Question
This is my code from HW7: public class House { private Room[] myHome ; public House( int rms) { myHome = new Room[rms]; } public
This is my code from HW7:
public class House {
private Room[] myHome;
public House(int rms) {
myHome = new Room[rms];
}
public void showHouse() {
System.out.println("This " + getNumBedrooms() + " bedroom house has " + myHome.length + " rooms; total area is " + getSqFeet() + " sq feet");
for (int incr = 0; incr myHome.length; incr++) {
System.out.println(" " + myHome[incr]);
}
}
public double getSqFeet() {
double area = 0;
for (int incr = 0; incr myHome.length; incr++) {
area += myHome[incr].getSqFeet();
}
return area;
}
public double getNumBedrooms() {
double brs = 0;
for (int incr = 0; incr myHome.length; incr++) {
if ( (myHome[incr].getClass() == Bedroom.class)
|| (myHome[incr].getClass().getSuperclass() == Bedroom.class) ){
brs++;
}
}
return brs;
}
public static void main(String[] args) {
House h = new House(8);
int rooms = 0;
h.myHome[rooms++] = new Kitchen(12, 16);
h.myHome[rooms++] = new LivingRoom(16, 20, true);
h.myHome[rooms++] = new MasterBedroom(17, 17);
h.myHome[rooms++] = new Bedroom(13, 13);
h.myHome[rooms++] = new Bedroom(0, 0);
h.myHome[rooms++] = new Bathroom(9, 9, "three-quarter");
h.myHome[rooms++] = new Bathroom(8, 11, "full");
h.myHome[rooms++] = new Den(14, 15.5, false, true);
h.myHome[4].setSize(12.5, 14.5);
h.showHouse();
System.out.println(); // blank line
House tinyHouse = new House(3);
rooms = 0;
tinyHouse.myHome[rooms++] = new Kitchen(5, 6);
tinyHouse.myHome[rooms++] = new MasterBedroom(10, 6);
tinyHouse.myHome[rooms++] = new Bathroom(5, 6, "three-quarter");
tinyHouse.showHouse();
}
}
public abstract class Room {
protected static final double HUGESIZE = 5280; // no room is more than a mile wide or long
protected double width, length;
public Room() {
this(0, 0);
}
public Room(double wd, double ln) {
this.setSize(wd, ln);
}
public boolean setSize(double wd, double ln) {
if ( (wd >= 0) && (wd HUGESIZE) && (ln >= 0) && (ln HUGESIZE)) {
width = wd;
length = ln;
return true; // indicates success
}
return false; // indicates failure
}
public double getSqFeet() { return width*length; }
public double getWidth() { return width; }
public double getLength() { return length; }
public String toString() {
return "WD x LN: [" + width + " x " + length + "] ";
}
}
public class Bathroom extends Room {
private String bathType;
public Bathroom() {
super();
bathType = "full";
}
public Bathroom(double w, double l, String type) {
super(w, l);
bathType = type;
}
public String toString() {
return "Bathroom: " + super.toString() + bathType;
}
}
public class Bedroom extends Room {
public Bedroom() {
super();
}
public Bedroom(double w, double l) {
super(w, l);
}
public String toString() {
return "Bedroom: " + super.toString();
}
}
public class Kitchen extends Room {
public Kitchen() {
super();
}
public Kitchen(double w, double l) {
super(w, l);
}
public String toString() {
return "Kitchen: " + super.toString();
}
}
public abstract class SittingRoom extends Room {
private boolean hasCeilingFan;
public SittingRoom() {
super();
hasCeilingFan = false;
}
public SittingRoom(double w, double l, boolean f) {
super(w, l);
hasCeilingFan = f;
}
public String toString() {
return super.toString() + (hasCeilingFan ? "w/fan " : "");
}
}
public class Den extends SittingRoom {
boolean hasFireplace;
public Den() {
super();
hasFireplace = true; // for now, all Dens have fireplaces
}
public Den(double w, double l, boolean fan, boolean fp) {
super(w, l, fan);
hasFireplace = fp;
}
public String toString() {
return "Den: " + super.toString() + (hasFireplace ? "w/fireplace" : "");
}
}
public class LivingRoom extends SittingRoom {
public LivingRoom() {
super();
}
public LivingRoom(double w, double l, boolean fan) {
super(w, l, fan);
}
public String toString() {
return "LivingRoom: " + super.toString();
}
}
public class MasterBedroom extends Bedroom {
public MasterBedroom() {
super();
}
public MasterBedroom(double w, double l) {
super(w, l);
}
public String toString() {
return "Master " + super.toString();
}
}
In this assignment, you will add suitable equality operators as required to the class hierarchy that you developed in HW7. As discussed in class, testing the equality of objects of class type is tricky and requires a good equals() method in the class definition. With an inheritance hierarchy like this, you need to decide if every class needs its own equals method or if we can inherit a suitable method from the superclass in some cases. For this assignment, you are to add a suitable equals method for the classes in the House assignment. You should not add any more code to the classes than you must. To test your resulting classes, here is a method that will test useful s and write the results. It's an ugly routine and there are better ways to do this, but I wanted it to be as simple to read as possible
Step by Step Solution
There are 3 Steps involved in it
Step: 1
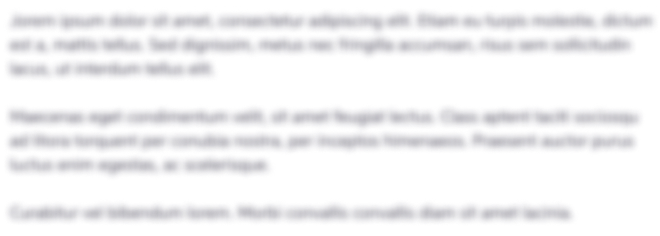
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started