Question
This is a C++ Scenario - The only change to the Employee class is that there is a new attribute: +benefit : Benefit Notice that
This is a C++ Scenario -
The only change to the Employee class is that there is a new attribute:
+benefit : Benefit
Notice that there is a "+" for this attribute, meaning that it is public. Make sure to examine the multi-arg constructor's signature!
Also, the dotted directed line between Employee and iEmployee specifies that the Employee class must implement the iEmployee abstract class, and thus provide an implementation for the calculatePay method.
Modify the Employee Class
Using the UML Diagrams from Step 1, create the Benefit class. To get an idea of how to format displayBenefits, take a look at the output in Step 5.
Add a Benefit attribute to the Employee class.
Initialize the new Benefit attribute in both Employee constructors. Again, take note of the multi-arg constructors parameter list!
Create the iEmployee interface (abstract class in C++).
Modify the Employee class to implement the new interface so that Employee will have to implement the calculatePay method.
Modify the Employee class to call displayBenefit when displaying Employee information.
Modify the Main Method
Notice that the Employee class now has a public benefit object inside it. This means that you can access the set methods of the Benefit object with the following code:
.benefit.
As an example, to set the lifeInsurance attribute inside an Employee object called emp, we could execute the following code:
emp.benefit.setLifeInsurance(lifeInsurance);
The steps required to modify the Main class are below. New steps are in bold.
Create an Employee object using the default constructor.
Prompt for and then set the first name, last name, and gender. Consider using your getInput method from Week 1 to obtain data from the user for this step as well as Step 3.
Prompt for and then set the dependents and annual salary using the overloaded setters that accept Strings.
Prompt for and set healthInsurance, lifeInsurance, and vacation.
Using your code from Week 1, display a divider that contains the string "Employee Information".
Display the Employee Information.
Display the number of employees created using getNumEmployees(). Remember to access getNumEmployees using the class name, not the Employee object.
Create a Benefit object called benefit1 using the multi-arg construction. Use any information you want for health insurance, life insurance, and vacation.
Create another Employee object and use the constructor to fill it with the following: "Mary", "Noia", 'F', 5, 24000.0, benefit1
Using your code from Week 1, display a divider that contains the string "Employee Information".
Display the employee information.
Display the number of employees created using getNumEmployees(). Remember to access getNumEmployees using the class name, not the Employee object.
My Code:
Source.cpp
Main
#include
#include
#include
#include "Employee.h"
void DisplayApplicationInformation();
void DisplayDivider(string outputTitle);
string GetInput(string inputType);
void TerminateApplication();
int Employee :: numEmployees = 0;
int main(){
DisplayApplicationInformation();
DisplayDivider("Start Program");
Employee employeeObject1;
DisplayDivider(" Employee 1 ");
string firstName = GetInput("First Name: ");
employeeObject1.setFirstName(firstName);
string lastName = GetInput("Last Name: ");
employeeObject1.setLastName(lastName);
string gender = GetInput("Gender: ");
char firstCharacterGender = gender[0];
employeeObject1.setGender(firstCharacterGender);
string dependents = GetInput("Dependents: ");
employeeObject1.setDependents(dependents);
string annualSalary = GetInput("Annual Salary: ");
employeeObject1.setAnnualSalary(annualSalary);
employeeObject1.displayEmployee();
cout
DisplayDivider("Number of Employee Object(s) Created");
cout
DisplayDivider(" Employee 2 ");
Employee employeeObject2("Mary", "Noia", 'F', 2, 150000.00);
DisplayDivider(" Employee Information ");
employeeObject2.displayEmployee();
cout
DisplayDivider("Number of Employee Object(s) Created");
cout
TerminateApplication();
}
void DisplayApplicationInformation(){
cout
cout
cout
cout
}
void DisplayDivider(string outputTitle){
cout
cout
}
string GetInput(string inputType){
string strInput;
cout
getline(cin, strInput);
return strInput;
}
void TerminateApplication(){
cout
cout
cout
system("pause");
}
Employee.cpp
Employee Class
#include
#include
#include
#include "Employee.h"
Employee :: Employee(){
firstName = "not given";
lastName = "not given";
gender = 'U'; // U for unknown
dependents = 0;
annualSalary = 20000;
numEmployees++;
}
Employee::Employee(string first, string last, char gen, int dep, double salary){
firstName = first;
lastName = last;
gender = gen;
dependents = dep;
annualSalary = salary;
numEmployees++;
}
double Employee :: calculatePay(){
return annualSalary/52;
}
void Employee :: displayEmployee(){
cout
cout
cout
cout
cout
}
string Employee :: getFirstName(){
return firstName;
}
void Employee :: setFirstName(string first){
firstName = first;
}
string Employee :: getLastName(){
return lastName;
}
void Employee :: setLastName(string last){
lastName = last;
}
char Employee :: getGender(){
return gender;
}
void Employee :: setGender(char gen){
gender = gen;
}
int Employee :: getDependents(){
return dependents;
}
void Employee :: setDependents(int dep){
dependents = dep;
}
void Employee :: setDependents(string dep){
dependents = stoi(dep);
}
double Employee :: getAnnualSalary(){
return annualSalary;
}
void Employee :: setAnnualSalary(double salary){
annualSalary = salary;
}
void Employee :: setAnnualSalary(string salary){
annualSalary = stod(salary);
}
int Employee :: getNumEmployees(){
return numEmployees;
}
Employee.h
Employee Header
using namespace std;
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
class Employee{
private:
string firstName;
string lastName;
char gender;
int dependents;
double annualSalary;
static int numEmployees;
public:
Employee();
Employee(string first, string last, char gen, int dep, double salary);
double calculatePay();
void displayEmployee();
string getFirstName();
void setFirstName(string first);
string getLastName();
void setLastName(string last);
char getGender();
void setGender(char gen);
int getDependents();
void setDependents(int dep);
void setDependents(string dep);
double getAnnualSalary();
void setAnnualSalary(double salary);
void setAnnualSalary(string salary);
static int getNumEmployees();
};
#endif
Emplovee - firstName string lastName string -gender: char - dependents: int > iEmployee +calculatePay): double _ annua!Salary : double -static numEmployees : int = 0 +benefit: Beneft +Employee() +Employee(in fname String, in Iname Benefit String, in gen: char, in dep: int, in sal double) -healthinsurance string lifeinsurance double vacation int +Benefit() +Benefit (in health string, in life: double, in vacation int) +displayBenefits(): void +getHealthinsurance(): string +setHealthlnsutance (in hins string): void +getLifelnsurance0): double +setLifelnsurance(in lifelns:double): void +getVacation(): int +setVacation(in vaca int): void 1+calculatePay) double +displayEmployee() void +static getNumEmployees: int +getFirstName): string +setFirstName(in name String): void +getLastName) String +setLastName (in name String): void +getGender): char +setGender(in gen: char): void +getDependents): int +setDependents(in dep: int) void +getAnnualSalary double +setAnnualSalary (in sal: double): void +setAnnualSalarv(in sal : String): void Emplovee - firstName string lastName string -gender: char - dependents: int > iEmployee +calculatePay): double _ annua!Salary : double -static numEmployees : int = 0 +benefit: Beneft +Employee() +Employee(in fname String, in Iname Benefit String, in gen: char, in dep: int, in sal double) -healthinsurance string lifeinsurance double vacation int +Benefit() +Benefit (in health string, in life: double, in vacation int) +displayBenefits(): void +getHealthinsurance(): string +setHealthlnsutance (in hins string): void +getLifelnsurance0): double +setLifelnsurance(in lifelns:double): void +getVacation(): int +setVacation(in vaca int): void 1+calculatePay) double +displayEmployee() void +static getNumEmployees: int +getFirstName): string +setFirstName(in name String): void +getLastName) String +setLastName (in name String): void +getGender): char +setGender(in gen: char): void +getDependents): int +setDependents(in dep: int) void +getAnnualSalary double +setAnnualSalary (in sal: double): void +setAnnualSalarv(in sal : String): voidStep by Step Solution
There are 3 Steps involved in it
Step: 1
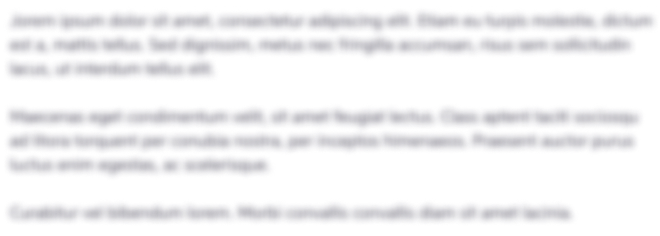
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started