Question
This is a CSC 220 or java data structures programming project. Everything in Bold letters is the Question. There were some attached files under th
This is a CSC 220 or java data structures programming project. Everything in Bold letters is the Question. There were some attached files under th name of pj3-s2017-stud. There was under PJ3 and another file which said DataFile. I copy and pasted the java files below the questions. I will also put the screenshots of the folder pj3-s2017-stud at the end of the page.
1. Introduction
We want to write a program to simulate a bank with multiple tellers. The goal of this simulation is to collect statistical information of the banks customers and tellers. A bank service area consists of several tellers and a customer waiting line (or queue). In each time unit, at most one new customer arrives at the waiting line. If the customer waiting line is too long, the customer leaves without completing transaction; otherwise, the customer gets into the waiting line. If all tellers are busy, customers in the waiting lines must wait to for a teller. If a teller is free and customers are waiting, the first customer in the waiting line advances to the tellers counter and begins transaction. When a customer is done, the customer departs and the teller becomes free. We will run the simulation through many units of time. At the end of each time unit, your program should print out a "snap-shot" of queues, customers and tellers. At the end of the program, your program should print out statistics and conclude simulation.
2. Assumptions and Requirements
2.1. Assumptions
- At most one customer arrives per time unit - All numbers are positive integer numbers (>=0), except average values should be displayed to two decimal places - No time lost in between events: a customer arriving and entering waiting line a customer arriving and leaving without banking a customer completing transaction and departing a customer leaving the waiting line, advancing to a teller and beginning transaction
2.2. The limits of simulations parameters
- Maximum number of tellers 10 - Maximum simulation length 10000 - Maximum transaction time 500 - Maximum customer queue limit 50 - Probability of a new customer 1% - 100%
2.3. Input parameters and customer (random/file) data
- The following data are read at the beginning of the simulation:
int numTellers; // number of Tellers. int simulationTime; // time to run simulation int customerQLimit; // customer queue limit int chancesOfArrival; // probability of a new Customer ( 1 - 100) int maxTransactionTime; // maximum transaction time per customer int dataSource; // data source: from file or random
- Sample input layout :
$ java PJ3.BankSimulator
*** Get Simulation Parameters ***
Enter simulation time (positive integer) : 10 Enter the number of tellers : 3 Enter chances (0% < & <= 100%) of new customer : 75 Enter maximum transaction time of customers : 5 Enter customer queue limit : 2 Enter 0/1 to get data from Random/file : 1 <- see datails below Enter filename : DataFile <- for input 1 above
- In each time unit of simulation, your program will need two positive integers numbers to compute boolean anyNewArrival & int transactionTime. A user should have two options to specify the source of those numbers (input 1 or 0):
For user input 1, numbers are read from a file. A filename should be provided at the beginning of simulation. Each line in a datafile should contain two positive numbers (> 0). A datafile should contain sufficient data for simulationTime. In each time unit, anyNewArrival & transactionTime are computed in getCustomerData() as follows :
read data1 and data2 from the file; anyNewArrival = (((data1%100)+1)<= chancesOfArrival); transactionTime = (data2%maxTransactionTime)+1;
For user input 0, numbers are generated by method nextInt() in a Random object, dataRandom, which should be constructed at the beginning of simulation. In each time unit, anyNewArrival & transactionTime are computed in getCustomerData() as follows :
anyNewArrival = ((dataRandom.nextInt(100)+1) <= chancesOfArrival); transactionTime = dataRandom.nextInt(maxTransactionTime)+1;
2.4. Input/Output information
- At the end of each time unit, you program should print out "snap-shot" of simulation. At the end of simulation, you program need to print out end of simulation report
- Sample run is provided at the end of Readme file.
2.5 Data structures and simulation algorithm
- I have defined package PJ3 with the Customer, Teller and ServiceArea classes. You need to implement their methods. I also provide an outline of a simulation program BankSimulator.java.
3. Compile and run program
- You need to download PJ3 zip file (with sample datafie) from ilearn.
- Compile programs (you are in directory containing Readme file):
javac PJ3/*.java
- Run programs (you are in directory containing Readme file):
// Run simulation java PJ3.BankSimulator
4. Due Date
- 11:55 of Wednesday 4/26/2017 - To submit your project, you need to zip all source files (*.java) and upload it to ilearn.
===================================================================================== SAMPLE RUN =====================================================================================
=> Java PJ3.Customer Customer Info:customerID=1:transactionTime=5:arrivalTime=18:finishTime=28
=> java PJ3.Teller tellerID=5:startFreeTime=0:endFreeTime=0:startBusyTime=0:endBusyTime=0:totalFreeTime=0:totalBusyTime=0:totalCustomer=0>>customer:null
tellerID=5:startFreeTime=0:endFreeTime=20:startBusyTime=20:endBusyTime=25:totalFreeTime=20:totalBusyTime=0:totalCustomer=1>>customer:customerID=1:transactionTime=5:arrivalTime=15:finishTime=25
tellerID=5:startFreeTime=25:endFreeTime=20:startBusyTime=20:endBusyTime=25:totalFreeTime=20:totalBusyTime=5:totalCustomer=1>>customer:customerID=1:transactionTime=5:arrivalTime=15:finishTime=25
Teller ID : 5 Total free time : 20 Total busy time : 5 Total # of customers : 1 Average transaction time : 5.00
=> java PJ3.ServiceArea [customerID=1:transactionTime=18:arrivalTime=10:finishTime=0, customerID=2:transactionTime=33:arrivalTime=11:finishTime=0, customerID=3:transactionTime=21:arrivalTime=12:finishTime=0, customerID=4:transactionTime=37:arrivalTime=13:finishTime=0] =============================================== Remove customer:customerID=1:transactionTime=18:arrivalTime=10:finishTime=0 Remove customer:customerID=2:transactionTime=33:arrivalTime=11:finishTime=0 Remove customer:customerID=3:transactionTime=21:arrivalTime=12:finishTime=0 Remove customer:customerID=4:transactionTime=37:arrivalTime=13:finishTime=0 =============================================== freeTellerQ:[tellerID=1:startFreeTime=0:endFreeTime=0:startBusyTime=0:endBusyTime=0:totalFreeTime=0:totalBusyTime=0:totalCustomer=0>>customer:null, tellerID=2:startFreeTime=0:endFreeTime=0:startBusyTime=0:endBusyTime=0:totalFreeTime=0:totalBusyTime=0:totalCustomer=0>>customer:null, tellerID=3:startFreeTime=0:endFreeTime=0:startBusyTime=0:endBusyTime=0:totalFreeTime=0:totalBusyTime=0:totalCustomer=0>>customer:null, tellerID=4:startFreeTime=0:endFreeTime=0:startBusyTime=0:endBusyTime=0:totalFreeTime=0:totalBusyTime=0:totalCustomer=0>>customer:null] =============================================== Remove free teller:tellerID=1:startFreeTime=0:endFreeTime=0:startBusyTime=0:endBusyTime=0:totalFreeTime=0:totalBusyTime=0:totalCustomer=0>>customer:null Remove free teller:tellerID=2:startFreeTime=0:endFreeTime=0:startBusyTime=0:endBusyTime=0:totalFreeTime=0:totalBusyTime=0:totalCustomer=0>>customer:null Remove free teller:tellerID=3:startFreeTime=0:endFreeTime=0:startBusyTime=0:endBusyTime=0:totalFreeTime=0:totalBusyTime=0:totalCustomer=0>>customer:null Remove free teller:tellerID=4:startFreeTime=0:endFreeTime=0:startBusyTime=0:endBusyTime=0:totalFreeTime=0:totalBusyTime=0:totalCustomer=0>>customer:null =============================================== freeTellerQ:[] busyTellerQ:[] =============================================== Assign customers to free tellers =============================================== Insert tellers to busyTellerQ busyTellerQ:[tellerID=1:startFreeTime=0:endFreeTime=13:startBusyTime=13:endBusyTime=31:totalFreeTime=13:totalBusyTime=0:totalCustomer=1>>customer:customerID=1:transactionTime=18:arrivalTime=10:finishTime=31, tellerID=2:startFreeTime=0:endFreeTime=13:startBusyTime=13:endBusyTime=46:totalFreeTime=13:totalBusyTime=0:totalCustomer=1>>customer:customerID=2:transactionTime=33:arrivalTime=11:finishTime=46, tellerID=3:startFreeTime=0:endFreeTime=13:startBusyTime=13:endBusyTime=34:totalFreeTime=13:totalBusyTime=0:totalCustomer=1>>customer:customerID=3:transactionTime=21:arrivalTime=12:finishTime=34, tellerID=4:startFreeTime=0:endFreeTime=13:startBusyTime=13:endBusyTime=50:totalFreeTime=13:totalBusyTime=0:totalCustomer=1>>customer:customerID=4:transactionTime=37:arrivalTime=13:finishTime=50] =============================================== Remove busy teller:tellerID=1:startFreeTime=31:endFreeTime=13:startBusyTime=13:endBusyTime=31:totalFreeTime=13:totalBusyTime=18:totalCustomer=1>>customer:customerID=1:transactionTime=18:arrivalTime=10:finishTime=31 Remove busy teller:tellerID=3:startFreeTime=34:endFreeTime=13:startBusyTime=13:endBusyTime=34:totalFreeTime=13:totalBusyTime=21:totalCustomer=1>>customer:customerID=3:transactionTime=21:arrivalTime=12:finishTime=34 Remove busy teller:tellerID=2:startFreeTime=46:endFreeTime=13:startBusyTime=13:endBusyTime=46:totalFreeTime=13:totalBusyTime=33:totalCustomer=1>>customer:customerID=2:transactionTime=33:arrivalTime=11:finishTime=46 Remove busy teller:tellerID=4:startFreeTime=50:endFreeTime=13:startBusyTime=13:endBusyTime=50:totalFreeTime=13:totalBusyTime=37:totalCustomer=1>>customer:customerID=4:transactionTime=37:arrivalTime=13:finishTime=50
=> java PJ3.BankSimulator
*** Get Simulation Parameters ***
Enter simulation time (positive integer) : 10 Enter the number of tellers : 3 Enter chances (0% < & <= 100%) of new customer : 75 Enter maximum transaction time of customers : 5 Enter customer queue limit : 2 Enter 0/1 to get data from Random/file : 1 Enter filename : DataFile
*** Start Simulation ***
Teller #1 to #3 are ready...
--------------------------------------------- Time : 0 customer #1 arrives with transaction time 5 units customer #1 wait in the customer queue customer #1 gets a teller teller #1 starts serving customer #1 for 5 units --------------------------------------------- Time : 1 customer #2 arrives with transaction time 2 units customer #2 wait in the customer queue customer #2 gets a teller teller #2 starts serving customer #2 for 2 units --------------------------------------------- Time : 2 customer #3 arrives with transaction time 5 units customer #3 wait in the customer queue customer #3 gets a teller teller #3 starts serving customer #3 for 5 units --------------------------------------------- Time : 3 No new customer! customer #2 is done teller #2 is free --------------------------------------------- Time : 4 customer #4 arrives with transaction time 3 units customer #4 wait in the customer queue customer #4 gets a teller teller #2 starts serving customer #4 for 3 units --------------------------------------------- Time : 5 No new customer! customer #1 is done teller #1 is free --------------------------------------------- Time : 6 customer #5 arrives with transaction time 3 units customer #5 wait in the customer queue customer #5 gets a teller teller #1 starts serving customer #5 for 3 units --------------------------------------------- Time : 7 customer #6 arrives with transaction time 5 units customer #6 wait in the customer queue customer #4 is done teller #2 is free customer #3 is done teller #3 is free customer #6 gets a teller teller #2 starts serving customer #6 for 5 units --------------------------------------------- Time : 8 No new customer! --------------------------------------------- Time : 9 No new customer! customer #5 is done teller #1 is free
============================================
End of simulation report
# total arrival customers : 6 # customers gone-away : 0 # customers served : 6
*** Current Tellers Info. ***
# waiting customers : 0 # busy tellers : 1 # free tellers : 2
Total waiting time : 0 Average waiting time : 0.00
Busy Tellers Info. :
Teller ID : 2 Total free time : 2 Total busy time : 8 Total # of customers : 3 Average transaction time : 2.67
Free Tellers Info. :
Teller ID : 3 Total free time : 5 Total busy time : 5 Total # of customers : 1 Average transaction time : 5.00
Teller ID : 1 Total free time : 2 Total busy time : 8 Total # of customers : 2 Average transaction time : 4.00
BankSimulator.java (FIRST SET OF FILE IN THE FOLDER)
package PJ3;
import java.util.*; import java.io.*;
// You may add new functions or data in this class // You may modify any functions or data members here // You must use Customer, Teller and ServiceArea classes // to implement Bank simulator
class BankSimulator {
// input parameters private int numTellers, customerQLimit; private int simulationTime, dataSource; private int chancesOfArrival, maxTransactionTime;
// statistical data private int numGoaway, numServed, totalWaitingTime;
// internal data private int customerIDCounter; // customer ID counter private ServiceArea servicearea; // service area object private Scanner dataFile; // get customer data from file private Random dataRandom; // get customer data using random function
// most recent customer arrival info, see getCustomerData() private boolean anyNewArrival; private int transactionTime;
// initialize data fields private BankSimulator() { // add statements }
private void setupParameters() { // read input parameters // setup dataFile or dataRandom // add statements }
// Refer to step 1 in doSimulation() private void getCustomerData() { // get next customer data : from file or random number generator // set anyNewArrival and transactionTime // see Readme file for more info // add statements }
private void doSimulation() { // add statements
// Initialize ServiceArea
// Time driver simulation loop for (int currentTime = 0; currentTime < simulationTime; currentTime++) {
// Step 1: any new customer enters the bank? getCustomerData();
if (anyNewArrival) {
// Step 1.1: setup customer data // Step 1.2: check customer waiting queue too long? // if it is too long, update numGoaway // else enter customer queue } else { System.out.println("\tNo new customer!"); }
// Step 2: free busy tellers that are done at currentTime, add to free cashierQ // Step 3: get free tellers to serve waiting customers at currentTime } // end simulation loop
// clean-up - close scanner }
private void printStatistics() { // add statements into this method! // print out simulation results // see the given example in project statement // you need to display all free and busy gas pumps
// need to free up all customers in queue to get extra waiting time. // need to free up all tellers in free/busy queues to get extra free & busy time. }
// *** main method to run simulation ****
public static void main(String[] args) { BankSimulator runBankSimulator=new BankSimulator(); runBankSimulator.setupParameters(); runBankSimulator.doSimulation(); runBankSimulator.printStatistics(); }
}
Customer.java
// DO NOT ADD NEW METHODS OR DATA FIELDS! // DO NOT MODIFY METHODS OR DATA FIELDS!
package PJ3;
class Customer { private int customerID; private int transactionTime; private int arrivalTime; private int finishTime;
// default constructor Customer() { }
// constructor to set customerID, transactionTime, arrivalTime // and compute finishTime Customer(int customerid, int transactiontime, int arrivaltime) { customerID = customerid; transactionTime = transactiontime; arrivalTime = arrivaltime; }
int getTransactionTime() { return transactionTime; }
int getArrivalTime() { return arrivalTime; }
int getFinishTime() { return finishTime; }
int getCustomerID() { return customerID; }
void setFinishTime(int finishtime) { finishTime=finishtime; }
public String toString() { return "customerID="+customerID+":transactionTime="+ transactionTime+":arrivalTime="+arrivalTime+ ":finishTime="+finishTime; }
public static void main(String[] args) { // quick check! Customer mycustomer = new Customer(1,5,18); mycustomer.setFinishTime(28); System.out.println("Customer Info:"+mycustomer);
} }
ServiceArea.java
// DO NOT ADD NEW METHODS OR NEW DATA FIELDS! // DO NOT MODIFY METHODS OR NEW DATA FIELDS!
package PJ3;
import java.util.*;
//-------------------------------------------------------------------------- // // Define simulation queues in a service area. Queues hold references to Customer // and Teller objects // // Customer (FIFO) queue is used to hold waiting customers. If the queue is too long // (i.e. > customerQLimnit), customer goes away without entering customer queue // // There are several tellers in a service area. Use PriorityQueue to // hold BUSY tellers and FIFO queue to hold FREE tellers, // i.e. a teller that is FREE for the longest time should start be used first. // // To handle teller in PriorityQueue, we need to define comparator // for comparing 2 teller objects. Here is a constructor from Java API: // // PriorityQueue(int initialCapacity, Comparator super E> comparator) // // For priority queue, the default compare function is "natural ordering" // i.e. for numbers, minimum value is returned first // // User can define own comparator class for PriorityQueue. // For teller objects, we like to have smallest end busy interval time first. // i.e. use Teller's getEndBusyIntervalTime() // // The following class define compare() for two tellers :
class CompareTellers implements Comparator
class ServiceArea { // Private data fields: // define one priority queue private PriorityQueue
// define two FIFO queues private Queue
// define customer queue limit private int customerQLimit;
// Constructor public ServiceArea() { // add statements }
// Constructor public ServiceArea(int numTellers, int customerQlimit) { // add additional statements
// use ArrayDeque to construct FIFO queue objects
// construct PriorityQueue object // overide compare() in Comparator to compare Teller objects busyTellerQ= new PriorityQueue
// initialize customerQlimit
// Construct Teller objects and insert into FreeTellerQ // assign teller ID from 1, 2,..., numTellers }
// ------------------------------------------------- // freeTellerQ methods: remove, insert, empty, size // -------------------------------------------------
public Teller removeFreeTellerQ() { // remove and return a free teller // Add statetments return null; }
public void insertFreeTellerQ(Teller teller) { // insert a free teller // Add statetments }
public boolean emptyFreeTellerQ() { // is freeTellerQ empty? // Add statetments return false; }
public int numFreeTellers() { // get number of free tellers // Add statetments return 0; }
// ------------------------------------------------------- // busyTellerQ methods: remove, insert, empty, size, peek // -------------------------------------------------------
public Teller removeBusyTellerQ() { // remove and return a busy teller // Add statetments return null; }
public void insertBusyTellerQ(Teller teller) { // insert a busy teller // Add statetments }
public boolean emptyBusyTellerQ() { // is busyTellerQ empty? return busyTellerQ.isEmpty(); }
public int numBusyTellers() { // get number of busy tellers // Add statetments return 0; }
public Teller getFrontBusyTellerQ() { // get front of busy tellers // "retrieve" but not "remove" // Add statetments return null; }
// ------------------------------------------------------- // customerQ methods: remove, insert, empty, size // and check isCustomerQTooLong() // -------------------------------------------------------
public Customer removeCustomerQ() { // remove and return a customer // Add statetments return null; }
public void insertCustomerQ(Customer customer) { // insert a customer // Add statetments }
public boolean emptyCustomerQ() { // is customerQ empty? // Add statetments return false; }
public int numWaitingCustomers() { // get number of customers // Add statetments return 0; }
public boolean isCustomerQTooLong() { // is customerQ too long? // Add statetments return false; }
public void printStatistics() { System.out.println("\t# waiting customers : "+numWaitingCustomers()); System.out.println("\t# busy tellers : "+numBusyTellers()); System.out.println("\t# free tellers : "+numFreeTellers()); }
public static void main(String[] args) {
// quick check
// create a ServiceArea and 4 customers ServiceArea sc = new ServiceArea(4, 5); Customer c1 = new Customer(1,18,10); Customer c2 = new Customer(2,33,11); Customer c3 = new Customer(3,21,12); Customer c4 = new Customer(4,37,13);
// insert customers into customerQ sc.insertCustomerQ(c1); sc.insertCustomerQ(c2); sc.insertCustomerQ(c3); sc.insertCustomerQ(c4); System.out.println(""+sc.customerQ); System.out.println("==============================================="); System.out.println("Remove customer:"+sc.removeCustomerQ()); System.out.println("Remove customer:"+sc.removeCustomerQ()); System.out.println("Remove customer:"+sc.removeCustomerQ()); System.out.println("Remove customer:"+sc.removeCustomerQ()); System.out.println("===============================================");
// remove tellers from freeTellerQ System.out.println("freeTellerQ:"+sc.freeTellerQ); System.out.println("==============================================="); Teller p1=sc.removeFreeTellerQ(); Teller p2=sc.removeFreeTellerQ(); Teller p3=sc.removeFreeTellerQ(); Teller p4=sc.removeFreeTellerQ(); System.out.println("Remove free teller:"+p1); System.out.println("Remove free teller:"+p2); System.out.println("Remove free teller:"+p3); System.out.println("Remove free teller:"+p4); System.out.println("==============================================="); System.out.println("freeTellerQ:"+sc.freeTellerQ); System.out.println("busyTellerQ:"+sc.busyTellerQ); System.out.println("===============================================");
// insert customers to tellers p1.freeToBusy (c1, 13); p2.freeToBusy (c2, 13); p3.freeToBusy (c3, 13); p4.freeToBusy (c4, 13); System.out.println("Assign customers to free tellers");
// insert tellers to busyTellerQ System.out.println("==============================================="); System.out.println("Insert tellers to busyTellerQ"); sc.insertBusyTellerQ(p1); sc.insertBusyTellerQ(p2); sc.insertBusyTellerQ(p3); sc.insertBusyTellerQ(p4); System.out.println("busyTellerQ:"+sc.busyTellerQ); System.out.println("===============================================");
// remove tellers from busyTellerQ p1=sc.removeBusyTellerQ(); p2=sc.removeBusyTellerQ(); p3=sc.removeBusyTellerQ(); p4=sc.removeBusyTellerQ();
p1.busyToFree(); p2.busyToFree(); p3.busyToFree(); p4.busyToFree();
System.out.println("Remove busy teller:"+p1); System.out.println("Remove busy teller:"+p2); System.out.println("Remove busy teller:"+p3); System.out.println("Remove busy teller:"+p4);
}
};
Teller.java
// DO NOT ADD NEW METHODS OR DATA FIELDS! // DO NOT MODIFY ANY METHODS OR DATA FIELDS!
package PJ3;
class Teller {
// teller id and current customer which is served by this cashier private int tellerID; private Customer customer;
// start time and end time of current free/busy interval private int startFreeTime; private int endFreeTime; private int startBusyTime; private int endBusyTime;
// for keeping statistical data private int totalFreeTime; private int totalBusyTime; private int totalCustomers;
// Constructor Teller() { this(-1); }
// Constructor with teller id Teller(int tellerId) { // add statements }
//-------------------------------- // accessor methods //--------------------------------
int getTellerID () { return tellerID; }
Customer getCustomer() { return customer; }
int getEndBusyTime() { return endBusyTime; }
//-------------------------------- // mutator methods //--------------------------------
void setCustomer(Customer newCustomer) { customer = newCustomer; }
void setStartFreeTime (int time) { startFreeTime = time; }
void setStartBusyTime (int time) { startBusyTime = time; } void setEndFreeTime (int time) { endFreeTime = time; }
void setEndBusyTime (int time) { endBusyTime = time; } void updateTotalFreeTime() { //add statements }
void updateTotalBusyTime() { //add statements }
void updateTotalCustomers() { //add statements }
//-------------------------------- // Teller State Transition methods //--------------------------------
// From free interval to busy interval void freeToBusy (Customer newCustomer, int currentTime) { // goal : start serving newCustomer at currentTime // // steps : set endFreeTime, update TotalFreeTime // set startBusyTime, endBusyTime, customer // update totalCustomers
//add statements }
// Transition from busy interval to free interval Customer busyToFree () { // goal : end serving customer at endBusyTime // // steps : update TotalBusyTime, set startFreeTime // return customer
//add statements return null; }
//-------------------------------- // Print statistical data //-------------------------------- void printStatistics () { // print teller statistics, see project statement System.out.println("\t\tTeller ID : "+tellerID); System.out.println("\t\tTotal free time : "+totalFreeTime); System.out.println("\t\tTotal busy time : "+totalBusyTime); System.out.println("\t\tTotal # of customers : "+totalCustomers); if (totalCustomers > 0) System.out.format("\t\tAverage transaction time : %.2f%n ",(totalBusyTime*1.0)/totalCustomers); }
public String toString() { return "tellerID="+tellerID+ ":startFreeTime="+startFreeTime+":endFreeTime="+endFreeTime+ ":startBusyTime="+startBusyTime+":endBusyTime="+endBusyTime+ ":totalFreeTime="+totalFreeTime+":totalBusyTime="+totalBusyTime+ ":totalCustomer="+totalCustomers+">>customer:"+customer; }
public static void main(String[] args) { // quick check Customer mycustomer = new Customer(1,5,15); Teller myteller = new Teller(5); myteller.setStartFreeTime(0); System.out.println(myteller); myteller.freeToBusy(mycustomer, 20); System.out.println(" "+myteller); myteller.busyToFree(); System.out.println(" "+myteller); System.out.println(" "); myteller.printStatistics();
}
};
Screenshots
Step by Step Solution
There are 3 Steps involved in it
Step: 1
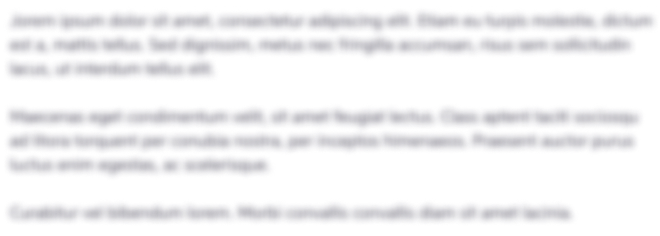
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started