Question
This is C++ please submit all files of code with their names. Turn your unorderedSet class into a template. Use the arrayListType and unorderedArrayListType templates
This is C++ please submit all files of code with their names.
Turn your unorderedSet class into a template. Use the arrayListType and unorderedArrayListType templates listed below.. Research the mathematical operations of set union and set intersection and overload the + and - operators to perform the these operations. Write a program to test your template with integer and string sets.
Turn in your unorderedSet template and your test program (make no changes to the arrayListType and unorderedArrayListType templates). Also turn in one or more screen shots showing the results of your testing.
arrayListType.h*********************
#ifndef H_arrayListType #define H_arrayListType #include#include using namespace std; template class arrayListType { public: const arrayListType & operator=(const arrayListType &); //Overloads the assignment operator bool isEmpty() const; //Function to determine whether the list is empty //Postcondition: Returns true if the list is empty; // otherwise, returns false. bool isFull() const; //Function to determine whether the list is full //Postcondition: Returns true if the list is full; // otherwise, returns false. int listSize() const; //Function to determine the number of elements in //the list. //Postcondition: Returns the value of length. int maxListSize() const; //Function to determine the maximum size of the list //Postcondition: Returns the value of maxSize. void print() const; //Function to output the elements of the list //Postcondition: Elements of the list are output on the // standard output device. bool isItemAtEqual(int location, const elemType& item) const; //Function to determine whether item is the same as //the item in the list at the position specified //by location. //Postcondition: Returns true if the list[location] // is the same as item; otherwise, // returns false. // If location is out of range, an // appropriate message is displayed. virtual void insertAt(int location, const elemType& insertItem) = 0; //Function to insert insertItem in the list at the //position specified by location. //Note that this is an abstract function. //Postcondition: Starting at location, the elements of // the list are shifted down, // list[location] = insertItem; length++; // If the list is full or location is out of // range, an appropriate message is displayed. virtual void insertEnd(const elemType& insertItem) = 0; //Function to insert insertItem an item at the end of //the list. Note that this is an abstract function. //Postcondition: list[length] = insertItem; and length++; // If the list is full, an appropriate // message is displayed. void removeAt(int location); //Function to remove the item from the list at the //position specified by location //Postcondition: The list element at list[location] is // removed and length is decremented by 1. // If location is out of range, an // appropriate message is displayed. elemType& retrieveAt(int location) const; //Function to retrieve the element from the list at the //position specified by location //Postcondition: Returns value of item at list[location] // If location is out of range, the program // is terminated. virtual void replaceAt(int location, const elemType& repItem) = 0; //Function to replace repItem the elements in the list //at the position specified by location. //Note that this is an abstract function. //Postcondition: list[location] = repItem // If location is out of range, an // appropriate message is displayed. void clearList(); //Function to remove all the elements from the list //After this operation, the size of the list is zero. //Postcondition: length = 0; virtual int seqSearch(const elemType& searchItem) const = 0; //Function to search the list for searchItem. //Note that this is an abstract function. //Postcondition: If the item is found, returns the // location in the array where the item is // found; otherwise, returns -1. virtual void remove(const elemType& removeItem) = 0; //Function to remove removeItem from the list. //Note that this is an abstract function. //Postcondition: If removeItem is found in the list, // it is removed from the list and length // is decremented by one. arrayListType(int size = 100); //Constructor //Creates an array of the size specified by the //parameter size. The default array size is 100. //Postcondition: The list points to the array, length = 0, // and maxSize = size; arrayListType (const arrayListType & otherList); //Copy constructor virtual ~arrayListType(); //Destructor //Deallocate the memory occupied by the array. protected: elemType *list; //array to hold the list elements int length; //variable to store the length of the list int maxSize; //variable to store the maximum //size of the list }; template bool arrayListType ::isEmpty() const { return (length == 0); } // //end isEmpty template bool arrayListType ::isFull() const { return (length == maxSize); } //end isFull template int arrayListType ::listSize() const { return length; } //end listSize template int arrayListType ::maxListSize() const { return maxSize; } //end maxListSize template void arrayListType ::print() const { cout << "Length = " << length << endl; for (int i = 0; i < length; i++) cout << list[i] << " "; cout << endl; } //end print template bool arrayListType ::isItemAtEqual(int location, const elemType& item) const { if (location < 0 || location >= length) { cout << "The location of the item to be removed " << "is out of range." << endl; return false; } else return (list[location] == item); } //end isItemAtEqual template void arrayListType ::removeAt(int location) { if (location < 0 || location >= length) cout << "The location of the item to be removed " << "is out of range." << endl; else { for (int i = location; i < length - 1; i++) list[i] = list[i + 1]; length--; } } //end removeAt template elemType& arrayListType ::retrieveAt(int location) const { assert(location >= 0 && location < length); return list[location]; } //end retrieveAt template void arrayListType ::clearList() { length = 0; } //end clearList template arrayListType ::arrayListType(int size) { if (size <= 0) { cout << "The array size must be positive. Creating " << "an array of the size 100. " << endl; maxSize = 100; } else maxSize = size; length = 0; list = new elemType[maxSize]; } //end constructor template arrayListType ::~arrayListType() { delete [] list; } //end destructor template arrayListType ::arrayListType (const arrayListType & otherList) { maxSize = otherList.maxSize; length = otherList.length; list = new elemType[maxSize]; //create the array for (int j = 0; j < length; j++) //copy otherList list [j] = otherList.list[j]; }//end copy constructor template const arrayListType & arrayListType ::operator= (const arrayListType & otherList) { if (this != &otherList) //avoid self-assignment { delete [] list; maxSize = otherList.maxSize; length = otherList.length; list = new elemType[maxSize]; for (int i = 0; i < length; i++) list[i] = otherList.list[i]; } return *this; } //end overloading operator= #endif
*********************************** unorderedArrayListType.h *************************************************
#ifndef H_unorderedArrayListType #define H_unorderedArrayListType #include "arrayListType.h" templateclass unorderedArrayListType: public arrayListType { public: void insertAt(int location, const elemType& insertItem); void insertEnd(const elemType& insertItem); void replaceAt(int location, const elemType& repItem); int seqSearch(const elemType& searchItem) const; void remove(const elemType& removeItem); unorderedArrayListType(int size = 100); //Constructor }; template void unorderedArrayListType ::insertAt(int location, const elemType& insertItem) { if (location < 0 || location >= this->maxSize) cout << "The position of the item to be inserted " << "is out of range." << endl; else if (this->length >= this->maxSize) //list is full cout << "Cannot insert in a full list" << endl; else { for (int i = this->length; i > location; i--) this->list[i] = this->list[i - 1]; //move the elements down this->list[location] = insertItem; //insert the item at //the specified position this->length++; //increment the length } } //end insertAt template void unorderedArrayListType ::insertEnd (const elemType& insertItem) { if (this->length >= this->maxSize) //the list is full cout << "Cannot insert in a full list." << endl; else { this->list[this->length] = insertItem; //insert the item at the end this->length++; //increment the length } } //end insertEnd template int unorderedArrayListType ::seqSearch (const elemType& searchItem) const { int loc; bool found = false; for (loc = 0; loc < this->length; loc++) if (this->list[loc] == searchItem) { found = true; break; } if (found) return loc; else return -1; } //end seqSearch template void unorderedArrayListType ::remove (const elemType& removeItem) { int loc; if (this->length == 0) cout << "Cannot delete from an empty list." << endl; else { loc = seqSearch(removeItem); if (loc != -1) this->removeAt(loc); else cout << "The item to be deleted is not in the list." << endl; } } //end remove template void unorderedArrayListType ::replaceAt(int location, const elemType& repItem) { if (location < 0 || location >= this->length) cout << "The location of the item to be " << "replaced is out of range." << endl; else this->list[location] = repItem; } //end replaceAt template unorderedArrayListType :: unorderedArrayListType(int size) : arrayListType (size) { } //end constructor #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
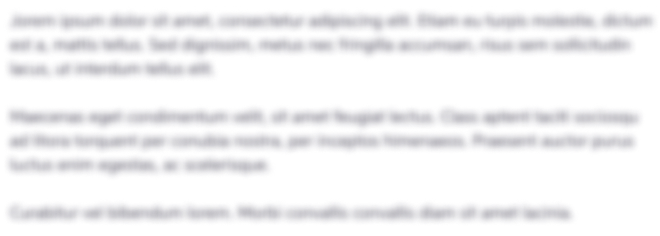
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started