Answered step by step
Verified Expert Solution
Question
1 Approved Answer
THIS IS FOR C++ ONLY. PROGRAMMING HELP BEGINS AFTER PSUEDOCODE. Pseudocode define constant OASDI_RATE 6.2% define constant FOUR01K_RATE 6% define constant MEDICARE_RATE 1.45% define constant
THIS IS FOR C++ ONLY. PROGRAMMING HELP BEGINS AFTER PSUEDOCODE. Pseudocode define constant OASDI_RATE 6.2% define constant FOUR01K_RATE 6% define constant MEDICARE_RATE 1.45% define constant MED_INS_DEDUCT_EMPL_ONLY $32.16 define constant MED_INS_DEDUCT_EMPL_ONE $64.97 define constant MED_INS_DEDUCT_EMPL_FAMILY $110.13 define constant MED_INS_STATUS_EMPL_ONLY 0 define constant MED_INS_STATUS_EMPL_ONE 1 define constant MED_INS_STATUS_EMPL_FAMILY 2 function main () int define ifstream object named fin call open_input_file (fin, "payroll.txt") -- will not return if the file could not be opened define string objects first_name, last_name read the first and last name from fin into first_name and last_name define double variables pay_rate, hrs_worked read the pay rate and hours worked from fin into pay_rate and hrs_worked define int variable med_ins_status read the medical insurance status code from fin into med_ins_status close the input file by calling fin.close() double gross_pay calc_gross_pay (pay_rate, hrs_worked) double four01k_deduct gross_pay FOUR01K_RATE double med_ins_deduct calc_med_ins_deduct (med_ins_status) double fed_tax_gross_pay gross_pay - med_ins_deduct - four01k_deduct double tax_fed calc_tax_fed (fed_tax_gross_pay) double tax_oasdi fed_tax_gross_pay OASDI_RATE double tax_medicare fed_tax_gross_pay MEDICARE_RATE double tax_state calc_tax_state (fed_tax_gross_pay) double tax_total tax_fed + tax_oasdi + tax_medicare + tax_state double net_pay fed_tax_gross_pay - tax_total define ofstream object named fout call open_output_file (fout, "paycheck.txt") -- will not return if the file could not be opened configure fout to output real numbers in fixed notation with 2 digits after the decimal point configure fout for right-justification mode send "-----------------------------", endl to fout send "EMPLOYEE: ", last_name, ", ", first_name, endl, endl to fout send "PAY RATE: $" to fout send pay_rate, endl to fout displaying the value in a field of width 8 send "HOURS: " to fout send hrs_worked, endl to fout displaying the value in a field of width 8 send "GROSS PAY: $" to fout send gross_pay, endl to fout displaying the value in a field of width 8 send "MED INS DEDUCT: $" to fout send med_ins_deduct to fout displaying the value in a field of width 8 send "401K DEDUCT: $" to fout send four01k_deduct, endl to fout displaying the value in a field of width 8 send "FED TAX GROSS PAY: $" to fout send fed_tax_gross_pay, endl to fout displaying the value in a field of width 8 send "TAX - FEDERAL: $" to fout send tax_fed, endl to fout displaying the value in a field of width 8 send "TAX - OASDI: $" to fout send tax_oasdi, endl to fout displaying the value in a field of width 8 send "TAX - MEDICARE: $" to fout send tax_medicare, endl to fout displaying the value in a field of width 8 send "TAX - STATE: $" to fout send tax_state, endl to fout displaying the value in a field of width 8 send "TAX - TOTAL: $" to fout send tax_total, endl to fout displaying the value in a field of width 8 send "NET PAY: $" to fout send net_pay, endl to fout displaying the value in a field of width 8 send "-----------------------------", endl to fout close the output file by calling fout.close() return 0 end function main function error_exit (msg : string) send msg, endl to cout call exit(-1) end function error_exit function calc_gross_pay (pay_rate : double, hrs_worked : double) double double gross_pay 0 if hrs_worked 80 then gross_pay hrs_worked pay_rate else gross_pay (80 pay_rate) + (hrs_worked - 80) (1.5 pay_rate) end if return gross_pay end function calc_gross_pay function calc_med_ins_deduct (med_ins_status : int) double med_ins_deduct 0 if med_ins_status is MED_INS_STATUS_EMPL_ONLY then med_ins_deduct MED_INS_DEDUCT_EMPL_ONLY else if med_ins_status is MED_INS_STATUS_EMPL_ONE then med_ins_deduct MED_INS_DEDUCT_EMPL_ONE else med_ins_deduct MED_INS_DEDUCT_FAMILY end if return med_ins_deduct end function calc_med_ins_deduct function calc_tax_fed (fed_tax_gross_pay : double) double double tax_fed 0 if fed_tax_gross_pay $384.62 and fed_tax_gross_pay < $1413.67 then tax_fed fed_tax_gross_pay 7.97% else if fed_tax_gross_pay $1413.67 and fed_tax_gross_pay < $2695.43 then tax_fed fed_tax_gross_pay 12.75% else if fed_tax_gross_pay $2695.43 then tax_fed fed_tax_gross_pay 19.5% end if return tax_fed end function calc_tax_fed function calc_tax_state (fed_tax_gross_pay : double) double double tax_state 0 if fed_tax_gross_pay < $961.54 then tax_state fed_tax_gross_pay 1.19% else if fed_tax_gross_pay < $2154.66 then tax_state fed_tax_gross_pay 3.44% else tax_state fed_tax_gross_pay 7.74% end if return tax_state end function calc_tax_state function open_input_file (fin : ifstream&, filename : string) fin.open(filename.c_str()) if !fin then error_exit("Could not open input file for reading.") endif end function open_input_file function open_output_file (fout : ofstream&, filename : string) fout.open(filename.c_str()) if !fout then error_exit("Could not open output file for writing.") endif end function open_output_file end of program START OF PROGRAMMING HELP. ???'s indicate HELP WITH PROGRAMMING CODES. // Include required header files. ??? // For exit() ??? // For ifstream, ofstream ??? // For setprecision(), setw() ??? // For endl, fixed ??? // For string class using namespace std; // This is the percentage rate for calculating the OASDI deduction (this is com- // monly known as social security). It is 6.2% of the employee's federal taxable // gross pay. ??? define constant OASDI_RATE // All employees are required to contribute 6.0% of their pretax gross pay to // the company 401K plan. const double FOUR01K_RATE = 0.06; // This is the percentage rate for calculating the medicare deduction. It is // 1.45% of the employee's federal taxable gross pay. ??? define constant MEDICARE_RATE // These constants are the monthly costs for each of the medical insurance // plans. The amount an employee pays depends on his or her medical insurance // status (see the next group of constants following this group). ??? define MED_INS_DEDUCT_EMPL_ONLY ??? define MED_INS_DEDUCT_EMPL_ONE ??? define MED_INS_DEDUCT_FAMILY // These constants match the numbers for the employee's medical insurance status // that will be in the input file. const int MED_INS_STATUS_EMPL_ONLY = 0; // Employee Only const int MED_INS_STATUS_EMPL_ONE = 1; // Employee + One const int MED_INS_STATUS_FAMILY = 2; // Family //------------------------------------------------------------------------------ // FUNCTION: error_exit() // // Called when something "bad" happens. Displays the specified error message // 'msg' and then terminates the program with an exit code of -1. //------------------------------------------------------------------------------ void error_exit(string msg) { cout << msg << endl; exit(-1); } //------------------------------------------------------------------------------ // FUNCTION: calc_gross_pay() // // Calculates and returns an employee's gross pay which is based on the number // of hours worked (in parameter hrs_worked) and the employee's pay rate (in // parameter pay_rate). //------------------------------------------------------------------------------ ??? Implement the pseudocode from the lab project document //------------------------------------------------------------------------------ // FUNCTION: calc_med_ins_deduct() // // Determines and returns the employee's medical insurance deduction which is // based on the employee's medical insurance status in parameter med_ins_status. //------------------------------------------------------------------------------ ??? Implement the pseudocode from the lab project document //------------------------------------------------------------------------------ // FUNCTION: calc_tax_fed() // // Calculates and returns the employee's federal income tax which is based on // his or her federal taxable gross pay (in parameter fed_tax_gross_pay) and the // federal tax withholding percentage table in the lab project document. //------------------------------------------------------------------------------ ??? Implement the pseudocode from the lab project document. ??? Hint: see calc_tax_state(). The code you will write for this function is ??? similar to the code in that function. //------------------------------------------------------------------------------ // FUNCTION: calc_tax_state() // // Calculates and returns the employee's state income tax which is based on his // or her federal taxable gross pay (in parameter fed_tax_gross_pay) and the // state tax withholding percentage table in the lab project document. //------------------------------------------------------------------------------ double calc_tax_state(double fed_tax_gross_pay) { double tax_state = 0; if (fed_tax_gross_pay < 961.54) { tax_state = fed_tax_gross_pay * 0.0119; } else if (fed_tax_gross_pay < 2145.66) { tax_state = fed_tax_gross_pay * 0.0344; } else { tax_state = fed_tax_gross_pay * 0.0774; } return tax_state; } //------------------------------------------------------------------------------ // FUNCTION: open_input_file() // // Opens the file named by filename for reading. If the file cannot be opened // then call error_exit() to terminate the program. //------------------------------------------------------------------------------ void open_input_file(ifstream& fin, string filename) { // Pass the C-string inside of filename to fin.open() to open the file. fin.open(filename.c_str()); // If the file could not be opened (most likely because it does not exist), // then the ifstream variable fin will be false. So, if fin is false, we // call the error_exit() function to terminate the program. if (!fin) { error_exit("Could not open input file for reading."); } } //------------------------------------------------------------------------------ // FUNCTION: open_output_file() // // Opens the file named by filename for writing. If the file cannot be opened // then call error_exit() to terminate the program. //------------------------------------------------------------------------------ void open_output_file(ofstream& fout, string filename) { // Pass the C-string inside of filename to fout.open() to open the file. fout.open(filename.c_str()); // If the file could not be opened then the ofstream variable fout will be // false. So, if fout is false, we call the error_exit() function to // terminate the program. if (!fout) { error_exit("Could not open output file for writing."); } } //------------------------------------------------------------------------------ // FUNCTION: main() // // This is the starting point of execution. //------------------------------------------------------------------------------ int main() { // Define an ifstream object named 'fin' to read from the input file. Then // call open_input_file() passing "payroll.txt" as the name of the file to // be opened. If the file could not be opened for reading, then open_input_ // file() will not return. ??? // Read the employee's last and first names from the input file into string // objects/variables named last_name and first_name, which must be defined. string first_name, last_name; fin >> last_name >> first_name; // Read the employee's pay rate from the input file into double variable // pay_rate, which must be defined. double pay_rate; fin >> pay_rate; // Read the employee's hours worked from the input file into double variable // hrs_worked, which must be defined. ??? // Read the employee's medical insurance status from the input file into int // variable med_ins_status, which must be defined. ??? // Close the input file because we're done reading from it. ??? // Call calc_gross_pay() to calculate the employee's gross pay. The input // params are pay_rate and hrs_worked. The return value is assigned to vari- // able gross_pay which must be defined as a double. double gross_pay = calc_gross_pay(pay_rate, hrs_worked); // Calculate the employee's mandatory contribution to his/her 401k. This is // gross_pay multiplied by the 401K rate. Assign the result to variable // four01k_deduct which must be defined as a double. ??? // Call calc_med_ins_deduct() to determine the employee's medical insurance // deduction which is based on the employee's medical insurance status // stored in med_ins_status. The return value is assigned to variable // med_ins_deduct which must be defined as a double. double med_ins_deduct = calc_med_ins_deduct(med_ins_status); // Calculate the employee's federal taxable gross pay which is gross_pay // minus the deductions for medical insurance and 401K. Assign the result // to variable fed_tax_gross_pay which must be defined as a double. ??? // Call calc_tax_fed() to calculate the employee's federal income tax. The // input parameter is fed_tax_gross_pay and the returned value is assigned to // variable tax_fed which must be defined as a double. ??? // Calculate the amount withheld for OASDI and store in tax_oasdi, which // must be defined as a double. double tax_oasdi = fed_tax_gross_pay * OASDI_RATE; // Calculate the amount withheld for medicare and store in tax_medicare, // which must be defined as a double. ??? // Call calc_tax_state() to determine the employee's state tax deduction. The // input parameter is fed_tax_gross_pay and the returned value is assigned to // variable tax_state which must be defined as a double. double tax_state = calc_tax_state(fed_tax_gross_pay); // Calculate the employee's total tax which is the sum of his/her federal // tax, OASDI tax, medicare tax, and state tax. Assign the sum to tax_total, // which must be defined as a double. ??? // Calculate the employee's net pay which is federal taxable gross pay minus // total_tax. Assign the sum to net_pay, which must be defined as a double. ??? // Define an ofstream object named 'fout' to write to the output file. Then // call open_output_file() passing "paycheck.txt" as the name of the file to // be opened. If the file could not be opened for writing, then open_output_ // file() will not return. ??? // Configure fout so real numbers will be printed in fixed notation with two // digits after the decimal point. ??? // Configure fout so the numbers will be printed right-justified in their // respective fields. ??? // Output the employee paycheck. All numerical values are output in a field // of width 8. fout << "-----------------------------" << endl; fout << "EMPLOYEE: " << last_name << ", " << first_name << endl << endl; fout << "PAY RATE: $" << setw(8) << pay_rate << endl; fout << "HOURS: " << setw(8) << hrs_worked << endl; fout << "GROSS PAY: $" << setw(8) << gross_pay << endl; fout << "MED INS DEDUCT: $" << setw(8) << med_ins_deduct << endl; fout << "401K DEDUCT: $" << setw(8) << four01k_deduct << endl; fout << "FED TAX GROSS PAY: $" << setw(8) << fed_tax_gross_pay << endl; fout << "TAX - FEDERAL: $" << setw(8) << tax_fed << endl; fout << "TAX - OASDI: $" << setw(8) << tax_oasdi << endl; fout << "TAX - MEDICARE: $" << setw(8) << tax_medicare << endl; fout << "TAX - STATE: $" << setw(8) << tax_state << endl; fout << "TAX - TOTAL: $" << setw(8) << tax_total << endl; fout << "NET PAY: $" << setw(8) << net_pay << endl; fout << "-----------------------------" << endl; // Close the output file by calling the close() function on fout. ??? // Return 0 from main() to indicate to the OS that the program terminated normally. ??? }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
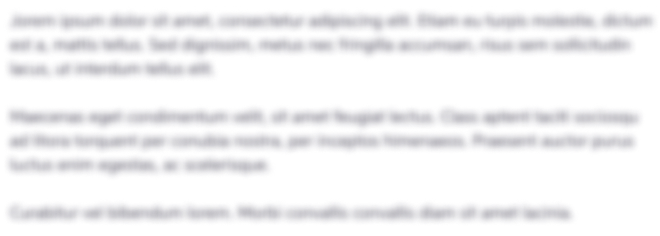
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started