Question
This is the code of PointCP : public class PointCP { //Instance variables ************************************************ /** * Contains C(artesian) or P(olar) to
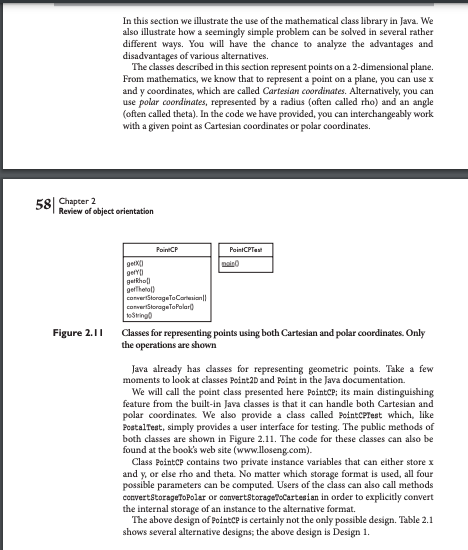
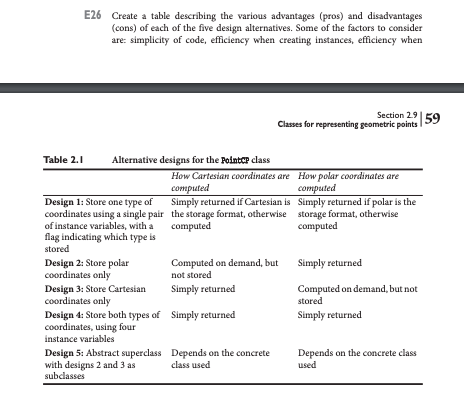
This is the code of PointCP :
public class PointCP
{
//Instance variables ************************************************
/**
* Contains C(artesian) or P(olar) to identify the type of
* coordinates that are being dealt with.
*/
private char typeCoord;
/**
* Contains the current value of X or RHO depending on the type
* of coordinates.
*/
private double xOrRho;
/**
* Contains the current value of Y or THETA value depending on the
* type of coordinates.
*/
private double yOrTheta;
//Constructors ******************************************************
/**
* Constructs a coordinate object, with a type identifier.
*/
public PointCP(char type, double xOrRho, double yOrTheta)
{
if(type != 'C' && type != 'P')
throw new IllegalArgumentException();
this.xOrRho = xOrRho;
this.yOrTheta = yOrTheta;
typeCoord = type;
}
//Instance methods **************************************************
public double getX()
{
if(typeCoord == 'C')
return xOrRho;
else
return (Math.cos(Math.toRadians(yOrTheta)) * xOrRho);
}
public double getY()
{
if(typeCoord == 'C')
return yOrTheta;
else
return (Math.sin(Math.toRadians(yOrTheta)) * xOrRho);
}
public double getRho()
{
if(typeCoord == 'P')
return xOrRho;
else
return (Math.sqrt(Math.pow(xOrRho, 2) + Math.pow(yOrTheta, 2)));
}
public double getTheta()
{
if(typeCoord == 'P')
return yOrTheta;
else
return Math.toDegrees(Math.atan2(yOrTheta, xOrRho));
}
/**
* Converts Cartesian coordinates to Polar coordinates.
*/
public void convertStorageToPolar()
{
if(typeCoord != 'P')
{
//Calculate RHO and THETA
double temp = getRho();
yOrTheta = getTheta();
xOrRho = temp;
typeCoord = 'P'; //Change coord type identifier
}
}
/**
* Converts Polar coordinates to Cartesian coordinates.
*/
public void convertStorageToCartesian()
{
if(typeCoord != 'C')
{
//Calculate X and Y
double temp = getX();
yOrTheta = getY();
xOrRho = temp;
typeCoord = 'C'; //Change coord type identifier
}
}
/**
* Calculates the distance in between two points using the Pythagorean
* theorem (C ^ 2 = A ^ 2 + B ^ 2). Not needed until E2.30.
*
* @param pointA The first point.
* @param pointB The second point.
* @return The distance between the two points.
*/
public double getDistance(PointCP pointB)
{
// Obtain differences in X and Y, sign is not important as these values
// will be squared later.
double deltaX = getX() - pointB.getX();
double deltaY = getY() - pointB.getY();
return Math.sqrt((Math.pow(deltaX, 2) + Math.pow(deltaY, 2)));
}
/**
* Rotates the specified point by the specified number of degrees.
* Not required until E2.30
*
* @param point The point to rotate
* @param rotation The number of degrees to rotate the point.
* @return The rotated image of the original point.
*/
public PointCP rotatePoint(double rotation)
{
double radRotation = Math.toRadians(rotation);
double X = getX();
double Y = getY();
return new PointCP('C',
(Math.cos(radRotation) * X) - (Math.sin(radRotation) * Y),
(Math.sin(radRotation) * X) + (Math.cos(radRotation) * Y));
}
/**
* Returns information about the coordinates.
*
* @return A String containing information about the coordinates.
*/
public String toString()
{
return "Stored as " + (typeCoord == 'C'
? "Cartesian (" + getX() + "," + getY() + ")"
: "Polar [" + getRho() + "," + getTheta() + "]") + "\n";
}
}
58 In this section we illustrate the use of the mathematical class library in Java. We also illustrate how a seemingly simple problem can be solved in several rather different ways. You will have the chance to analyze the advantages and disadvantages of various alternatives. Figure 2.11 The classes described in this section represent points on a 2-dimensional plane. From mathematics, we know that to represent a point on a plane, you can use x and y coordinates, which are called Cartesian coordinates. Alternatively, you can use polar coordinates, represented by a radius (often called rho) and an angle (often called theta). In the code we have provided, you can interchangeably work with a given point as Cartesian coordinates or polar coordinates. Chapter 2 Review of object orientation get() gery) |getRhe{] gefthenol) Point CP convertStorageToCartesion convertStorageToPolar) PointCPTast main toString Classes for representing points using both Cartesian and polar coordinates. Only the operations are shown Java already has classes for representing geometric points. Take a few moments to look at classes Point2D and Point in the Java documentation. We will call the point class presented here PointCP; its main distinguishing feature from the built-in Java classes is that it can handle both Cartesian and polar coordinates. We also provide a class called Point Prest which, like PostalTest, simply provides a user interface for testing. The public methods of both classes are shown in Figure 2.11. The code for these classes can also be found at the book's web site (www.lloseng.com). Class PointCP contains two private instance variables that can either store x and y, or else rho and theta. No matter which storage format is used, all four possible parameters can be computed. Users of the class can also call methods convertStorageToPolar or convertStoragefoCartesian in order to explicitly convert the internal storage of an instance to the alternative format. The above design of PointCP is certainly not the only possible design. Table 2.1 shows several alternative designs; the above design is Design 1.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
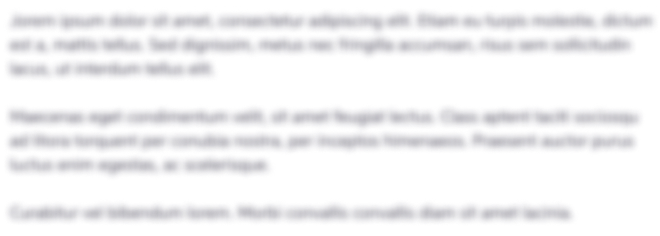
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started