Answered step by step
Verified Expert Solution
Question
1 Approved Answer
This is a java program. Do it in vs code. I need both solution for convert.java Class (PART 1) and convertTest.java class (PART 2). provide
This is a java program. Do it in vs code. I need both solution for convert.java Class (PART 1) and convertTest.java class (PART 2). provide the output too.
Do part 1 and part 2 please
1. Create a new package named "p1_converting" to contain the "Part1 Convert Java Code Files". NOTES: - A package in Java Project is simply just a folder in Windows/Mac - Using the "." Symbol in the package name means that there are more than one package (folder) Examples: - "p1_converting" one package/folder named "p1_converting" - "p1.converting" two packages/folders: - "p1" is the first package/folder - "converting" is the second package/folder which is inside "p1" 2. Create a new class (java) file named "Convert". This class will be used/called by the main class later to run your application, so do not add the "main()" method inside this class. Now all your code starting from the following point/question (3) will be written inside the "Convert" class. 3. Create two class fields/variables (without values): a) A field/variable named "massResult" of "double" data type and make it "static" b) A field/variable named "tempResult" of "double" data type and make it "static" 4. Create a method named "getKilogramValue()". This method will be used to convert a mass (aka weight) in pound (lb) unit to kilogram ( kg ) unit: a) Make it "static" and "private", with a returning value of "double" data type b) It accepts one parameter of "double" data type represents the mass value in pound c) It returns the result of the formula for converting the passing value which is in pound to kilogram 5. Create a method named "getPoundValue()". This method will be used to convert a mass in kilogram (kg) unit to pound (lb) unit: a) Make it "static" and "private", with a returning value of "double" data type b) It accepts one parameter of "double" data type represents the mass value in kilogram c) It returns the result of the formula for converting the passing value which is in kilogram to pound HINTS For Points 4 and 5: - The approximation for converting kilograms ( kg ) to pounds (lb) is 1kg=2.2lb. - To convert from kilogram to pound multiply (kg) value by 2.2 - To convert from pound to kilogram divide (Ib) value by 2.2 6. Create a method named "getFahrenheitValue ()". This method will be used to convert a temperature value in Celsius unit to Fahrenheit unit: a) Make it "static" and "private", with a returning value of "double" data type b) It accepts one parameter of "double" data type represents the temp value in Celsius c) It returns the result of the formula for converting the passing value which is in Cel to Fah 7. Create a method named "getCelsiusValue ()". This method will be used to convert a temperature value in Fahrenheit unit to Celsius unit: d) Make it "static" and "private", with a returning value of "double" data type e) It accepts one parameter of "double" data type represents the temp value in Fahrenheit f) It returns the result of the formula for converting the passing value which is in Fah to Cel HINTS For Points 6 and 7: - Celsius to Fahrenheit Formula Example: (12C9/5)+32=53.6F - The formula in programming: value in F=( value in C9/5)+32 - Fahrenheit to Celsius Formula: (12F32)5/9=11.11C - The formula in programming: value in C= (value in F32)5/9 NOTES AND HINTS: \$. By the end of answering these questions: 4,5,6, and 7 you will have these four methods: getKilogramValue() Converts Pound to Kilogram getFahrenheitValue(] Converts Celsius to Fahrenheit * Each method from all the list above has these same features: Static Member "static": it belongs to the class, not to an instance (object) Access Modifier: "private" to be used/called within the class itself only Accepts a double value (argument) and returns a double data type value The returned value is the result of the formulas for converting a unit into another one * Notice that all these methods are declared to be: "static" To be called inside another static methods, for this reason, they have to be "statis", otherwise, Java compiler will throw this error: [Cannot make a static reference to the nonstatic method methodName(double) from the type Convert] "private" We don't want to make them public to be used outside the class through its instances/objects which to emphasis the idea of "Encapsulation" in OOP * Every pair of related methods will be called by a public static method that you will create in the coming questions as shown below: "getKilogramValue()" \& "getPoundValue()" will be called inside "getMass()" method "getFahrenheit() " \& "getCelsiusValue()" will be called inside "getTemperature()" method In the next questions (8) and (7), you will create these two methods: getMass() \& getTemperature() 8. Create a method named "getTemperature (). This method will be used to convert between Celsius and Fahrenheit temperatures: g) Make it "static" and "public", with a returning value of "double" data type h) It accepts two parameters: - First parameter of "double" data type the temperature value - Second parameter of "integer" data type the user's choice either 1 or 2 a. If the passing value (argument) is 1 converting Cel to Fah b. If the passing value (argument) is 2 converting Fah to Cel i) You can use any type of conditional structure (if or switch) to check the choice variable value: a. When the choice value is 1 you will call "getFahrenheit () method and assign the result (returned value) of this method to the class variable (field) "tempResult" b. When the choice value is 2 you will call "getCelsius ()" method and assign the result (returned value) of this method to the class variable (field) "tempResult" c. Otherwise (when the choice is neither 1 nor 2): i. Print and error message (using System.err) "Invalid Choice! Only 1 or 2 is allowed" ii. Assign the value of 0 to the "tempResult" j) At the end, outside the conditional block, you will need to return the value of the variable "tempResult" This method has to have a numeric return data type 9. Create a method named "getMass()". This method will be used to convert between Pound and Kilogram mass (aka weight) unit: k) Make it "static" and "public", with a returning value of "double" data type l) It accepts two parameters: - First parameter of "double" data type the mass (aka weight) value - Second parameter of "integer" data type the user's choice either 1 or 2 a. If the passing value (argument) is 1 converting Pound to Kilogram b. If the passing value (argument) is 2 converting Kilogram to Pound m) You can use any type of conditional structure (if or switch) to check the choice variable value: a. When the choice value is 1 you will call "getKilogram ()" method and assign the result (returned value) of this method to the class variable (field) "massResult" b. When the choice value is 2y you will call "getPound ()" method and assign the result (returned value) of this method to the class variable (field) "massResult" c. Otherwise (when the choice is neither 1 nor 2): i. Print and error message (using System.err) "Invalid Choice! Only 1 or 2 is allowed" ii. Assign the value of 0 to the "massResult" n) At the end, outside the conditional block, you will need to return the value of the variable "massResult" this method has to have a numeric return data type 1. Create a new class file (Java file) named "ConvertTest". This class will be used to run your Java project so it will contain the "main()" method as an entry point to run your application. Now all your code starting from the following question (2) will be written inside the main() method of the "ConvertTest" class. 2. Ask the user to choose between either Temperature converting or Mass converting: Example: Enter ' 1 ' for weight converting, or '2' for temperature converting: Then save the user's input " 1 " or " 2 " into a int variable with any descriptive name you choose 3. Using "if" statement or "switch" statement condition to check: a) If the user input is 1 go to the if/case block to calculate the wanted mass/weight value b) If the user input is 2 go to the if/case block to calculate the wanted temperature value c) Else/Default output any simple message to show that the user's input was invalid In the next questions (4) and (5), you will write the full conditional structure code for calculating mass and the temperature based on the user's choice (input) 4. Inside the conditional block for calculating the wanted mass/weight value: a) Ask the user to input a temperature value Example: Enter a temperature value: Then save the user's input into a variable with any descriptive name you choose b) Ask the user again to choose between converting LB to KG or KG to LB Example: Enter '1' for converting LB to KG, or '2' for converting KG to LB: Then save the user's input " 1 " or "2" into a variable with any descriptive name you choose c) Using "if" statement or "switch" statement condition to check: i. If the user option is 1 you will go to the if/case block to call the static public method "getMass" and passing the required arguments for the required method's parameter Hint: - You will pass the temperature value that you receive from the user's input - You will need to pass the option value of 1 for converting LB to KG ii. Display the result in a nice format: Example: 33LB is 15KB iii. If the user option is 2 you will go to the if/case block to call the static public method "getMass" and passing the required arguments for the required method's parameter Hint: - You will pass the temperature value that you receive from the user's input - You will pass the option value of 2 for converting KG to LB iv. Display the result in a nice format: Example: 15 KB is 33 LB v. If the user option is something else (neither 1 nor 2 ) go to the else/default block to display a warning message to notify the user about entering an invalid option NOTES AND HINTS: * To make your code easier to read and maintain, it's better to avoid using too many nested if conditions. * In the previous questions (3) and (4): > You have first conditional structure block to check what type/kind of conversion: weight or temperature conversion Then you have a second conditional structure block to check from which unit to which unit you want to convert: - lb to kg or kg to lb - C to F or F to C With too many conditions like in this example, it's recommended for example to use "if" and "switch" statement, and it's up to you whether you prefer to have an "if" block first and place switch inside it or the other way * Refer to the "Code Hint" in the next page for more clarifications and better understanding 5. Inside the conditional block for calculating the wanted temperature value, repeat the same logic/idea as you have written in point/question (4) but this time you will calculate the temperature instead of the mass/weight, you will also have the option 1 for getting Fahrenheit temperature (instead of Kilogram) and the option 2 for Celsius temperature (instead of Pound)Step by Step Solution
There are 3 Steps involved in it
Step: 1
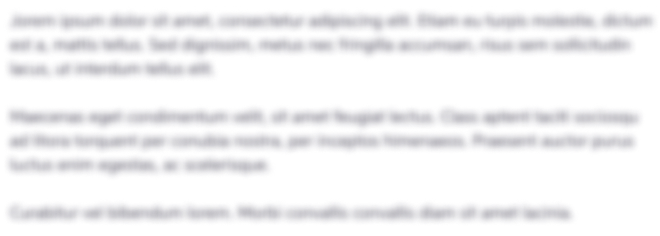
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started