Question
(This is a question that piggybacks off other questions which will be shown below) The question in mind: (PLEASE ANSWER THIS QUESTION) Write an even
(This is a question that piggybacks off other questions which will be shown below) The question in mind:
(PLEASE ANSWER THIS QUESTION) Write an even better calculator program calc3.cpp that can understand squared numbers. We are going to use a simplified notation X^ to mean X2. For example, 10^ + 7 - 51^ should mean 102 + 7 512. Example: When reading input file formulas.txt 5^; 1000 + 6^ - 5^ + 1; the program should report: $ ./calc3 < formulas.txt 25 1012 A hint: To take into account ^, dont add or subtract new numbers right away after reading them. Instead, remember the number, read the next operator and if it is a ^, square the remembered number, then add or subtract it. |
The question it piggybacks off of:
(DON'T ANSWER THIS QUESTION, IT IS JUST SHOWN FOR REFERENCE) Write a better version of the calculator, calc2.cpp, that can evaluate multiple arithmetic expressions. Lets use the semicolon symbol that must be used at the end of each expression in the input. Assuming that the input file formulas.txt looks as follows: 15 ; 10 + 3 + 0 + 25 ; 5 + 6 - 7 - 8 + 9 + 10 - 11 ; When we run the program with that input, the output should evaluate all of the expressions and print them each on its own line: $ ./calc2 < formulas.txt 15 38 4 |
The code I wrote for the piggyback question is as follows:
(CODE FOR QUESTION THAT SHOULDN'T BE ANSWERED, PLEASE TRY TO USE THIS CODE AND ADD ON TO IT TO ANSWER THE QUESTION. THANK YOU) #include using namespace std; int main() { char c; //Declaring ints/chars int sums = 0; char previousOp = '+'; int previousNum = 0; int finalNum = 0; while (cin >> c) { //Using while loop with cin input if (c == '+' || c == '-') { if (previousOp == '+') { sums += finalNum; previousNum = -1; finalNum = 0; } else if (previousOp == '-') { //Satisfying an elif loop sums -= finalNum; previousNum = -1; finalNum = 0; } if(c == '+') { previousOp = '+'; } else if(c == '-'){ //Satisfying second part of if loop with elif. previousOp = '-'; } } else if (c == ';') { // perform the arithmetic operation for the last term if (previousOp == '+') { sums += finalNum; } else if (previousOp == '-') { sums -= finalNum; } // print the result cout << sums << endl; // reset the variables sums = 0; previousOp = '+'; previousNum = 0; finalNum = 0; } else { //Else loop to satisfy other conditions. int num = c - '0'; if (previousNum == -1) { finalNum = num; previousNum = num; } else { //Else loop to satisfy other conditions. finalNum = finalNum*10 + num; previousNum = num; } } } return 0; } |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
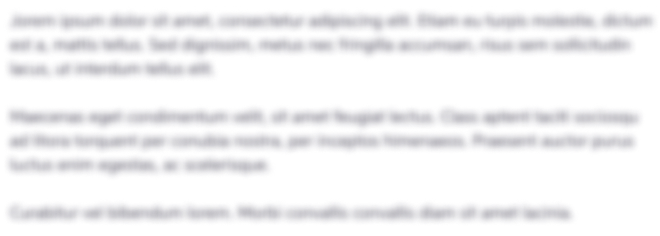
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started