Question
This is for a Java lab; I'm stuck on it, and can't even figure out what todo. If anyone is able to help, please do.
This is for a Java lab; I'm stuck on it, and can't even figure out what todo. If anyone is able to help, please do. Thank you in advanced.
Attached as well is a picture of what the tiles look like:
Intarraybag.java:
public class IntArrayBag implements Cloneable {
// instance variables
private int[ ] data; private int manyItems;
// constructor : behavior #1
public IntArrayBag( ) { final int INITIAL_CAPACITY = 10;
manyItems = 0;
data = new int[INITIAL_CAPACITY]; }
// another constructor : behavior #1
public IntArrayBag(int initialCapacity) { if (initialCapacity
throw new IllegalArgumentException ("The initialCapacity is negative: " + initialCapacity);
data = new int[initialCapacity]; manyItems = 0; } // add one item : behavior #2
public void add(int element) { if (manyItems == data.length) { // Ensure twice as much space as we need. ensureCapacity((manyItems + 1)*2); }
data[manyItems] = element; manyItems++; }
// add many items : behavior #3
public void addMany(int... elements)
// this is a variable arity method. We can specify as many parameters // as needed
{ if (manyItems + elements.length > data.length) { // Ensure twice as much space as we need. ensureCapacity((manyItems + elements.length)*2); }
System.arraycopy(elements, 0, data, manyItems, elements.length); manyItems += elements.length; }
public void addAll(IntArrayBag addend) { ensureCapacity(manyItems + addend.manyItems); System.arraycopy(addend.data, 0, data, manyItems, addend.manyItems); manyItems += addend.manyItems; } public IntArrayBag clone( ) { // Clone an IntArrayBag object. IntArrayBag answer; try { answer = (IntArrayBag) super.clone( ); } catch (CloneNotSupportedException e) { throw new RuntimeException ("This class does not implement Cloneable"); } answer.data = data.clone( ); return answer; }
// see the remainder of the IntArrayBag.java file in // the Sept 7 notes
public int countOccurrences(int target) { int count = 0; for (int index = 0; index
return count; } public void ensureCapacity(int minimumCapacity) { int biggerArray[ ]; // declaration if (data.length
System.arraycopy(data, 0, biggerArray, 0, manyItems);
data = biggerArray; } }
public int getCapacity( ) { return data.length; }
public boolean remove(int target) { int index; // must declare before the loop
for (index = 0; (index
public static IntArrayBag union(IntArrayBag b1, IntArrayBag b2) {
// a call to this method would look like // IntArrayBag.union( first IntArrayBag, second IntArrayBag )
// If either b1 or b2 is null, then a NullPointerException is // thrown. // In the case that the total number of items is beyond // Integer.MAX_VALUE, there will be an arithmetic overflow and // the bag will fail. IntArrayBag answer = new IntArrayBag(b1.getCapacity( ) + b2.getCapacity( ));
// Dr. Steiner replaced the calls to System.arraycopy // with two simple loops
for (int i = 0; i
for (int j = 0; j
answer.manyItems = b1.manyItems + b2.manyItems; return answer; } }
A: Create a class called Tile. * An object of this class represents a tile in the game called Qwirkle. You can look up the description and game play instructions online A Qwirkle tile has two attributes: 1. color-implement this as an integer between 1 and 6 (inclusive). The colors are orange, green, yello, red, blue, and purple. 2. shape- implement this as an integer between 1 and 6 (inclusive). The shapes are four-pointed star, clover, eight-pointed star, diamond, square, and circle A Qwirkle tile has typical object-oriented behaviors (only these) 1. create a tile with a given color and given shape 2. access the color of the tile 3. access the shape of the tile 4. change the color of the tile S. change the shape of the tile 6. provide a String representation of the tile 7. compare one tile for equality with another 8. clone a tile Reminder: Incremental Programming Write a little bit of code. Compile, debug. Repeat. B: Create a test program for the QwirkleBag class (.. .coming up in part C). Name your program QwirkleTester java. e Write a main method . Inside the main method, start with the statements QwirkleBag game1-new QwirkleBag) ystem.out.printin( "I created a new QwirkleBag. Here's what's in it:n") System.out.printin (game1)i * Now go to part C and do the steps at least up to the toString method. Then come back, compile, and run the test programStep by Step Solution
There are 3 Steps involved in it
Step: 1
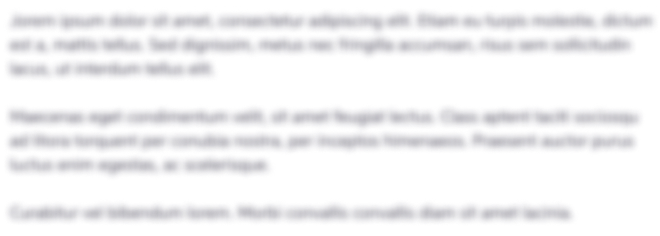
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started