Question
This is c++ program i need help with and it needs to be compiled with GNU++ Compiler it has part A, B please do both
This is c++ program i need help with and it needs to be compiled with GNU++ Compiler
it has part A, B please do both
Part A
Part B
Baseball.cpp
#include "Player.h"
#include
#include
// declaration of functions
void displayArray(Player team[], int team_size);
int sequeSearch(Player team[], int team_size,int number);
// implementation of the main program
int main()
{
// 1) connect to input file
fstream fin("baseball.txt");
// objects used to store data
const int LIST_LENGTH = 20;
// number, hits, walks, outs
int number = 0, hits, walks, outs,players,index,teamSize = 0;
// 2) output descriptive messages
cout
// 3) declare an array of LIST_LENGTH players
Player team[LIST_LENGTH];
// 4) attempt to input the data for the first Player
fin >> number >> hits >> walks >> outs;
// 5) loop on end of file
while (!fin.eof())
{
// 6) find the index of this Player's number
int index=sequeSearch(team, teamSize, number);
// 7) if player number is not in the list
if(index == -1)
{
// 7a) set the Number field for team[teamSize]
// 7b) set the Hits field for team[teamSize]
// 7c) ste the Walks field for team[teamSize]
// 7d) set the Outs field for team[teamSize]
// 7e) increase teamSize by 1
team[teamSize].setNumber(number);
team[teamSize].setHits(hits);
team[teamSize].setWalks(walks);
team[teamSize].setOuts(outs);
teamSize++;
}
else
{
// 8) else player number is in the list
// 8a) update the Hits field for team[index]
// 8b) update the Walks field for team[index]
// 8c) update the Outs field for team[index]
team[index].setHits(hits);
team[index].setWalks(walks);
team[index].setOuts(outs);
}
// 9) attempt to input the data for the next Player
fin >> number >> hits >> walks >> outs;
}
// 10) display the results
displayArray(team,teamSize);
// 11) disconnect from input file
fin.close();
} // end of main
void displayArray(Player team[], int team_size)
{
cout
// 2) loop over team size
for (int i=0; i
{
// 3) display i'th player
cout
}
}
int sequeSearch(Player team[], int team_size,int number)
{
for (int i=0; i
{
if(team[i].getNumber()==number)//check number exists then return i
return i;
}
return -1;//if number not found in list tyhen return -1
}
Baseball.txt
baseball.txt 1 2 2 2 19 0 5 1 2 0 0 6 18 4 2 0 4 1 2 3 12 2 2 2 7 0 0 3 8 1 4 1 10 2 2 2 3 2 1 3 11 6 0 0 2 0 5 1 19 0 0 6 17 4 2 0 9 3 2 1 4 2 1 3 3 1 2 3 7 0 0 3
Player.cpp
#include "Player.h" #include
Player::Player() { Number = Hits = Walks = Outs = 0; }
int Player::getNumber() const { return Number; }
int Player::getHits() const { return Hits; }
int Player::getWalks() const { return Walks; }
int Player::getOuts() const { return Outs; }
void Player::setNumber(int n) { Number = n; }
void Player::setHits(int h) { Hits = h; }
void Player::setWalks(int w) { Walks = w; }
void Player::setOuts(int o) { Outs = o; }
// support for assignments const Player& Player::operator=(const Player & p) { // bypass self assignment if (this != &p) { Number = p.Number; Hits = p.Hits; Walks = p.Walks; Outs = p.Outs; } return *this; }
// support for output to the monitor ostream& operator
Player.h
Player.h:
#ifndef PLAYER #define PLAYER #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
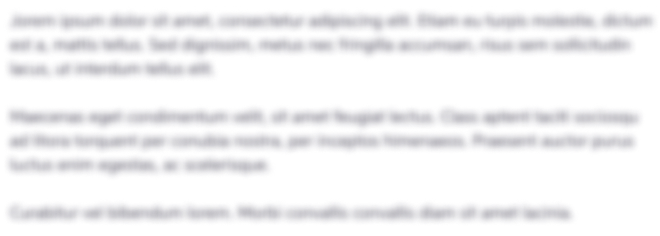
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started