(1111111, 111, "Fred", "Laker");
bill1.PRINT_CUSTOMERS(-2,-2); // Print the contents of in.1
return 0; } ---------CONTENTS OF in.4 FILE:--------------- Ajayi Aaba 1111111 111 10 11 Bjayi Baba 1111112 124 11 12 Djayi Daba 1111114 126 12 13 Cjayi Caba 1111113 125 13 14 Fjayi Faba 1111116 128 14 15 Ejayi Eaba 1111115 127 15 16 Jjayi Jaba 1111117 129 16 17 ---------EXPECTED CONTENTS OF out.4 FILE:----- ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 5 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 2 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM CHANGE_NAME INTERFACE: CUSTOMER NAME HAS BEEN CHANGED. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS 5 CUSTOMERS.CUSTOMER NAME: Bjayi, Baba PHONE NUMBER: 1111112 PIN: 124 INCOMING: 11 OUTGOING: 12 CUSTOMER NAME: Cjayi, Caba PHONE NUMBER: 1111113 PIN: 125 INCOMING: 13 OUTGOING: 14 CUSTOMER NAME: Djayi, Daba PHONE NUMBER: 1111114 PIN: 126 INCOMING: 12 OUTGOING: 13 CUSTOMER NAME: Fjayi, Faba PHONE NUMBER: 1111116 PIN: 128 INCOMING: 14 OUTGOING: 15 CUSTOMER NAME: Laker, Fred PHONE NUMBER: 1111111 PIN: 111 INCOMING: 10 OUTGOING: 11 ++++++++++++++++ END ++++++++++++++++++++++ :::::::::::::: EXAMPLE 3 ::::::::::::::::::::: ---------CONTENTS OF main4_3.cc FILE:----------- #include "p4.h" // example program: main4_3.cc int main () { OVERLOADED_PHONE_BILL bill1("in.4", 5); // instantiate an object bill1 OVERLOADED_PHONE_BILL bill2("in.4", 2); // instantiate an object bill2 if (bill1 > bill2) { bill1.PRINT_CUSTOMERS(1111111,111); // Print the contents of in.4 } else{} return 0; }---------CONTENTS OF in.4 FILE:--------------- Ajayi Aaba 1111111 111 10 11 Bjayi Baba 1111112 124 11 12 Djayi Daba 1111114 126 12 13 Cjayi Caba 1111113 125 13 14 Fjayi Faba 1111116 128 14 15 Ejayi Eaba 1111115 127 15 16 Jjayi Jaba 1111117 129 16 17 ---------EXPECTED CONTENTS OF out.4 FILE:----- ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 5 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 2 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR > INTERFACE: +++ RETURNS TRUE. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS 5 CUSTOMERS. CUSTOMER NAME: Ajayi, Aaba PHONE NUMBER: 1111111 PIN: 111 INCOMING: 10 OUTGOING: 11 ++++++++++++++++ END ++++++++++++++++++++++ :::::::::::::: EXAMPLE 4 ::::::::::::::::::::: ---------CONTENTS OF main4_4.cc FILE:----------- #include "p4.h"
// example program: main4_4.cc int main () { OVERLOADED_PHONE_BILL bill1("in.4", 5); // instantiate an object bill1 OVERLOADED_PHONE_BILL bill2("in.4", 2); // instantiate an object bill2 OVERLOADED_PHONE_BILL bill3("in.4", 2); // instantiate an object bill3
bill1.CHANGE_NAME(1111119, 111, "Fred", "Laker");
if (bill2 > bill1) {
} else { bill2.PRINT_CUSTOMERS(-1,-1); // Print the contents of in.4 } if (bill2 == bill3) { bill2.PRINT_CUSTOMERS(-1,-1); // Print the contents of in.4 } else{}
return 0; } ---------CONTENTS OF in.4 FILE:--------------- Ajayi Aaba 1111111 111 10 11 Bjayi Baba 1111112 124 11 12 Djayi Daba 1111114 126 12 13 Cjayi Caba 1111113 125 13 14 Fjayi Faba 1111116 128 14 15 Ejayi Eaba 1111115 127 15 16 Jjayi Jaba 1111117 129 16 17 ---------EXPECTED CONTENTS OF out.4 FILE:----- ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 5 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 2 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 2 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM CHANGE_NAME INTERFACE: CUSTOMER 1111119 NOT IN DATABASE. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR > INTERFACE: +++ RETURNS FALSE. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS 2 CUSTOMERS. CUSTOMER NAME: Ajayi, Aaba PHONE NUMBER: 1111111 PIN: 111 INCOMING: 10 OUTGOING: 11 CUSTOMER NAME: Bjayi, Baba PHONE NUMBER: 1111112 PIN: 124 INCOMING: 11 OUTGOING: 12 ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR == INTERFACE: +++ RETURNS TRUE. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS 2 CUSTOMERS. CUSTOMER NAME: Ajayi, Aaba PHONE NUMBER: 1111111 PIN: 111 INCOMING: 10 OUTGOING: 11 CUSTOMER NAME: Bjayi, Baba PHONE NUMBER: 1111112 PIN: 124 INCOMING: 11 OUTGOING: 12 ++++++++++++++++ END ++++++++++++++++++++++
:::::::::::::: EXAMPLE 5 ::::::::::::::::::::: ---------CONTENTS OF main4_5.cc FILE:----------- #include "p4.h" // example program: main4_5.cc int main () { OVERLOADED_PHONE_BILL bill1("in.4", 5); // instantiate an object bill1 OVERLOADED_PHONE_BILL bill2("in.4", 2); // instantiate an object bill2 bill1.CHANGE_NAME(1111111, 101, "Fred", "Laker");
if (bill2 == bill1) {
} else { bill2.PRINT_CUSTOMERS(-1,-1); // Print the contents of in.4 }
bill1 -= bill2; bill1.PRINT_CUSTOMERS(-1,-1); // Print the contents of in.4return 0; } ---------CONTENTS OF in.4 FILE:--------------- Ajayi Aaba 1111111 111 10 11 Bjayi Baba 1111112 124 11 12 Djayi Daba 1111114 126 12 13 Cjayi Caba 1111113 125 13 14 Fjayi Faba 1111116 128 14 15 Ejayi Eaba 1111115 127 15 16 Jjayi Jaba 1111117 129 16 17 ---------EXPECTED CONTENTS OF out.4 FILE:----- ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 5 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 2 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM CHANGE_NAME INTERFACE: CUSTOMER 1111111 NOT IN DATABASE. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR == INTERFACE: +++ RETURNS FALSE. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS 2 CUSTOMERS. CUSTOMER NAME: Ajayi, Aaba PHONE NUMBER: 1111111 PIN: 111 INCOMING: 10 OUTGOING: 11 CUSTOMER NAME: Bjayi, Baba PHONE NUMBER: 1111112 PIN: 124 INCOMING: 11 OUTGOING: 12 ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR -= INTERFACE: +++ 2 CUSTOMERS DELETED.
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ OUTPUT FROM PRINT_CUSTOMERS INTERFACE: THIS OVERLOADED_PHONE_BILL HAS 3 CUSTOMERS. CUSTOMER NAME: Djayi, Daba PHONE NUMBER: 1111114 PIN: 126 INCOMING: 12 OUTGOING: 13 CUSTOMER NAME: Cjayi, Caba PHONE NUMBER: 1111113 PIN: 125 INCOMING: 13 OUTGOING: 14 CUSTOMER NAME: Fjayi, Faba PHONE NUMBER: 1111116 PIN: 128 INCOMING: 14 OUTGOING: 15 ++++++++++++++++ END ++++++++++++++++++++++ :::::::::::::: EXAMPLE 6 ::::::::::::::::::::: ---------CONTENTS OF main4_6.cc FILE:----------- #include "p4.h" // example program: main4_6.cc int main () { OVERLOADED_PHONE_BILL bill1("in.4", 5); // instantiate an object bill1 OVERLOADED_PHONE_BILL bill2("in.4", 2); // instantiate an object bill2
bill1 -= bill2; bill1.PRINT_CUSTOMERS(-1,-1); // Print the contents of in.4
bill1 += bill2; bill1.PRINT_CUSTOMERS(-1,-1); // Print the contents of in.4
return 0; }---------CONTENTS OF in.4 FILE:--------------- Ajayi Aaba 1111111 111 10 11 Bjayi Baba 1111112 124 11 12 Djayi Daba 1111114 126 12 13 Cjayi Caba 1111113 125 13 14 Fjayi Faba 1111116 128 14 15 Ejayi Eaba 1111115 127 15 16 Jjayi Jaba 1111117 129 16 17 ---------EXPECTED CONTENTS OF out.4 FILE:----- ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 5 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++ CONSTRUCTOR SUCCESSFULLY INITIATED AN OBJECT. THIS OVERLOADED_PHONE_BILL FROM in.4 FILE HAS 2 CUSTOMERS. ++++++++++++++++ END ++++++++++++++++++++++ ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ +++ OUTPUT FROM OVERLOADED OPERATOR -= INTERFACE: +++ 2 CUSTOMERS DELETED. ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ ++++++++++++++++ START ++++++++++++++++++++++
PART 2 (CODES WILL GO THIS PART)
#include
using namespace std;
// declare the output file ofstream output_file("out.4",ios::out);
class OVERLOADED_PHONE_BILL{
public: // public interfaces for this class
OVERLOADED_PHONE_BILL(char *, int); // example: g.OVERLOADED_PHONE_BILL("i_f_name",3); // first constructor reads information from in_file_name; // for first threee customers; // returns nothing (void)
OVERLOADED_PHONE_BILL(void); // example: g.OVERLOADED_PHONE_BILL(); // second constructor; // initializes n to zero; // returns nothing (void) void PRINT_CUSTOMERS(int, int); // example: g.PRINT_CUSTOMERS(x, y); // interface to print information; // where x is customer phone number, and y is the pin. // if x and y are -1 and -1, print all customers; // if x and y are -2 and -2, sort the customers // in alphabetical order based on last names // and then print them; sorted names are stored in // private data;
// returns no values; void CHANGE_NAME(int, int, char *, char *); // example: g.CHANGE_NAME(x, y, "FRED","LAKER"); // update the first and last names for phone number x // with pin y as FRED LAKER. // returns no values;
int operator > (OVERLOADED_PHONE_BILL &); // example: if (g1 > g2) // returns 1 if the maximum number of outgoing calls // in g1 is graeter than the maximum in g2; // returns 0 otherwise;
int operator == (OVERLOADED_PHONE_BILL &); // example: if (g1 == g2) // returns 1 if the number of customers in g1 // and g2 are equal; // returns 0 otherwise;
void operator += (OVERLOADED_PHONE_BILL &); // example: g1 += g2; // append the customers in g2 to g1; // assume that all customers are unique; // returns no values;
void operator -= (OVERLOADED_PHONE_BILL &); // example: g1 -= g2; // delete the customers of g2 from g1 if they exist // in g1 (phone number, first and last names must match); // g2 does not change; // returns no values; private: // private var to be used by this class only (not from main)
int n; // no of customers; int customer_phone_num[10]; // customers' phone numbers; int customer_pin[10]; // customers' pin numbers; int noof_incoming_calls[10]; // no of incoming calls to a customer; int noof_outgoing_calls[10]; // no of outgoing calls from a customer; char first_names[10][15]; // customers' first names (15 char max); char last_names[10][15]; // customers' last names (15 char max); char in_file_name[10]; // input file name:
};
OVERLOADED_PHONE_BILL::OVERLOADED_PHONE_BILL(char* i_f_name, int x) { // code for constructor goes here
int i; n = x;
// Copy the input file name to in_file_name strcpy(in_file_name, i_f_name); for (i=0; i<10; i++) { customer_phone_num[i] = 0; customer_pin[i] = 0; noof_incoming_calls[i] = 0; noof_outgoing_calls[i] = 0;
}
// declare the input file
// read private data elements from in.4 file for n customers:
}
OVERLOADED_PHONE_BILL::OVERLOADED_PHONE_BILL(void) { // code for second constructor goes here // This constructor sets n to 0 n=0;
}
void OVERLOADED_PHONE_BILL:: PRINT_CUSTOMERS(int x, int y) {
// print private data elements for n customers:
// code for PRINT_CUSTOMERS goes here }
void OVERLOADED_PHONE_BILL:: CHANGE_NAME(int x, int y, char *FIRST, char *LAST) { // code for CHANGE_NAME goes here }
int OVERLOADED_PHONE_BILL:: operator > (OVERLOADED_PHONE_BILL & second_bill) { // code for operator > goes here }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
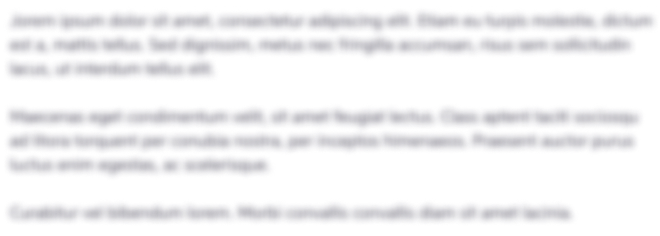
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started