Question
This is in C++. How would validate the weight, height and depth without asking for user input in this Box class() program? #include #include using
This is in C++. How would validate the weight, height and depth without asking for user input in this Box class() program?
#include
#include
using namespace std;
// A Box class consisting of data members height, width and depth declared as private which can't be accesible outside class class Box { private: double height, width, depth; public: // Default constructor which initializes width, height & depth to 1.0 Box() { height = width = depth = 1.0; } // Parameterized constructor used for initializing data members of class Box(double h, double w, double d) { height = h; width = w; depth = d; } // Increasing dimensions for the box by using resize function and storing it in height, width & depth void resize(double h, double w, double d) { height = h; width = w; depth = d; } // Used for initializing height value void set_height(double h) { height = h; // Checks height value and if it is less than 0.01 then an invalid_argument exception will be thrown with a message if(h < 0.01) { throw invalid_argument("Height is less than 0.01."); } } // Used for initializing width value void set_width(double w) { width = w; // Checks width value and if it is less than 0.01 then an invalid_argument exception will be thrown with a message if(w < 0.01) { throw invalid_argument("Width is less than 0.01."); } } // Used for initializing depth value void set_depth(double d) { depth = d; // Checks width value and if it is less than 0.01 then an invalid_argument exception will be thrown with a message if(d < 0.01) { throw invalid_argument("Depth is less than 0.01."); } } // Returns dimensions double get_height() { return height; } double get_width() { return width; } double get_depth() { return depth; } // Returns volume of box double volume() { return height * width * depth; } // Print the contents of object void toString() { cout << "Height:\t" << height << endl; cout << "Width:\t" << width << endl; cout << "Depth:\t" << depth << endl; cout << endl; } };
int main() { // Box b1 calling default constructor and Box b2 calling parameterized constructor Box b1, b2(9, 8, 3); cout << "Box b1:" << endl; // Print b1 contents b1.toString(); cout << "Box b2:" << endl; // Print b2 contents b2.toString(); // Calculate volume of boxes b1 & b2 cout << "Volume of b1: " << b1.volume() << endl; cout << "Volume of b2: " << b2.volume() << endl; cout << endl; cout << "Changing width of b2 to 0.9:" << endl; // Changing width of second box b2.set_width(0.90);
cout << "Changing width of b2 to 0.90, width: " << b2.get_width() << endl; cout << "Volume: " << b2.volume() << endl; cout << endl; // Resizing first box b1.resize(2.3, 1.5, 5.2); cout << "After resizing b1:" << endl; // Printing box b1 contents after resizing its dimensions b1.toString(); cout << "New volume:" << endl; // Calculating volume of new box cout << "Volume: " << b1.volume() << endl;
try { cout << "If width is set under 0.01:" << endl; b1.set_width(0.001); } // Exception handler catches the exception thrown by set_width() function and handles it catch(invalid_argument e) { cout << "0.01 is the minimum width for the box!" << endl; }
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
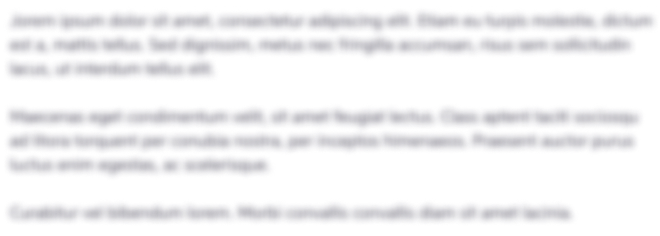
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started