Question
This is done in Eclipse. Language is Java. Use SwingWorker to implement the Account Balance scenerio with GUI. Concurrency in Swing: https://docs.oracle.com/javase/tutorial/uiswing/concurrency/index.html javax.swing.SwingWorker class Type
This is done in Eclipse. Language is Java.
Use SwingWorker to implement the Account Balance scenerio with GUI.
Concurrency in Swing:
https://docs.oracle.com/javase/tutorial/uiswing/concurrency/index.html
javax.swing.SwingWorker
Type Parameters:
T - the result type returned by this SwingWroker's doInBackground and get methods
V - the type used for carrying out intermediate results by this SwingWorker's publish and process methods
Below is the code done in class, referenced at the beginning Account balance.
import java.util.Random;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class ThreadRun {
//1. create 2 accounts
private Account account1 = new Account();
private Account account2 = new Account();
//in order to fix the multithreading issue, use lock
private Lock lock1 = new ReentrantLock();
private Lock lock2 = new ReentrantLock();
//in order to solve the deadlock issue, can create a method
//put all in one method...
private void acquireLocks(Lock firstLock, Lock secondLock) throws InterruptedException {
//use tryLock() method - a method in ReentrantLock()
//it returns immediately and true if it has lock
while(true) {
//try to acquire lock
boolean gotFirstLock = false;
boolean gotSecondLock = false;
try {
gotFirstLock = firstLock.tryLock();
gotSecondLock = secondLock.tryLock();
} finally {
if(gotFirstLock && gotSecondLock) {
return;
}
if(gotFirstLock) {
firstLock.unlock();
}
if(gotSecondLock) {
secondLock.unlock();
}
}
//if fail to acquire lock, do this
Thread.sleep(1);
}
}
public void FirstThread() throws InterruptedException{
Random random = new Random();
//let's make a scenario of transferring some money from acc1 --> acc2
for(int i=0;i<10000;i++) {
//lock1.lock();
//lock2.lock();
acquireLocks(lock1,lock2);
try {
Account.transfer(account1, account2, random.nextInt(100));
} finally {
lock1.unlock();
lock2.unlock();
}
}
}
public void SecondThread() throws InterruptedException{
Random random = new Random();
//let's make a scenario of transferring some money from acc2 --> acc1
for(int i=0;i<10000;i++) {
//lock1.lock();
//lock2.lock();
acquireLocks(lock2, lock1);
try {
Account.transfer(account2, account1, random.nextInt(100));
} finally {
lock2.unlock();
lock1.unlock(); //unlock order doesn't really matter
}
}
}
public void finished() {
System.out.println("Account1 balance is " + account1.getBalance());
System.out.println("Account2 balance is " + account2.getBalance());
System.out.println("Total balance is " + (account1.getBalance() + account2.getBalance()));
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
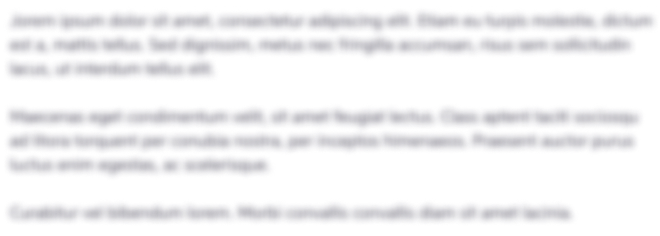
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started