Question
THIS IS THE CODE class Department(): Department class - creates Department objects, each holding a roster of student objects majoring in the Department ----------------------------------------------------------
THIS IS THE CODE
class Department(): """ Department class - creates Department objects, each holding a roster of student objects majoring in the Department ---------------------------------------------------------- """ univ_students = 0 # class variable - total # students in all Departments count = 0 # class variable - total # Departments created
def __init__(self, d_code= '', d_name = '', capacity = 5, minGPA = 2.5): self.d_code = d_code self.d_name = d_name self.capacity = capacity self.minGPA = minGPA self.num_students = 0 self.avgGPA = 0.0 self.roster = [ ] # self.qualified = True # internal attribute used by addStudent() self.reason = '' #
def addStudent(self, s_obj): if not s_obj or not isinstance(s_obj, Student): return False, 'add failed: obj type' else: self.qualified, self.reason = self.isQualified(s_obj) if self.qualified: self.roster.append(s_obj) self.num_students += 1 Department.univ_students += 1 self.calcAvgGPA() s_obj.setMajor(self.d_code) # set Student's major to Dept else: return False, self.reason return True, 'added' def isQualified (self, s_obj): if self.num_students >= self.capacity: # Check Dept. capacity return (False, 'add failed: over capacity') elif s_obj.gpa() min return (False, 'add failed: minGPA') elif not s_obj.isEnrolled(): # Student must be enrolled return (False, 'add failed: not enrolled') else: if len(self.roster) > 0: # Check student not already for s in self.roster: # in Dept. major roster if s.sameStudent(s_obj): return (False, 'add failed: already in Department') return (True, '')
def calcAvgGPA(self): # recalculates Dept. avg GPA self.avgGPA = 0 for s in self.roster: self.avgGPA += s.gpa() return (round(self.avgGPA / len(self.roster),2))
def printRoster(self): print(' List of students in Department ', self.d_name) for s in self.roster: print(s)
class Student(): """ Student Class - creates Student objects ---------------------------------------- """ def __init__(self, g_num, name, status = 'Freshman', major = 'IST', enrolled = 'y', credits = 0, qpoints = 0): self.g_num = str(g_num) self.name = str(name) self.status = str(status) self.major = str(major) self.enrolled = str(enrolled) self.credits = credits self.qpoints = qpoints def __str__(self):
return(str(self.name) + ', ' + str(self.g_num) + ', ' + str(self.status) + ', ' + str(self.major) + ', active: ' + str(self.enrolled) + ', credits = ' + str(self.credits) + ', gpa = {0:4.2f}'.format(self.gpa())) # calculate gpa, don't store def gpa (self) : if self.credits > 0: # prevent division by zero... return self.qpoints / self.credits else : return 0 # ...if zero credits, return gpa = 0 def setMajor (self, major) : self.major = major return True
def sameStudent (self, s_obj): return(self.g_num == str(s_obj.g_num) and self.name == str(s_obj.name) ) # return True if g_num, name match def isEnrolled (self): return (self.enrolled == 'y') # return True if student is enrolled
print(' Start of Department and Student class demo **************') s1 = Student('G34568', 'David Miller', status = 'sophomore', major = 'Hist', enrolled = 'y', credits = 30, qpoints = 90) s2 = Student('G21345', 'Sonia Fillmore', status = 'senior', major = 'Math', enrolled = 'y', credits = 90, qpoints = 315) s3 = Student('G42156', 'Chris Squire', status = 'sophomore', major = 'Musc', enrolled = 'y', credits = 45, qpoints = 160) s4 = Student('G10928', 'Tal wilkenfeld', status = 'junior', major = 'ARTS', enrolled = 'y', credits = 70, qpoints = 225) s5 = Student('G22157', 'Larry Graham', status = 'senior', major = 'CHHS', enrolled = 'y', credits = 105, qpoints = 290) s6 = Student('G31345', 'John Entwistle', status = 'freshman', major = 'CSci', enrolled = 'y', credits = 15, qpoints = 35) s7 = Student('G44568', 'Esperanza Spalding', status = 'junior', major = 'ENGR', enrolled = 'y', credits = 65, qpoints = 250) s8 = Student('G55345', 'Tim Bogert', status = 'sophomore', major = 'Hist', enrolled = 'y', credits = 45, qpoints = 120) s9 = Student('G66113', 'Gordon Sumner', status = 'freshman', major = 'Musc', enrolled = 'y', credits = 15, qpoints = 45) s10 = Student('G11311', 'Paul McCartney', status = 'senior', major = 'ARTS', enrolled = 'y', credits = 110, qpoints = 275) s11 = Student('G22111', 'Elizabeth Smythe', status = 'junior', major = 'ENGR', enrolled = 'y', credits = 85, qpoints = 250) s12 = Student('G31312', 'John McVie', status = 'sophomore', major = 'Hist', enrolled = 'y', credits = 45, qpoints = 120) s13 = Student('G31312', 'Nawt Enrolled', status = 'sophomore', major = 'Hist', enrolled = 'n', credits = 45, qpoints = 120) s14 = Student('G11112', 'Toolow G. Peyay', status = 'freshman', major = 'Undc', enrolled = 'y', credits = 20, qpoints = 38) print('List of Students created: ') print('s2= ',s1) print('s2= ',s2) print('s3= ',s3) print('s4= ',s4) print('s5= ',s5) print('s6= ',s6) print('s7= ',s7) print('s8= ',s8) print('s9= ', s9) print('s10= ',s10) print('s11= ',s11) print('s12= ',s12) print('s13= ',s13) print('s14= ',s14) d1 = Department('ENGR', 'Engineering', 5, 2.75) d2 = Department('ARTS', 'Art and Architecture', 15, 2.0) d3 = Department('CHHS', 'College of Health and Human Sevrices', 10, 2.5) print(' Departments established:') print(d1) print(d2) print(d3) print(' Adding s1 - s5 to ENGR Department- print Roster follows') d1.addStudent(s1) d1.addStudent(s2) d1.addStudent(s3) d1.addStudent(s4) d1.addStudent(s5) d1.printRoster() a, b = d1.addStudent(s6) print(' Attempting to add ', s6.name, ' to ', d1.d_code, ' - over capacity, ret values: ', a, b) d1.printRoster()
print(' Adding ', s6.name, ' and ', s7.name, ' to ', d2.d_code, ', printRoster follows: ') d2.addStudent(s6) d2.addStudent(s7) d2.printRoster() print(' Adding ', s8.name, ' and ', s9.name, ' to ', d3.d_code, ', printRoster follows: ') d3.addStudent(s8) a, b = d3.addStudent(s9) print(' Attempting to add qualified student , ', s9.name, ' to ', d3.d_code, ', CHHS, return values; ', a, b) d3.printRoster() a, b = d3.addStudent(s14) print(' Attempting to add low GPA student ', s14.name, ', return values: ', a, b) a, b = d2.addStudent(s13) print(' Attempting to add non-enrolled student ', s13.name, ', return values: ', a, b) a, b = d3.addStudent(s8) print(' Attempting to add dupe student ', s8.name, ', return values: ', a, b) print(' Adding s10 to ENGR, s11 to ARTS, s12 to CHHS, then print all 3 roster') d1.addStudent(s10) d2.addStudent(s11) d3.addStudent(s12) d1.printRoster() d2.printRoster() d3.printRoster() print(' ***** End of Student class demo **********')
In this assignment you will modify the Department and Student Classes in A3 to use inheritance. Specifically, a generic Person' class will be created to hold basic things about a person. The Student class, and a new class named Faculty', will be created as subclasses to Person. Person will have the following attribules and Attribute Definition number identifier individual's name individual's address individual's t individual's email address str one number email str Method Name Pu Output/returns Constructor sets initial attribute values in above table Displays a readable version of Person: Person: +g num+name + address inst See Person data as a ble strin samePerson "self Person's g num and name Person True if g num and ompares with the input Person object-returns True if match, otherwise returns Fal object name match, otherwise False Student and Faculty will inherit the above and be defined as follows: Student: inherits all Person attributes, but adds status, major, enrolled, credits, and qpoints (same as A2) Faculty: inherits all Person attributes, but adds rank, active, teach load, specialty, funding Student and Faculty both inherit the new samePerson method that does exactly what same Student does, except for a generic Person object Student uses the Person constructor, but adds its own attribute definitions as defined in A2, specifically major, enrolled, credits, and qpoints. Faculty uses the Person constructor, but adds its own attribute definitions as follows: Type str str int str aAttr Definition rank teach load fund in valid: Professor, Associate Professor, Assistant Professor, Instructor the faculty member active number of credit hours ta valid: teach dollar amount of s range 0 to 24 research, administration, combination re search, range 0 to 10 million Student and Faculty both override the tr method as follows: Student returns the string Sudent:g num+name+status +major Faculty retums the string Faculty: +name+rank+specialty Department must be modified to track the roster of students and faculty and implement a separate addFaculty method. It will also use the samePerson) method instead of sameStudentO It is permissible to define two rosters, one each for faculty and students, and an addFaculty method apart from the addStudent method However, use your best judgment as to how to implement these. The printRoster' method can be made generic by adding a parameter, 's'or, to detemine which roster to printStep by Step Solution
There are 3 Steps involved in it
Step: 1
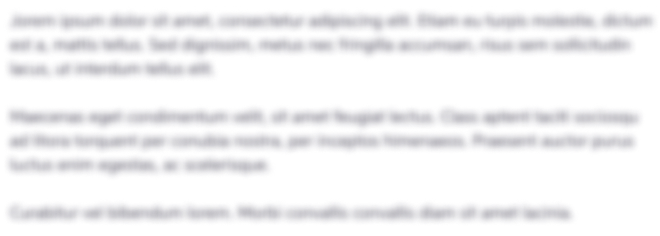
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started