Question
This is for my Unity Game Programming I class. It's a Strategy Design Pattern Programming project. Programming using the Strategy Design Pattern. You must provide
This is for my Unity Game Programming I class. It's a Strategy Design Pattern Programming project.
Programming using the Strategy Design Pattern.
You must provide code that uses and demonstrates the Strategy Design Pattern.
The goal of this project is to show off your understanding of the Design Pattern, NOT getting the project working without it.
Make sure your project has no errors or warnings.
Create one of the following ideas using the Strategy Design Pattern.
1. Score Calculations: Update the Score/Game info singleton code from week 2 to use the strategy design pattern to calculate the user's game score differently based on some kind of change. The change can either be a power up, a location (zone), time-based.
2. AI: Someone is following me: Create three game objects (cube, sphere, and cylinder). These objects will move around when the main camera is not looking at them. All three will have different strategies when it comes to moving, so you will need three different moving algorithms. This project has to work in Unity3D.
Your Strategy interface/class will NOT extend the MonoBehaviour class.
Your concrete strategy classes will NOT extend the MonoBehaviour class.
All code will be done in C#.
Here are the programming hints that was provided.
Lookup OnMouseDown from the Unity3d's manual section. Here is the link to the manual... https://docs.unity3d.com/Manual/index.html
We can write information to the console window by using: Debug.Log Debug.Log("Your message here.");
The code I put below is the Singleton design code from week two that needs to be done in the Strategy Design pattern as noted above in the instructions. I only need one of the two ideas above to be done in the Strategy Design pattern. If idea 1 is chosen then use the code posted below and change it to the Strategy Design Pattern instead of Singleton. The idea of the code below is supposed to be a coin collecting game. And the code must be in C#. This is everything I listed from the instructions that were given to me I'm sorry I can't explain any further. I appreciate the help thank you. EDIT: I posted this question before but I am posting this again because the answer I got was not in C# at it looked more C++. Thank you. I need it in C#.
Coin.cs:
using UnityEngine;
using System.Collections;
public class CoinSpawner : MonoBehaviour {
public GameObject coin; //Coin object
public float yPosition; //Position for spawn
private bool isTop; //For check last position of coin
public static CoinSpawner instance;
// Use this for initialization
void Awake() {
instance = this;
}
void Start() {
SpawnCoin(); //Spawn coin to top when start
}
public void SpawnCoin() {
GameObject c = Instantiate(coin) as GameObject; //Spawn Coin
c.name = "Coin";
c.transform.tag = "Coin";
if (isTop) {
c.transform.position = new Vector2(0.0f, -yPosition); //Spawn down Coin
} else {
c.transform.position = new Vector2(0.0f, yPosition); //Spawn Top Coin
}
isTop = !isTop;
}
}
Program.cs:
using UnityEngine;
using UnityEngine.EventSystems;
using System.Collections;
using System.Collections.Generic;
// this is player instance appy this script to the 2d sprite player with 2d collider and 2d rigidbody
public class Player : MonoBehaviour {
[HideInInspector]
public bool facingUp = true; // For determining which way the player is currently facing.
public static Player instance;
public float speed; //Speed of player
public AudioClip deadSound; //Sound When player dead
public AudioClip coinSound; //Coin Picker sound
private Vector3 direction; //Direction of player
private int fingerId = -1; //For every non-mobile Platform
private bool isDead; //For check status of Player
public List playerSprite = new List();
float timer = 5.0f;
void Awake() {
instance = this;
if (Application.isMobilePlatform) {
fingerId = 0; //for mobile and unity
}
transform.GetComponent().sprite = playerSprite[Random.Range(0, playerSprite.Length)];
direction = Vector3.up; //When starting the game. Rabbit will go to the top first.
}
// Update is called once per frame
void Update() {
//When click and Player alive and not paused.
if (Input.GetMouseButtonDown(0) && !isDead && Time.timeScale != 0) {
//For Block click through UI
if (EventSystem.current.IsPointerOverGameObject(fingerId)) {
return; //Not Change Direction
}
ChangeDirection(); //Change Direction
}
transform.Translate(direction * speed * Time.deltaTime);
}
void ChangeDirection() {
if (direction == Vector3.down) {
direction = Vector3.up; //Change direction to down
} else {
direction = Vector3.down; //Change direction to up
}
Flip();
}
void Flip()
{
// Switch the way the player is labelled as facing.
facingUp = !facingUp;
// Multiply the player's x local scale by -1.
Vector3 theScale = transform.localScale;
theScale.y *= -1;
transform.localScale = theScale;
}
void OnCollisionEnter2D(Collision2D coll) {
if (coll.transform.tag == "myrope")
{
ChangeDirection(); //Change direction when it collides with the rope.
}
//When Player collide with Obstacle while alive.
if (coll.transform.tag == "Obstacle" && !isDead) {
//Debug.Log(coll.collider.name);
isDead = true;
SoundManager.instance.PlaySingle(deadSound); //Play Dead sound
GameController.instance.GameOver(); //Call Gameover
}
}
void OnTriggerEnter2D(Collider2D coll) {
//When Player trigger with Coin while alive. && !isDead
if (coll.transform.tag == "Coin") {
Destroy(coll.gameObject); //Destroy Coin
SoundManager.instance.PlaySingle(coinSound); //Play coin picker sound
GameController.instance.addScore(); //Add score
CoinSpawner.instance.SpawnCoin(); //Spawn a new coin
}
}
}
Timer.cs:
using UnityEngine;
using System.Collections;
public class ObstacleSpawner : MonoBehaviour {
public text Timer;
public float timer;
void Update()
{
int Min1 = Mathf.FloorToInt(timer / 60F);
int Sec1 = Mathf.FloorToInt(timer - Min1 * 60);
timer -= Time.deltaTime;
Timer.Text = Sec1.tostring("00");
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
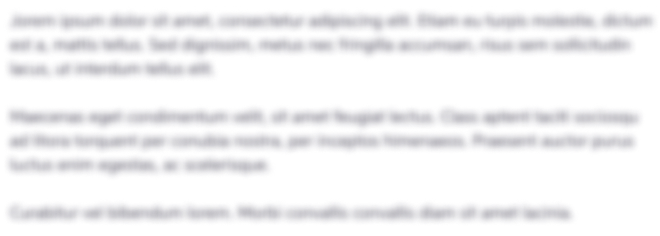
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started