Question
This is in subject of Java I'm having a hard time figuring out why the hourly or salary input (h or s) gets immediately processed
This is in subject of Java I'm having a hard time figuring out why the hourly or salary input (h or s) gets immediately processed through the checkHours(sc). What I intended to happen in this code is to have the user input employee number, social security number, first and last name, and email and then asking if they're hourly or salary. If they're hourly, it will ask the user to input the amount of hours worked. If it does not pass the validation checkHours(sc) it will go through that method accordingly (checking if it's present, integer, within range, etc). If they're salary it should do the same. Then it should print out employee's number, social security number, first and last name, email, and their calculated pay.
The validation class is already created for us by the instructor, the instructor wishes for us not to modify it.
package business; import java.text.NumberFormat; import java.time.LocalDate; import java.time.format.DateTimeParseException; import java.math.*;
public class Validation { public static boolean isStringPresent(String stringData, String title) { if(stringData.isEmpty()) { System.out.println(title + " must be present, please re-enter: "); return false; } return true; }
public static boolean isInteger(String intData, String title) { try { int i = Integer.parseInt(intData); return true; } catch (NumberFormatException e) { System.out.println(title + " must be an integer. Please re-enter."); return false; } }
public static boolean isWithinRangeInteger(String intData, int min, int max, String title) { int i = Integer.parseInt(intData); if (i max) { System.out.println(title + " must be between " + min + " and " + max + ". " + " Please re-enter."); return false; } return true; }
public static boolean isLong(String longData, String title) { try { long i = Long.parseLong(longData); return true; } catch (NumberFormatException e) { System.out.println(title + " must be an integer. Please re-enter."); return false; } }
public static boolean isWithinRangeLong(String longData, long min, long max, String title) { long i = Long.parseLong(longData); if (i max) { System.out.println(title + " must be between " + min + " and " + max + ". " + " Please re-enter."); return false; } return true; } public static boolean isDouble(String doubleData, String title) { try { double d = Double.parseDouble(doubleData); return true; } catch (NumberFormatException e) { System.out.println(title + " must be a numeric value. Please re-enter."); return false; } } public static boolean isWithinRangeDouble(String doubleData, double min, double max, String title) { double d = Double.parseDouble(doubleData); if (d max) { System.out.println(title + " must be between " + min + " and " + max + ". " + " Please re-enter."); return false; } return true; }
public static boolean isDateValid(String date) { try { LocalDate parsedDate = LocalDate.parse(date);//parses the String into a LocalDate object return true; } catch (DateTimeParseException e) { System.out.println("Date must be in this format: yyyy-mm-dd & must be a valid date " + ". " + "Please re-enter."); return false; } }
public static BigDecimal formatRound(double number) { BigDecimal decimalRound = new BigDecimal(number); return decimalRound = decimalRound.setScale(2, RoundingMode.HALF_UP); } public static String formatAndRound(double number) { NumberFormat num = NumberFormat.getCurrencyInstance();
BigDecimal decimalRound = new BigDecimal(number); return num.format(decimalRound = decimalRound.setScale(2, RoundingMode.HALF_UP)); } }
1 package business; 2 public abstract class AbstractEmployeeAccounts 10 4 5 6 7 8 9 10- 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 280 29 30 31 32 33 34 35 360 37 38 39 400 41 42 43 440 45 46 47 private int empNum; private int empSSN; private String lastName; private String firstName; private String email; Abstract EmployeeAccounts() { empNum = 0; empSSN lastName = firstName = email } AbstractEmployeeAccounts(int empNum, int empSSN, String firstName, String lastName, String email) { this.empNum = empNum; this.empSSN = empSSN; this.lastName = lastName; this.firstName = firstName; this.email = email; } 18 public int getEmpNum() { return empNum; } public void setEmplum(int empNum) { this.empNum = empNum; } public int getEmpSSN() { return empSSN; } public void setEmpSSN(int empSSN) { this.empSSN - empSSN; } public String getLastName() { return lastName; } 480 public void setLastName(String lastName) { this.lastName = lastName; } 49 50 51 520 53 54 55 560 57 58 59 600 public String getFirstName() { return firstName; } public void setFirstName (String firstName) { this.firstName = firstName; } public String getEmail() { public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } 600 61 62 63 64 650 66 67 68 696 70 171 72 73 74 75 76 77 78 79 80} public String toString() { return "Employee Number: " + empNum + " " + "Social Security Number: + empSSN + " " + "First Name:" + firstName + " " + "Last Name: + lastName + " " + "Email: + email + " "; } public abstract double calcPay(double b); 40 5 7 8 90 10 11 12 13 140 15 16 17 18 19 1 package business; 2 public class Salary extends AbstractEmployeeAccounts public Salary() { 6 super(); } public Salary(int empNum, int empssn, String firstName, String lastName, String email) { super(empNum, empSsn, firstName, lastName, email); } public double calcPay(double salary) { double totalPay; totalPay salary/12; return totalPay; } 20 | 1 package business; 2 3 public class Hourly extends Abstract Employee Accounts 4 { 50 public Hourly 6 { super(); } 9 10- public Hourly(int empNum, int empssN, String firstName, String lastName, String email) { super (empNum, empSSN, firstName, lastName, email); 7 8 11 12 13 14 150 16 17 18 19 20 21 22 public double calcPay(double hours) { double totalPay; totalPay = hours * 15.95; return totalPay; } ? 1 package presentation; 30 import java.util.Scanner; 4 6 5 import business. Abstract EmployeeAccounts; import business. Hourly; 7 import business. Salary; 8 import business. Validation; 10 public class EmployeeAccount 11 { 120 public static void main(String args[]) 13 { 14 Scanner sc = new Scanner(System.in); 15 String continueAnswer = ""; 16 17 do 18 { 19 int empNum = checkEmpNum(sc); 20 int empSSN = checkEmpSSN(SC); 21 String firstName = checkFirstName (sc); 22 String lastName = checkLastName (sc); 23 String email = checkEmail(sc); 24 25 System.out.print("Hourly or salary? Enter h for hourly or s for salary "); 26 String response = sc.next(); 27 28 if (response.equalsIgnoreCase("h")) 29 { 30 double totalPay; 31 int hours = checkHours(sc); 32 Hourly hourly= new 33 Hourly(empNum, empSSN, firstName, lastName, email); 34 totalPay = hourly.calcPay(hours); 35 hourly.toString(); 36 System.out.print("Total pay: " + totalPay); 37 } 38 39 else if (response.equalsIgnoreCase("s")) 40 { 41 double totalPay; 42 int annualSalary = checkSalary(sc); 43 Salary salary = new 44 Salary(empNum, empSSN, firstName, lastName, email); 45 totalPay = salary.calcPay(annualSalary); 46 salary.toString(); 47 System.out.print("Total pay: " + totalPay); 48 } 49 50 System.out.print(" Do you wish to continue? "); 51 continueAnswer = sc.next(); 52 sc.nextLine(); 53 }while(continueAnswer.equalsIgnoreCase("y")); 54 } 550 public static int checkEmpNum(Scanner sc) 56 57 String answer = ""; 58 int min = 1000; 59 int max = 9999; 60 boolean errorCheck = false; boolean errorCheck = false; do { System.out.print("Enter employee number: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Employee number")&& Validation.isInteger(answer, "Employee number")&& Validation.iswithinRange Integer (answer, min, max, "Employee number"); }while(!errorCheck); int empNum = Integer.parseInt(answer); return empNum; } 11 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74- 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 public static int checkEmpSSN(Scanner sc) { String answer = int min = 111111111; int max = 999999999; boolean errorCheck = false; do { System.out.print(" Enter employee's social security number: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent(answer, "Employee social security number")&& Validation. isInteger(answer, "Employee social security number")&& Validation.iswithinRange Integer (answer, min, max, "Employee social security number"); }while(!errorCheck); int empSSN = Integer.parseInt(answer); return empSSN; } 932 94 95 96 private static String checkFirstName (Scanner sc) { String answer = ""; boolean errorCheck = false; 97 do 98 99 100 101 102 103 104 105 106 107 108- 109 110 { System.out.print(" Enter employee's first name: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Employee's first name"); } while(!errorCheck); String firstName = answer; return firstName; } private static String checkLastName (Scanner sc) { String answer = ""; boolean errorCheck = false; do { System.out.print(" Enter employee's last name: "); answer - sc.nextLine(); errorCheck = Validation. isStringPresent(answer, "Employee's last name"); } while(!errorCheck); Strine lastName = answer: 111 112 113 114 115 116 117 118 119 String lastName = answer; return lastName; } 111 private static String checkEmail(Scanner sc) { String answer = boolean errorCheck = false; do { System.out.print(" Enter employee's email: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Employee's email"); } while(!errorCheck); String email = answer; return email; } private static int checkHours (Scanner sc) { String answer = int min = 1; int max = 40; boolean errorCheck = false; { System.out.print(" Enter hours worked: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Hours worked")&& Validation.isInteger(answer, "Hours worked")&& Validation. isWithinRange Integer (answer, min, max, "Hours worked"); } while(!errorCheck); int hours = Integer.parseInt(answer); return hours; } 111 119 120 121 122 1236 124 125 126 127 128 129 130 131 132 133 134 135 136 | 137 1380 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 } do private static int checksalary(Scanner sc) { String answer = int min = 12000; int max = 125000; boolean errorCheck = false; do { System.out.print(" Enter salary: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Salary")&& Validation. isInteger (answer, "Salary") && Validation. isWithinRange Integer(answer, min, max, "Salary"); } while(!errorCheck); int salary = Integer.parseInt(answer); return salary: } 1 package business; 2 public abstract class AbstractEmployeeAccounts 10 4 5 6 7 8 9 10- 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 280 29 30 31 32 33 34 35 360 37 38 39 400 41 42 43 440 45 46 47 private int empNum; private int empSSN; private String lastName; private String firstName; private String email; Abstract EmployeeAccounts() { empNum = 0; empSSN lastName = firstName = email } AbstractEmployeeAccounts(int empNum, int empSSN, String firstName, String lastName, String email) { this.empNum = empNum; this.empSSN = empSSN; this.lastName = lastName; this.firstName = firstName; this.email = email; } 18 public int getEmpNum() { return empNum; } public void setEmplum(int empNum) { this.empNum = empNum; } public int getEmpSSN() { return empSSN; } public void setEmpSSN(int empSSN) { this.empSSN - empSSN; } public String getLastName() { return lastName; } 480 public void setLastName(String lastName) { this.lastName = lastName; } 49 50 51 520 53 54 55 560 57 58 59 600 public String getFirstName() { return firstName; } public void setFirstName (String firstName) { this.firstName = firstName; } public String getEmail() { public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } 600 61 62 63 64 650 66 67 68 696 70 171 72 73 74 75 76 77 78 79 80} public String toString() { return "Employee Number: " + empNum + " " + "Social Security Number: + empSSN + " " + "First Name:" + firstName + " " + "Last Name: + lastName + " " + "Email: + email + " "; } public abstract double calcPay(double b); 40 5 7 8 90 10 11 12 13 140 15 16 17 18 19 1 package business; 2 public class Salary extends AbstractEmployeeAccounts public Salary() { 6 super(); } public Salary(int empNum, int empssn, String firstName, String lastName, String email) { super(empNum, empSsn, firstName, lastName, email); } public double calcPay(double salary) { double totalPay; totalPay salary/12; return totalPay; } 20 | 1 package business; 2 3 public class Hourly extends Abstract Employee Accounts 4 { 50 public Hourly 6 { super(); } 9 10- public Hourly(int empNum, int empssN, String firstName, String lastName, String email) { super (empNum, empSSN, firstName, lastName, email); 7 8 11 12 13 14 150 16 17 18 19 20 21 22 public double calcPay(double hours) { double totalPay; totalPay = hours * 15.95; return totalPay; } ? 1 package presentation; 30 import java.util.Scanner; 4 6 5 import business. Abstract EmployeeAccounts; import business. Hourly; 7 import business. Salary; 8 import business. Validation; 10 public class EmployeeAccount 11 { 120 public static void main(String args[]) 13 { 14 Scanner sc = new Scanner(System.in); 15 String continueAnswer = ""; 16 17 do 18 { 19 int empNum = checkEmpNum(sc); 20 int empSSN = checkEmpSSN(SC); 21 String firstName = checkFirstName (sc); 22 String lastName = checkLastName (sc); 23 String email = checkEmail(sc); 24 25 System.out.print("Hourly or salary? Enter h for hourly or s for salary "); 26 String response = sc.next(); 27 28 if (response.equalsIgnoreCase("h")) 29 { 30 double totalPay; 31 int hours = checkHours(sc); 32 Hourly hourly= new 33 Hourly(empNum, empSSN, firstName, lastName, email); 34 totalPay = hourly.calcPay(hours); 35 hourly.toString(); 36 System.out.print("Total pay: " + totalPay); 37 } 38 39 else if (response.equalsIgnoreCase("s")) 40 { 41 double totalPay; 42 int annualSalary = checkSalary(sc); 43 Salary salary = new 44 Salary(empNum, empSSN, firstName, lastName, email); 45 totalPay = salary.calcPay(annualSalary); 46 salary.toString(); 47 System.out.print("Total pay: " + totalPay); 48 } 49 50 System.out.print(" Do you wish to continue? "); 51 continueAnswer = sc.next(); 52 sc.nextLine(); 53 }while(continueAnswer.equalsIgnoreCase("y")); 54 } 550 public static int checkEmpNum(Scanner sc) 56 57 String answer = ""; 58 int min = 1000; 59 int max = 9999; 60 boolean errorCheck = false; boolean errorCheck = false; do { System.out.print("Enter employee number: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Employee number")&& Validation.isInteger(answer, "Employee number")&& Validation.iswithinRange Integer (answer, min, max, "Employee number"); }while(!errorCheck); int empNum = Integer.parseInt(answer); return empNum; } 11 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74- 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 public static int checkEmpSSN(Scanner sc) { String answer = int min = 111111111; int max = 999999999; boolean errorCheck = false; do { System.out.print(" Enter employee's social security number: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent(answer, "Employee social security number")&& Validation. isInteger(answer, "Employee social security number")&& Validation.iswithinRange Integer (answer, min, max, "Employee social security number"); }while(!errorCheck); int empSSN = Integer.parseInt(answer); return empSSN; } 932 94 95 96 private static String checkFirstName (Scanner sc) { String answer = ""; boolean errorCheck = false; 97 do 98 99 100 101 102 103 104 105 106 107 108- 109 110 { System.out.print(" Enter employee's first name: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Employee's first name"); } while(!errorCheck); String firstName = answer; return firstName; } private static String checkLastName (Scanner sc) { String answer = ""; boolean errorCheck = false; do { System.out.print(" Enter employee's last name: "); answer - sc.nextLine(); errorCheck = Validation. isStringPresent(answer, "Employee's last name"); } while(!errorCheck); Strine lastName = answer: 111 112 113 114 115 116 117 118 119 String lastName = answer; return lastName; } 111 private static String checkEmail(Scanner sc) { String answer = boolean errorCheck = false; do { System.out.print(" Enter employee's email: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Employee's email"); } while(!errorCheck); String email = answer; return email; } private static int checkHours (Scanner sc) { String answer = int min = 1; int max = 40; boolean errorCheck = false; { System.out.print(" Enter hours worked: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Hours worked")&& Validation.isInteger(answer, "Hours worked")&& Validation. isWithinRange Integer (answer, min, max, "Hours worked"); } while(!errorCheck); int hours = Integer.parseInt(answer); return hours; } 111 119 120 121 122 1236 124 125 126 127 128 129 130 131 132 133 134 135 136 | 137 1380 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 } do private static int checksalary(Scanner sc) { String answer = int min = 12000; int max = 125000; boolean errorCheck = false; do { System.out.print(" Enter salary: "); answer = sc.nextLine(); errorCheck = Validation. isStringPresent (answer, "Salary")&& Validation. isInteger (answer, "Salary") && Validation. isWithinRange Integer(answer, min, max, "Salary"); } while(!errorCheck); int salary = Integer.parseInt(answer); return salary: }Step by Step Solution
There are 3 Steps involved in it
Step: 1
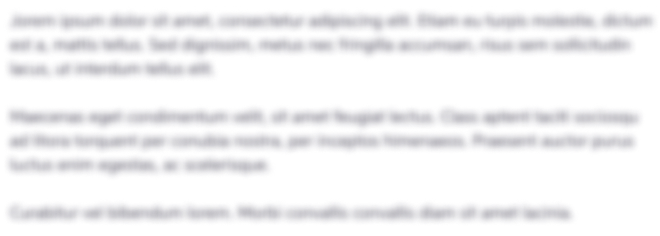
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started