Answered step by step
Verified Expert Solution
Question
1 Approved Answer
this is IndexNode.java this is IndexTree.java this is ReadingFromFileExample.java this is txt example 1 Your Assignment We will create an IndexTree, a special type of
this is IndexNode.java
this is IndexTree.java
this is ReadingFromFileExample.java
this is txt example
Step by Step Solution
There are 3 Steps involved in it
Step: 1
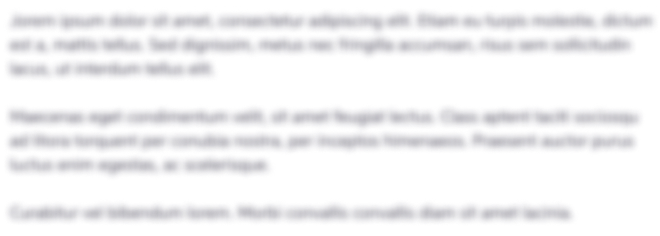
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started