Answered step by step
Verified Expert Solution
Question
1 Approved Answer
/** * This is a SIMPLE test harness for the Security class. * * Keyboard input is accepted from the user and then used to
/** * This is a SIMPLE test harness for the Security class. * * Keyboard input is accepted from the user and then used to instantiate a Security object. * Once an object has been instantiated, it will be stored in an array. * An enhanced for loop will then be used to output information about all objects in the array. */ import java.util.Scanner; // to accept keboard input public class Test_SecurityHierarchy { private static int limit = 8; private static Security[] custArray = new Security[ limit ]; // declared globally for simplicity public static void main(String[] args) { loadArray( ); outputSecuritys( ); } // end main private static void loadArray( ) { /************************************************************************************************************ * The first part of this method is simply 'set up' for the real work to be done later. * We will: * > Instantiate the Scanner here to accept keyboard input * > Create a 'holding' space for the Security object(s) to be isntantiated * > Declare variables to hold the user responses * > Declare a variable to use in controlling the input loop ************************************************************************************************************/ Scanner input = new Scanner( System.in ); // instantiate Scanner for use; tie to keyboard Security newSecurity; // "holding reference" for the Security object // Both Stock AND MutualFund objects 'fit' here /* * Create prompts for use in input loop */ // Aks what type of object String askObjType = "Do you wish to enter a Stock (1) or a MutualFund (2) ?: "; // Security-level prompts (for SUPERclass instance values) String askCustNumber = "Enter a Customer Number as 7 digits: "; String askPurchDt = "Enter a purchase date in yyyyddd (Julian) format: "; String askPurchPrc = "Enter the purchase price per share: "; String askShares = "Enter the number of shares purchased: "; String askSymbol = "Enter the symbol for the Security: "; // MutualFund prompts (for SUBclass instance values) String askType = "What type of Mutual Fund is this?: "; String askAdmin = "What is the administrative cost cap? Enter as cents per dollar: "; String askRptPeriod = "What is the reporting Period? Enter A (annual), Q (quarterly), or M (monthly): "; String askMgmt = "Is this fund actively (true) or passively (false) managed?: "; // Stock prompts (for SUBclass instance values) String askExchg = "On which exchange is this Stock traded?: "; String askDividends = "Does this Stock pay dividends? (true or false): "; String askDivDate = "In which quarter are dividends paid? Enter 0 if no dividends: "; String askDivAmount = "What is the expectged dividend per share? Enter 0 if no dividends: "; /* * Declare variables to hold the user responses */ // to hold SUPERclass instance values String custNumber; int purchDt; double purchPrc; double shares; String symbol; //================== // to hold MutualFund SUBclass instance values String type; double admin; char rptPeriod; boolean mgmt; //================== // to hold Stock SUBclass instance values String exchange; boolean dividends; int divDate; double divAmount; // Declare a variable to test type of object int objType = 0; // Declare a loop control variable int countObjects = 0; /************************************************************************************************************ * The data input loop will continue until the array has been filled. * * Each prompt is offered in turn; the response from the user is stored in the appropriate variable ************************************************************************************************************/ while( countObjects
Deliverables Submit your Java source files as a single (and single-level!!) zipped folder. Include the following source modules (only these, and only source!): Security.java MutualFund.java Stock.java CostBasis.java DO NOT submit class code (compiled/built code), edit backups (.javar files), or anything other than those items listed above, in the format specified. Requirements CostBasis Create the CostBasis interface according to the requirements in the UML The interface will be usedin the classes of the Security hierarchy to calculate the cost basis for a given investment, according to the instructions provided in the individual class diagrams. Security Create the Security class according to the requirements in the UML Class Diagram. Note that the UML Class Diagram provided does not include required standard methods-these should be included at all times as defaults. Naming for such methods MUST follow standard naming conventions. All classes in the Security hierarchy must implement the CostBasis interface. MutualFund Create the MutualFund class according to the requirements in the UML Class Diagram. Note that the UML Class Diagram provided does not include required standard methods-these should be included at all times as defaults. Naming for such methods MUST follow standard naming conventions. The MutualFund class must provide concrete instructions for implementation of the CostBasis interface Stock Create the Stock class according to the requirements in the UML Class Diagram. Note that the UML Class Diagram provided does not include required standard methods-these should be included at all times as defaults. Naming for such methods MUST follow standard naming conventions. The Stock class must provide concrete instructions for implementation of the CostBasis interface. The following is sample output from the test harness Test_SecurityHierarchy. Your output will depend on the test cases you use. Please keep in mind that I will use multiple, and different, test harnesses when grading your submission - so test thoroughly! Data input complete. Thank you The Mutua!Fund belongs to Customer# 1111111; 25845.000 shares of AAAA were purchased on 2001001 for $1.00 per share. This is a MoneyMarket fund Admin costs are capped at 0.001200 Reporting cycle is Monthly. The fund is managed passively. The cost basis for this investment is: $25,876.01 The Mutua!Fund belongs to Customer# 2222222; 2000.000 shares of BBBB were purchased on 2002002 for $500.00 per share. This is a ETF fund. Admin costs are capped at 0.004200 Reporting cycle is Annual. The fund is managed actively The cost basis for this investment is: $1,004,200.00 The stock belongs to Customer# 3333333; 10.000 shares of DDDD were purchased on 2003003 for $28.06 per share. This Stock is traded on the NYSE exchange This stock does not pay dividends The cost basis for this investment is: $2,806.00 The stock belongs to Customer# 4444444; 500.000 shares of DDDD were purchased on 2004004 for $200.00 per share. This Stock is traded on the NASDAQ exchange. A dividend of $1.25 will be paid in the 2 quarter. The cost basis for this investment is $99, 375.00 The stock belongs to Customer# 5555555; 1.000 shares of EEEE were purchased on 2005005 for $256.31 per share. This Stock is traded on the CBT exchange This stock does not pay dividends The cost basis for this investment is: $255.31 The stock belongs to Customer# 6666666; 2.000 shares of FFFF were purchased on 2006006 for $5000.10 per share. This Stock is traded on the NYSE exchange. A dividend of $25.00 will be paid in the 3 quarter. The cost basis for this investment is: $9,950.20 Deliverables Submit your Java source files as a single (and single-level!!) zipped folder. Include the following source modules (only these, and only source!): Security.java MutualFund.java Stock.java CostBasis.java DO NOT submit class code (compiled/built code), edit backups (.javar files), or anything other than those items listed above, in the format specified. Requirements CostBasis Create the CostBasis interface according to the requirements in the UML The interface will be usedin the classes of the Security hierarchy to calculate the cost basis for a given investment, according to the instructions provided in the individual class diagrams. Security Create the Security class according to the requirements in the UML Class Diagram. Note that the UML Class Diagram provided does not include required standard methods-these should be included at all times as defaults. Naming for such methods MUST follow standard naming conventions. All classes in the Security hierarchy must implement the CostBasis interface. MutualFund Create the MutualFund class according to the requirements in the UML Class Diagram. Note that the UML Class Diagram provided does not include required standard methods-these should be included at all times as defaults. Naming for such methods MUST follow standard naming conventions. The MutualFund class must provide concrete instructions for implementation of the CostBasis interface Stock Create the Stock class according to the requirements in the UML Class Diagram. Note that the UML Class Diagram provided does not include required standard methods-these should be included at all times as defaults. Naming for such methods MUST follow standard naming conventions. The Stock class must provide concrete instructions for implementation of the CostBasis interface. The following is sample output from the test harness Test_SecurityHierarchy. Your output will depend on the test cases you use. Please keep in mind that I will use multiple, and different, test harnesses when grading your submission - so test thoroughly! Data input complete. Thank you The Mutua!Fund belongs to Customer# 1111111; 25845.000 shares of AAAA were purchased on 2001001 for $1.00 per share. This is a MoneyMarket fund Admin costs are capped at 0.001200 Reporting cycle is Monthly. The fund is managed passively. The cost basis for this investment is: $25,876.01 The Mutua!Fund belongs to Customer# 2222222; 2000.000 shares of BBBB were purchased on 2002002 for $500.00 per share. This is a ETF fund. Admin costs are capped at 0.004200 Reporting cycle is Annual. The fund is managed actively The cost basis for this investment is: $1,004,200.00 The stock belongs to Customer# 3333333; 10.000 shares of DDDD were purchased on 2003003 for $28.06 per share. This Stock is traded on the NYSE exchange This stock does not pay dividends The cost basis for this investment is: $2,806.00 The stock belongs to Customer# 4444444; 500.000 shares of DDDD were purchased on 2004004 for $200.00 per share. This Stock is traded on the NASDAQ exchange. A dividend of $1.25 will be paid in the 2 quarter. The cost basis for this investment is $99, 375.00 The stock belongs to Customer# 5555555; 1.000 shares of EEEE were purchased on 2005005 for $256.31 per share. This Stock is traded on the CBT exchange This stock does not pay dividends The cost basis for this investment is: $255.31 The stock belongs to Customer# 6666666; 2.000 shares of FFFF were purchased on 2006006 for $5000.10 per share. This Stock is traded on the NYSE exchange. A dividend of $25.00 will be paid in the 3 quarter. The cost basis for this investment is: $9,950.20
Step by Step Solution
There are 3 Steps involved in it
Step: 1
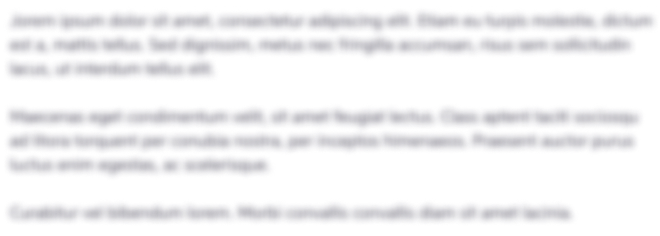
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started