Question
This is for C Programming: Please also explain the code in main.c: Thank you. The following program allows a user to add to and list
This is for C Programming: Please also explain the code in main.c: Thank you.
The following program allows a user to add to and list entries from a vector ADT, which maintains a list of employees.
Run the program, and provide input to add three employees' names and related data. Then use the list option to display the list.
Modify the program to implement the deleteEntry function.
Run the program again and add, list, delete, and list again various entries.
main.c:
#include
// Add an employee void AddEmployee(vector *employees) { Employee theEmployee; printf(" Enter the name to add: "); fgets(theEmployee.name, 50, stdin); theEmployee.name[strlen(theEmployee.name) - 1] = '\0'; // Remove trailing newline printf("Enter %s's department: ", theEmployee.name); fgets(theEmployee.department, 50, stdin); theEmployee.department[strlen(theEmployee.department) - 1] = '\0'; // Remove trailing newline printf("Enter %s's title: ", theEmployee.name); fgets(theEmployee.title, 50, stdin); theEmployee.title[strlen(theEmployee.title) - 1] = '\0'; // Remove trailing newline vector_push_back(employees, theEmployee); printf("%s's information has been added. ", theEmployee.name); return; }
// Delete an employee void DeleteEmployee(vector *employees) { printf("FIXME: Delete employee "); // FIXME: Ask the user for the employee # to delete, then delete that employee return; }
// List all employees void ListEmployees(vector *employees) { int nElements = 0; int i = 0; nElements = vector_size(employees); if (nElements > 0) { printf(" "); for (i = 0; i < nElements; ++i) { printf("%d) Name: %s", i, (*vector_at(employees, i)).name); printf(",\tDepartment: %s", (*vector_at(employees, i)).department); printf(",\tTitle: %s ", (*vector_at(employees, i)).title); } } else { printf(" There are no employees to list. "); } return; }
// Get the action the user wants to perform char GetAction(const char* prompt) { char answer[50] = ""; char firstChar = '?'; printf(" %s ", prompt); fgets(answer, 50, stdin); while (strlen(answer) == 1) { fgets(answer, 50, stdin); } firstChar = toupper(answer[0]); return firstChar; }
int main(void) { const char EXIT_CODE = 'X'; const char PROMPT_ACTION[50] = "Add, Delete, List or eXit (a,d,l,x): "; vector employees; vector_create(&employees, 0); // Create empty vector for employees char userAction = '?'; // Loop until the user enters the exit code. userAction = GetAction(PROMPT_ACTION); while (userAction != EXIT_CODE) { switch (userAction) { case 'A': AddEmployee(&employees); break; case 'D': DeleteEmployee(&employees); break; case 'L': ListEmployees(&employees); break; default : // Do nothing break; } userAction = GetAction(PROMPT_ACTION); } return 0; }
vector_employee.h:
#ifndef VECTOREMPLOYEE_H #define VECTOREMPLOYEE_H
// struct and typedef for Employee typedef struct EmployeeStruct { char name[50]; char department[50]; char title[50]; } Employee;
// struct and typedef declaration for Vector ADT typedef struct vector_struct { Employee* elements; unsigned int size; } vector;
// interface for accessing Vector ADT
// Initialize vector void vector_create(vector* v, unsigned int vectorSize);
// Destroy vector void vector_destroy(vector* v);
// Resize the size of the vector void vector_resize(vector* v, unsigned int vectorSize);
// Return pointer to element at specified index Employee* vector_at(vector* v, unsigned int index);
// Insert new value at specified index void vector_insert(vector* v, unsigned int index, Employee value);
// Insert new value at end of vector void vector_push_back(vector* v, Employee value);
// Erase (remove) value at specified index void vector_erase(vector* v, unsigned int index);
// Return number of elements within vector int vector_size(vector* v);
#endif
vecrot_employee.c:
#include
// Initialize vector with specified size void vector_create(vector* v, unsigned int vectorSize) { int i = 0; if (v == NULL) return; v->elements = (Employee*)malloc(vectorSize * sizeof(Employee)); v->size = vectorSize; for (i = 0; i < v->size; ++i) { strcpy(v->elements[i].name, ""); strcpy(v->elements[i].department, ""); strcpy(v->elements[i].title, ""); } }
// Destroy vector void vector_destroy(vector* v) { if (v == NULL) return; free(v->elements); v->elements = NULL; v->size = 0; }
// Resize the size of the vector void vector_resize(vector* v, unsigned int vectorSize) { int oldSize = 0; int i = 0; if (v == NULL) return; oldSize = v->size; v->elements = (Employee*)realloc(v->elements, vectorSize * sizeof(Employee)); v->size = vectorSize; for (i = oldSize; i < v->size; ++i) { strcpy(v->elements[i].name, ""); strcpy(v->elements[i].department, ""); strcpy(v->elements[i].title, ""); } }
// Return pointer to element at specified index Employee* vector_at(vector* v, unsigned int index) { if (v == NULL || index >= v->size) return NULL; return &(v->elements[index]); }
// Insert new value at specified index void vector_insert(vector* v, unsigned int index, Employee value) { int i = 0; if (v == NULL || index > v->size) return; vector_resize(v, v->size + 1); for (i = v->size - 1; i > index; --i) { v->elements[i] = v->elements[i-1]; } strcpy(v->elements[i].name, value.name); strcpy(v->elements[i].department, value.department); strcpy(v->elements[i].title, value.title); }
// Insert new value at end of vector void vector_push_back(vector* v, Employee value) { vector_insert(v, v->size, value); }
// Erase (remove) value at specified index void vector_erase(vector* v, unsigned int index) { int i = 0; if (v == NULL || index >= v->size) return; for (i = index; i < v->size - 1; ++i) { v->elements[i] = v->elements[i+1]; } vector_resize(v, v->size - 1); }
// Return number of elements within vector int vector_size(vector* v) { if (v == NULL) return -1; return v->size; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
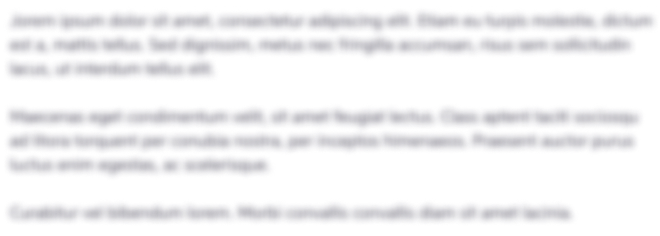
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started