Question
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// This is the code for my implementation of a singly linked list, I have done most of it I only need help with the
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
This is the code for my implementation of a singly linked list, I have done most of it I only need help with the last three method ( i had added comments with what the methods supposed to do).
Please use the same names and other methods in the class if needed.
Please add comments to explain the code and solution
Thank you!
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
public class SinglyLinkedList> implements List { private static class Node { private E value; private Node next; private Node(E value, Node next) { this.value=value; this.next=next; } } private Node head; public SinglyLinkedList() { head=null; } public int size() { //returns the size of the list Node p = head; int counter=0; while(p!=null) { counter++; p=p.next; } return counter; } public T get(int i) { //returns the element at position i Pre-Condition: first position index is 0. if (i<0) { return null; } else { Node p=head; int pos=0; while(pos p = new Node(item, null); //Create a new node; if(head==null && i!=0) { //if head is null and position is zero skip it. return; } else if (head==null && i==0){ // if head null and position is zero set at the head. head=p; } if (i==0) { // if position is zero then new node set at the head. p.next=head; head=p; } Node now = head; //Creating new node and setting it to head; Node pre = null; //Creating new node and setting it to null int x=0; while(x<0) { //loop until find the given position. pre = now; now = now.next; if (now ==null) { break; } x++; } p.next=now; //get the new node and linked the remaining nodes in the list. pre.next=p; //set the looped node to the new node. } public T remove(int i) { //removes item at position i of the list. T saved; Node remove; if (i==0) { remove = head; head = head.next; } else { Node p = head; for (int q=0; i<(i-1); q++) { p = p.next; } remove = p.next; p.next= p.next.next; } saved = remove.value; remove.value = null; remove.next = null; return saved; } public T min() { //returns the item with the minimum value in the list; T min=head.value; while(head!=null) { if(min.compareTo(head.next.value)==1) { min=head.next.value; } head=head.next; } return min; } public T minR() { //returns the item with the minimum value in the list; (implemented using a recursive method) if (head==null) { return null; } else { return minR(head); } } private T minR(Node p) { //returns the item with the minimum value in the list; (implemented using a recursive method) if(p.next==null) { return p.value; } else { T min = minR(p.next); int test =p.value.compareTo(min); if (test<0) { return p.value; } else { return min; } } } public T max() { //returns the item with the maximum value in the list; T max=head.value; while(head!=null) { if(max.compareTo(head.next.value)==-1) { max=head.next.value; } head=head.next; } return max; } public T maxR() { //returns the item with the maximum value in the list; (implemented using a recursive method) if (head==null) { return null; } else { return maxR(head); } } private T maxR(Node p) { //returns the item with the maximum value in the list; (implemented using a recursive method) if(p.next==null) { return p.value; } else { T max = maxR(p.next); int test =p.value.compareTo(max); if (test>0) { return p.value; } else { return max; } } } public boolean Empty() { //returns true if the list is empty. and false otherwise; return head==null; } public void addAthead(T item) { //adds an element at the head of the list" head= new Node (item,head); } public void addAtEnd(T item) { //adds an element at the end of the list Node p = new Node (item,null); if( head==null) { head=p; } else { Node q= head; while(q.next!=null) { q=q.next; } q.next= p; } } public void replace(T first, T second) { //replaces the element first (if found in list) with element second; int i = this.indexOf(first); int pos=0; while(pos duplicate(T item){ //duplicate every element in a linked list, that is equal to a certain value. return duplicate(item, head); } private List duplicate(T item, Node p){ //duplicate every element in a linked list, that is equal to a certain value. List list = new SinglyLinkedList(); if (p==null) { List list2 = new SinglyLinkedList(); return list2; } else { if(p.value==item) { list=duplicate(item,p.next); list.addAtEnd(p.value); list.addAtEnd(p.value); return list; } else { list=duplicate(item,p.next); list.addAtEnd(p.value); return list; } } } public void reverse() { //prints out the data elements of a linked list in reverse order. System.out.println("["+reverse(head)+"]"); } private String reverse(Node p) { //prints out the data elements of a linked list in reverse order. if (p==null) { return ""; } else { return head.value+","+reverse(p.next); } } public List countGreaterThan(T threshold){ //returns a list containing all the elements in the original list that are larger than threshold. return countGreaterThan(threshold, head); } private List countGreaterThan(T threshold, Node p){ //returns a list containing all the elements in the original list that are larger than threshold. List list = new SinglyLinkedList(); if (p==null) { List list2 = new SinglyLinkedList(); return list2; } else { if(p.value.compareTo(threshold)==-1) { list=countGreaterThan(threshold, p.next); list.addAtEnd(p.value); return list; } else { list=countGreaterThan(threshold, p.next); return list; } } } public String toString() { //returns a string representation of the elements in the list separated by a comma. String s= "("; Node p=head; while(p!=null) { s=s+" "+p.value; p=p.next; } return s; } public void removeEven() { //removes all the elements at even positions from the list int i= this.size(); int counter=0; while (counter inorder() { //returns a new list that has all the elements in the list sorted in an ascending order. } public boolean equals(Object other) { //returns true if other is list that has the same elements in the list with the same order. } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
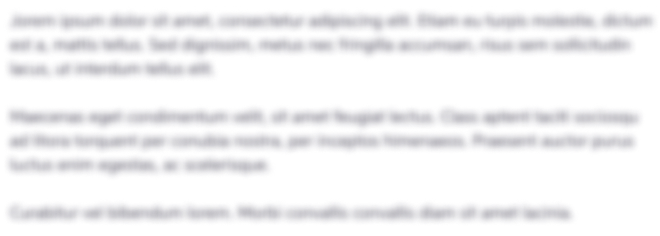
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started