Question
This is C. I current am having issues getting the second item to work and load. I am getting a random number and it is
This is C. I current am having issues getting the second item to work and load. I am getting a random number and it is not assign the value to it
Create three files to submit:
ItemToPurchase.h - Struct definition and related function declarations
ItemToPurchase.c - Related function definitions
main.c - main() function
Build the ItemToPurchase struct with the following specifications:
Data members (3 pts)
char itemName [ ]
int itemPrice
int itemQuantity
Related functionsMakeItemBlank() (2 pts)
Has a pointer to an ItemToPurchase parameter.
Sets item's name = "none", item's price = 0, item's quantity = 0
PrintItemCost()
Has an ItemToPurchase parameter.
Ex. of PrintItemCost() output:
Bottled Water 10 @ $1 = $10
(2) In main(), prompt the user for two items and create two objects of the ItemToPurchase struct. Before prompting for the second item, call fflush(stdin); to allow the user to input a new string. (2 pts) Ex:
Item 1 Enter the item name: Chocolate Chips Enter the item price: 3 Enter the item quantity: 1 Item 2 Enter the item name: Bottled Water Enter the item price: 1 Enter the item quantity: 10
(3) Add the costs of the two items together and output the total cost. (2 pts) Ex:
TOTAL COST Chocolate Chips 1 @ $3 = $3 Bottled Water 10 @ $1 = $10 Total: $13
Below is my code for each program
MAIN
#include
int main(void) { int i; int total; ItemToPurchase item1; ItemToPurchase item2; printf("Item 1 "); printf("Enter the item name: "); fflush(stdin); fgets((item1.itemName), 50, stdin); printf("Enter the item price: "); scanf("%d", &(item1.itemPrice)); printf("Enter the item quantity: "); scanf("%d", &(item1.itemQuantity)); fflush(stdin);
printf("Item 2 "); printf("Enter the item name: "); fflush(stdin); fgets((item2.itemName), 50, stdin); printf("Enter the item price: "); scanf("%d", &(item2.itemPrice)); printf("Enter the item quantity: "); scanf("%d", &(item2.itemQuantity)); total = (item1.itemPrice * item1.itemQuantity)+ (item2.itemPrice * item2.itemQuantity); printf("TOTAL COST "); printItemCost(item1); printItemCost(item2); printf("Total: $%d ", total); return 0; }
ITEMTOPURCHASE.H
#ifndef ITEM_TO_PURCHASE_H #define ITEM_TO_PURCHASE_H typedef struct ItemToPurchase_struct{ char itemName[50]; int itemPrice; int itemQuantity; } ItemToPurchase;
void MakeItemBlank(ItemToPurchase *item); void PrintItemCost(ItemToPurchase item); #endif
void MakeItemBlank(ItemToPurchase* item); void PrintItemCost(ItemToPurchase item)
#endif
ITEMTOPURCHASE.C
#include
void makeItemBlank(ItemToPurchase *item){ strcpy((item)->itemName, "None"); (item)->itemPrice=0; (item)->itemQuantity=0; }
void printItemCost(ItemToPurchase item){ printf("%s %d @ $%d = $%d ", item.itemName, item.itemQuantity,item.itemPrice, ((item.itemPrice) * (item.itemQuantity))); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
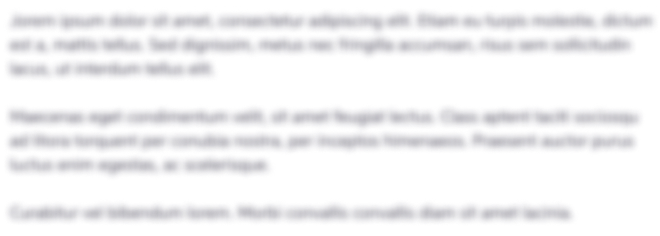
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started