Question
This is a right/left justification question on vector string for c++ Problem statement: Implement the function justify in the file justify.cpp to perform left and
Problem statement:
Implement the function justify in the file justify.cpp to perform left and right justification on a text input of type string. The justified text is a vector of strings. The assumptions for the input text are as follows:
1. The input text consists only of alphabets (lower and upper case), blank spaces ( ), and two punctuation marks (periods (.), and commas (,)).
2. Every word in a sentence in the input text is separated by a single blank space.
3. The punctuation marks immediately follow the last character of the preceding word, and a single space is present between a punctuation mark and the following word.
4. There are no new line characters in the input string.
We will perform a very primitive form of justification in this assignment using the following rules:
1. Every line in the justified text must have at least width 2 characters, with the exception of the last line, which may have fewer characters.
2. Every line in the justified text must have at most width characters, with the exception of lines that terminate with a punctuation mark (period or comma). In such cases, the line may have width+1 characters to accommodate the trailing punctuation mark.
3. Every line in the justified text must start with an alphabet (word). The first character in a line cannot be a punctuation mark or space.
4. If a word needs to be split across lines in order to follow the above rules, a hyphen (-) character must be inserted after the first part of the word. The hyphen character counts toward the total number of characters in a line.
This is given:
/*******************Programming Assignment 4***************/
/********************Text Justification**********************/
/*****************YOU MUST NOT SUBMIT THIS FILE**************/
/*********Print a given text with both left and right justification**************************/
#include \"justify.h\"
int main() {
unsigned int result;
unsigned int width;
string input;
vector output;
while (1) {
// first clear the output string
output.clear();
cout
cout
cin >> width;
if (width == 0)
break;
cout
// read in the input string
cin.ignore();
getline(cin, input);
// justify the input for given column width
result = justify(width, input, output);
switch(result) {
case ERROR_NONE:
cout
for (unsigned int i = 0; i
cout
break;
case ERROR_NO_CHARS:
cout
break;
case ERROR_ILLEGAL_WIDTH:
cout
break;
default:
cout
break;
}
} // enter interactive loop
}
This is also given:
/*******************Programming Assignment 4***************/
/********************Text Justification**********************/
/*****************YOU MUST NOT EDIT THIS FILE**************/
/*****************YOU MUST NOT SUBMIT THIS FILE**************/
/*********Definition of types, error codes and function prototype**************************/
#include
#include
#include
#include \"ctype.h\"
using namespace std;
#define MIN_COL_WIDTH 10
#define MAX_COL_WIDTH 60
// error codes
#define ERROR_NONE 0
#define ERROR_NO_CHARS 1
#define ERROR_ILLEGAL_WIDTH 2
// function prototype
unsigned int justify(unsigned int width, string in, vector &out);
What I have to do is write the function for the text justification taking into account the constraints at the beginning:
/********************Text Justification**********************/
/*****************YOU MUST EDIT and SUBMIT THIS FILE*********/
#include \"justify.h\"
unsigned int justify(unsigned int width, string in, vector & out) {
} // end function justify
Step by Step Solution
There are 3 Steps involved in it
Step: 1
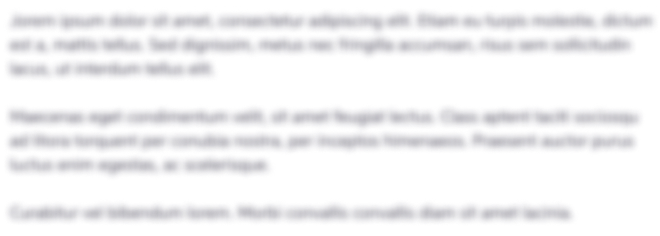
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started