Question
This is the code for 9.23; please make changes to the code how 9.24 asks. C++ using 3 file architecture. /** C++program that creates a
/**
C++program that creates a deck of 52 cards
and shuffle the cards and decal 52 cards to console.
*/
//include header files
#include
#include
#include
//include Card and DeckOfCards header files
#include "Card.h"
#include "DeckOfCards.h"
using namespace std;
int main()
{
//seed a random generator
srand(time(0));
//object of DeckOfCards class
DeckOfCards myDeckOfCards;
//calling shuffle to shuffle the deck
myDeckOfCards.shuffle();
// print the cards of deal
for ( int i = 0; i
{
//print four cards
cout
}
//pause program output on console
system("pause");
return 0;
} // end main
-----------------------------------------------------------------
//Card.h
#ifndef CARD_H
#define CARD_H
#include
#include
using namespace std;
//Card declaration
class Card
{
private:
string face;
string suit;
public:
Card(){}
Card( string face, string suit )
{
this->face = face;
this->suit = suit;
}
string toString()
{
return face + " of " + suit;
}
};
#endif CARD_H
-----------------------------------------------------------------
#ifndef DECK_OF_CARDS_H
#define DECK_OF_CARDS_H
#include
#include
#include
#include
#include "Card.h"
using namespace std;
//set constant for number of cards
const int NUMBER_OF_CARDS = 52;
class DeckOfCards
{
private:
//array of type Card of size,NUMBER_OF_CARDS
Card deck[NUMBER_OF_CARDS];
int currentCard;
public:
DeckOfCards()
{
//array of face
string faces[] = {
"Ace", "Deuce", "Three",
"Four", "Five", "Six",
"Seven", "Eight", "Nine",
"Ten", "Jack", "Queen", "King" };
//array of suit
string suits[] = { "Hearts", "Diamonds", "Clubs", "Spades" };
currentCard = 0;
for ( int pos = 0; pos
deck[ pos ] = Card( faces[ pos % 13 ], suits[ pos / 13 ] );
}
//Method to shuffle the deck
void shuffle()
{
currentCard = 0;
//shuffle the 52 cards with a random number
for ( int first = 0; first
{
// select a random number between 0 and 51
int second = rand()%( NUMBER_OF_CARDS);
//swap cards
Card temp = deck[ first ];
deck[ first ] = deck[ second ];
deck[ second ] = temp;
}
}
//Method to deal a card and return a new deal card
Card dealCard()
{
if ( currentCard
return deck[ currentCard++ ];
else
//return empty construtor
return Card();
}
};
#endif DECK_OF_CARDS_H
-----------------------------------------------------------------
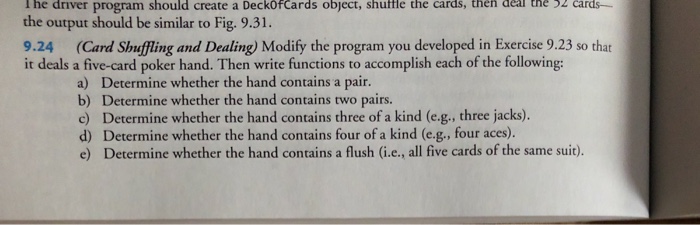
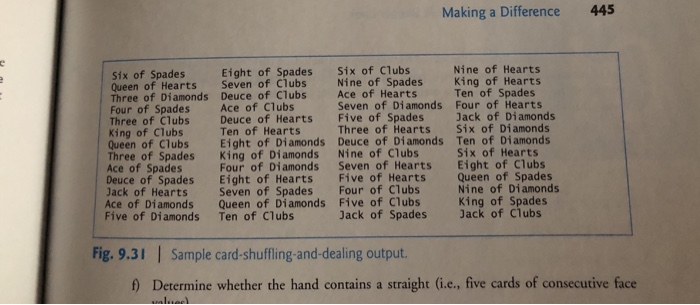
Step by Step Solution
There are 3 Steps involved in it
Step: 1
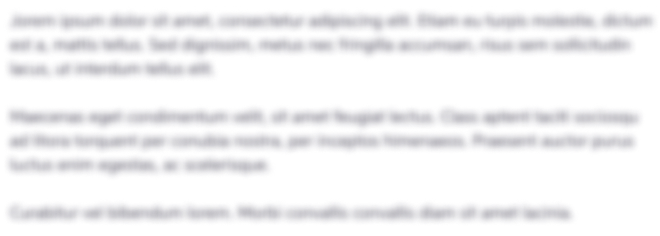
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started