Question
This is for PLP Software Tool 5.2. We are writing a program that receives a string of characters via the UART, checks if this string
This is for PLP Software Tool 5.2.
We are writing a program that receives a string of characters via the UART, checks if this string is a palindrome, and then uses a print function to print either Yes or No. A palindrome is a sequence of characters (typically a word or phrase) that is the same both forwards and backwards. For this project, strings will be terminated using a period (.). You may assume that a string will contain at least one letter in addition to a period (e.g., the input, b., should be considered a palindrome). You will not need to handle empty strings, strings containing only a period, or stings containing characters other than letters, spaces, and periods. This program should be able to handle multiple strings sent one after another or concatenated together. For example, the string: abba. data. should print Yes followed by No on the next line. Spaces should be ignored when checking for a palindrome and the palindrome should not be case sensitive. For example, A nut for a jar of Tuna. would be considered a palindrome.
Print Function
Attached is a template PLP project file. The PLP project includes a second ASM file titled, project3_lib.asm. This ASM file contains the print function used in this project. PLPTool concatenates all ASM files within a PLP project into a single location in memory (unless additional .org statements have been added to specify different location for code). No changes to project3_lib.asm should be made.
When called, depending on the value in register $a0, the following string will be displayed on the simulated UART devices output. If $a0 contains a zero then No will be displayed and if $a0 contains a non-zero value (e.g. one) then Yes will be displayed. The print function is called using the following instruction: call project3_print
To use the print function, this PLP program needs to initialize the stack pointer ($sp) before performing the function call (or any other operations involving the stack pointer). For this reason, the template project file includes an initialization that sets the stack pointer to 0x10fffffc (the last address of RAM).
Template Structure
The template project file contains six function stubs that need to be implemented. Five are called from the main loop and the sixth is called from period_check. The template file contains comments with descriptions of what each function needs to do and how it should be implemented. The table below provides a brief description of the functions.
poll_UART - Polling loop for UARTs status register, reading new character, and indicating character has been read
period_check - Checks if new character is a period and makes a nested function call to palindrome_check if it is
space_check - Skips saving space characters to array
case_check - Converts new character to uppercase if it is lowercase
array_push - Saves new character to array
palindrome_check - Moves inwards from front and back of array and compares characters at each step to determine if the string is a palindrome. If at any point mirroring characters are not equal, then it should use the print function to print No. If the comparison reaches or passes the midpoint then the print function should be used to print Yes.
Debugging Tools
To show that you properly tested your program, please save your program while it is in simulation mode (the blue simulation mode button has been toggled on) such that a CPU Memory Visualization window is open and shows at least the first 6 words of memory in the array. Please keep in mind that the starting address of the array is relative to the number of initializations placed before the assembler directive allocating space for the array. If you change your initializations you may need to adjust your CPU Memory Visualization base address. The Array Example video in this projects module demonstrates how to set up a CPU Memory Visualization window. It is acceptable to also see program contents in memory (these cells will be colored blue). For example, it would be acceptable to see the following in the visualizer after the string, abcd., has been sent by the user.
Additionally, the watcher window should contain, at minimum, registers $v0, $s1, and $s2. You may also include any other registers in the watcher window that you find useful for debugging
Points will be deducted if a CPU Memory Visualization window configured to show the start of the array does not open when simulation mode is toggled on or the watcher window does not include the minimum set of registers (the watcher window does not need to open when simulation mode is toggled on).
Rubric:
Function: poll_UART Interactions
The status register is properly polled to check when a new character has been received, the new character is copied into register $v0, a clear status command is sent, and all memory interactions use $s0 and the appropriate offset.
Function: period_check
Function goes to "palindrome_check" if a period character is found in $v0 and returns if one is not found.
Function: space_check
Function goes to "main" if a space character is found in $v0 and returns if one is not found.
Function: case_check
Function uses a single inequality to identify if $v0 is greater than 'Z' (i.e., it contains a lowercase character) and if so converts the lowercase character to its uppercase equivalent.
Function: array_push
Function properly saves the current character, $V0, at the address of the current array tail pointer, $s2, and updates the array tail pointer.
Function: palindrome_check Array Traversal
Function properly traverses the array from the front using the head pointer, $s1, and from the back using the tail pointer, $s2. During this traversal it compares characters read from both directions.
Function: palindrome_check Print Function Calls
Function contains the proper control flow to detect when the conditions are met that indicate a string is or is not a palindrome, calls the provided print function to produce the correct output, and resets to allow for the next string.
Debugging Tools: Watcher Window
When the Watcher Window is opened it contains at least registers $v0, $s1, and $s2.
Debugging Tools: CPU Memory Visualizer
When simulation mode is toggled on a CPU Memory Visualization window opens that displays at least the first 6 elements (words) of the array.
main.msm file
.org 0x10000000
# Initializations
# NOTE: You may add initializations after line 10, but please do not
# remove or change the initializations to $sp, $s0, $s1, or $s2
li $sp, 0x10fffffc # Starting address of empty stack
li $s0, 0xf0000000 # UART base address
li $s1, array_ptr # Array head pointer
li $s2, array_ptr # Array tail pointer
#################################################################### # Do not make changes to the jump to main, the allocation of # memory for the array, or the main loop ####################################################################
j main
nop
array_ptr: # Label pointing to 100 word array
.space 100
main:
jal poll_UART
nop
jal period_check
nop
jal space_check
nop
jal case_check
nop
jal array_push
nop
j main
nop
####################################################################
# ****************************************************************** ####################################################################
# The "poll_UART" function should poll the status register of the UART.
# If the 2^1 bit position (ready bit) is set to 1 then it
# should copy the receive buffer's value into $v0 and send
# a clear status command (2^1) to the command register before
# returning (a return statement is already included). In order to
# receive full credit, $s0 must contain the base address of the UART
# and must be used with the appropriate offsets to access UART
# registers and buffers
poll_UART:
jr $ra
nop
# The "period_check" function should check if the current character ($v0)
# is a period ("."). If it is a period then the function should go to the
# label, "palindrome_check". If the character is not a period then it
# should use the included return.
period_check:
jr $ra
nop
# The "space_check" function should check if the current character ($v0)
# is a space (" "). If it is then it should jump to "main" so
# that it skips saving the space character. If not it should
# use the included return.
space_check:
jr $ra
nop
# The "case_check" function should perform a single inequality check.
# If the current character ($v0) is greater than the ASCII value of 'Z',
# which indicates the current character is lowercase, then it should convert
# the value of $v0 to the uppercase equivalent and then return. If the
# current character ($v0) is already uppercase (meaning the inequality
# mentioned before was not true) then the function should return without
# performing a conversion.
case_check:
jr $ra
nop
# The "array_push" function should save the current character ($v0) to the
# current location of the tail pointer, $s2. Then it should increment the
# tail pointer so that it points to the next element of the array. Last
# it should use the included return statement.
array_push:
jr $ra
nop
# The "palindrome_check" subroutine should be jumped to by the period
# check function if a period is encountered. This subroutine should contain
# a loop that traverses the array from the front towards the back (using the
# head pointer, $s1) and from the back towards the front(using the tail
# pointer, $s2). If the string is a palindrome then as the array is traversed
# the characters pointed to should be equal. If the characters are not equal
# then the string is not a palindrome and the print function should be used
# to print "No". If the pointers cross (i.e. the head pointer's address is
# greater than or equal to the tail pointer's address) and the compared
# characters are equal then the string is a palindrome and "Yes" should be
# printed.
#
# Remember to restore the head and tail pointers to the first element
# of the array before the subroutine jumps back to main to begin processing the
# next string. Also, keep in mind that because the tail pointer is updated at
# the end of "array_push" it technically points one element past the last
# character in the array. You will need to compensate for this by either
# decrementing the pointer once at the start of the array or using an offset
# from this pointer's address.
palindrome_check:
j main
nop
project3_lib.asm
li $a0, control_message_p3
jal libplp_uart_write_string_p3
nop
control_flow_trap_p3:
j control_flow_trap_p3
nop
string_yes_p3:
.asciiz "Yes "
string_no_p3:
.asciiz " No "
control_message_p3:
.asciiz "Error: Program entered project3_print.asm due to missing control flow at the
end of main.asm "
project3_print:
push $ra
bne $a0 $0, set_ptr_yes
nop
li $a0, string_no_p3
j print_string_p3
nop
set_ptr_yes:
li $a0, string_yes_p3
print_string_p3:
jal libplp_uart_write_string_p3
nop
pop $ra
return
# From PLP UART Library
libplp_uart_write_p3:
lui $t0, 0xf000 #uart base address
libplp_uart_write_loop_p3:
lw $t1, 4($t0) #get the uart status
andi $t1, $t1, 0x01 #mask for the cts bit
beq $t1, $zero, libplp_uart_write_loop_p3
nop
sw $a0, 12($t0) #write the data to the output buffer
sw $t1, 0($t0) #send the data!
jr $31
nop
libplp_uart_write_string_p3: #we have a pointer to the string in a0, just loop and increment until we see a \0
move $t9, $31 #save the return address
move $t8, $a0 #save the argument
libplp_uart_write_string_multi_word_p3:
lw $a0, 0($t8) #first 1-4 characters
ori $t0, $zero, 0x00ff #reverse the word to make it big endian
and $t1, $t0, $a0 #least significant byte
sll $t1, $t1, 24
srl $a0, $a0, 8
and $t2, $t0, $a0 #second byte
sll $t2, $t2, 16
srl $a0, $a0, 8
and $t3, $t0, $a0 #third byte
sll $t3, $t3, 8
srl $a0, $a0, 8 #last byte in a0
or $a0, $t1, $a0
or $a0, $t2, $a0
or $a0, $t3, $a0
beq $a0, $zero, libplp_uart_write_string_done_p3
nop
ori $t7, $zero, 4
libplp_uart_write_string_loop_p3:
jal libplp_uart_write_p3 #write this byte
addiu $t7, $t7, -1
srl $a0, $a0, 8
bne $a0, $zero, libplp_uart_write_string_loop_p3
nop
beq $t7, $zero, libplp_uart_write_string_multi_word_p3
addiu $t8, $t8, 4 #increment for the next word
libplp_uart_write_string_done_p3:
jr $t9 #go home
nop
Step by Step Solution
There are 3 Steps involved in it
Step: 1
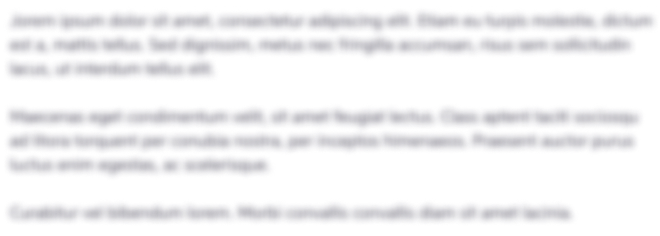
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started