Question
This is a menu-driven program that uses following options: (This is all 1 assignment) a) Add a new book to the list. The book details
This is a menu-driven program that uses following options: (This is all 1 assignment)
a) Add a new book to the list. The book details (book name, author name, publication year, number of copies) are given as function arguments. You should add the book to the list only if the book is not already present in the list and there is space in the array to add a new book.
b) Display the book information of all books in the list. See expected output.
c) Sort the book in the list by book name.
d) Find the newest book in the list. You have to necessarily use the Book pointer declared in the function and delete it before exiting the function.
#include
using namespace std;
class Book {
private:
string name, author; // data members
int pubYear, noOfCopies;
public:
Book(); // constructor
// member functions. Fucntion definition in book.cpp
void setName(string name_input);
void setAuthor(string author_input);
void setpubYear(int pubYear_input);
void setNoOfCopies(int noOfCopies_input);
void displayBookInfo();
string getName();
int getPubYear();
string getAuthor();
int getNoOfCopies();
};
#include "book.h"
#include
#include
using namespace std;
// Q1 Book (5 points)
// Book() constructor assigns the following default values to class data members
// name: abc
// author: xyz
// pubYear: 2000
// noOfCopies: 0
Book::Book()
{
}
// Q2 (10 points)
// Define the following class member functions
// These functions are to be used in functions in hw09q1.cpp when needed
// This function assigns 'name_input' to class data member 'name'
void Book::setName(string name_input)
{
}
// This function assigns author_input to class data member 'author'
void Book::setAuthor(string author_input)
{
}
// This function assigns 'pubYear_input' to class data member 'pubYear'
void Book::setpubYear(int pubYear_input)
{
}
// This function assigns 'noOfCopies_input' to class data member 'noOfCopies'
void Book::setNoOfCopies(int noOfCopies_input)
{
}
// This function displays the book name, author name, publication year and number of copies of the book.
// See expected output in question file.
void Book::displayBookInfo()
{
}
// This function returns the class data member 'name'.
string Book::getName()
{
return NULL; // REMOVE THIS LINE when implementing this function
}
// This function returns the class data member 'pubYear'.
int Book::getPubYear()
{
return 0; // REMOVE THIS LINE when implementing this function
}
// This function returns the class data member 'author'.
string Book::getAuthor()
{
return NULL; // REMOVE THIS LINE when implementing this function
}
// This function returns the class data member 'noOfCopies'.
int Book::getNoOfCopies()
{
return 0; // REMOVE THIS LINE when implementing this function
}
#include
using namespace std;
class Book {
private:
string name, author; // data members
int pubYear, noOfCopies;
public:
Book(); // constructor
// member functions. Fucntion definition in book.cpp
void setName(string name_input);
void setAuthor(string author_input);
void setpubYear(int pubYear_input);
void setNoOfCopies(int noOfCopies_input);
void displayBookInfo();
string getName();
int getPubYear();
string getAuthor();
int getNoOfCopies();
};
// You are given a partially completed program which consist of a class 'Book' defined in book.h
// The definitions of class member functions are to be filled in book.cpp
// hw09q1.c (this file) creates an array of objects 'b[]' and uses a menu driven program to add a book, display book info,
// sort the book list and to find the newest book(by publication year).
// You should start completing the program beginning from Q1. Question numbers are given around line 31.
// To begin, you should trace through the given code and understand how it works.
// Please read the instructions above each required function and follow the directions carefully.
// If you modify any of the given code, the return types, or the parameters, you risk getting compile error.
// You are not allowed to modify main ().
// ***** WRITE COMMENTS FOR IMPORANT STEPS OF YOUR CODE. *****
// ***** GIVE MEANINGFUL NAMES TO VARIABLES. *****
#include "book.h"
#include
#include
#define MAX_BOOKS 5
using namespace std;
// forward declaration of functions (already implmented)
void executeAction(char);
// functions that need implementation:
// in book.cpp :
// Q1 Book constructor // 5 points
// Q2 Book member functions // 15 points
// in this file (hw09q1.cpp) : Q3 through Q6
int addBook(string name_input, string author_input, int pubYear_input, int noOfCopies_input); // 10 points
void displayList(); // 5 points
void sortList(); // 10 points
void newestBook(); // 5 points
Book b[MAX_BOOKS]; // array of objects
int counter = 0; // number of books in the list
int main()
{
char choice = 'i'; // initialized to a dummy value
do
{
cout<<" CSE240 HW9 ";
cout << "Please enter your selection: ";
cout << "\t a: add a new book to the list ";
cout << "\t d: display entire list of books ";
cout << "\t s: sort the list by book name ";
cout << "\t n: find and display newest book ";
cout << "\t q: quit ";
cin >> choice;
cin.ignore(); // ignores the trailing
executeAction(choice);
} while (choice != 'q');
return 0;
}
// Ask for details from user for the given selection and perform that action
// Read the function case by case
void executeAction(char c)
{
string name_input, author_input;
int pubYear_input, noOfCopies_input, result = 0;
switch (c)
{
case 'a': // add book
// input book details from user
cout << "Please enter book name: ";
getline(cin, name_input);
cout << "Please enter author name: ";
getline(cin, author_input);
cout << "Please enter publication year: ";
cin >> pubYear_input;
cin.ignore();
cout << "Please enter no. of copies of book: ";
cin >> noOfCopies_input;
cin.ignore();
// add the book to the list
result = addBook(name_input, author_input, pubYear_input, noOfCopies_input);
if (result == 0)
cout<<" That book is already in the list or list is full! ";
else
cout << " Book successfully added to the list! ";
break;
case 'd': // display the list
displayList();
break;
case 's': // sort the list
sortList();
break;
case 'n': // find and display newest book
newestBook();
break;
case 'q': // quit
break;
default: cout << c <<" is invalid input! ";
}
}
// Q3 addBook (10 points)
// This function adds a new book with the details given in function arguments.
// Add the book in 'b' (array of objects) only if there is remaining capacity in the array and if the book does not already exist in the list
// This function returns 1 if the book is added successfully, else it returns 0 for the cases mentioned above.
int addBook(string name_input, string author_input, int pubYear_input, int noOfCopies_input)
{
return 1; // edit this line if needed
}
// Q4 displayList (5 points)
// This function displays the list of books.
// Parse the array and display the details of all books in the array. See expected output given in question file.
void displayList()
{
}
// Q5 sortList (10 points)
// This function sorts the books in the list alphabetically by book name.
// You may use the 'temp' object for sorting logic, if needed.
void sortList()
{
Book temp;
// enter your code here
cout << endl<< "Books sorted! Use d option to see the sorted result."<< endl;
}
// Q6 newestBook (5 points)
// This function uses 'newestBook' pointer to store the book details of the newest book in the list.
// Parse the array to find the newest book (by publication year) and copy its content to 'newestBook' object.
// Then display the book details of 'newestBook' object and delete the 'newestBook' object.
// NOTE: You necessarily have to use the 'newestBook' object to store the newest book details in it and delete it after displaying.
// You should not just print the newest book details from 'b[]' object.
void newestBook()
{
Book* newestBook = new Book;
// enter your code here
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
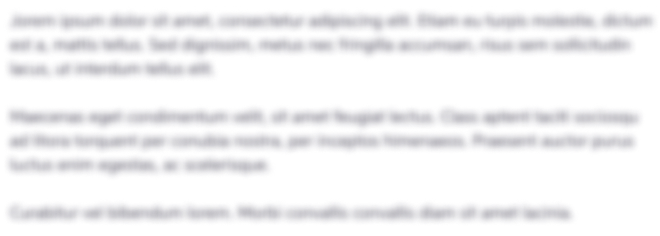
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started