Question
This is the Game of Life in JAVA. Here's a link to get a feel of what the game of life is https://bitstorm.org/gameoflife/ 1) Using
This is the Game of Life in JAVA.
Here's a link to get a feel of what the game of life is https://bitstorm.org/gameoflife/
1) Using the Java Swing API, create a graphical user interface for your Game of Life application.
2) Only the GameOfLife.java and the GoLDisplay.java should have to change.
3) Add/update Javadoc comments as needed and re-generate Javadoc documentation for the program using the Javadoc utility
*******GAME OF LIFE***********
import java.io.*; import java.util.Scanner;
public class GameOfLife {
/** * Creates a new instance of GameOfLife
. */ public GameOfLife() { }
/** * @param args the command line arguments */ public static void main(String[] args) { // Create objects required for the game loop GoLBoard myBoard = new GoLBoard(); GoLDisplay out = new GoLDisplay(); Scanner in = new Scanner(System.in); if (args.length > 0) { if (args[0].equals("extended")) { myBoard = new GoLBoard(new GoLExtRandomInitializer()); } else { myBoard = new GoLBoard(new GoLRandomInitializer()); } } else { myBoard = new GoLBoard(new GoLRandomInitializer()); }
out.displayBoard(myBoard);
while (in.next().charAt(0)=='n'){
myBoard.nextRound(); out.displayBoard(myBoard);
} } }
******GameOfLIfeBoard*********
//Implements the functions of the Game of Life board public class GoLBoard {
//Internal storage for board data protected GoLCell[][] boardData;
private int births; //counters for births, deaths and rounds private int deaths; // private int rounds = 0; //
//Default constructor; uses the RandomInitializer public GoLBoard() {
this(new GoLRandomInitializer());
}
//Constructor that allows an arbitrary initializer public GoLBoard(GoLInitializer myInitializer) {
boardData = myInitializer.getCellArray(); }
//Reset the board public GoLBoard reset() {
return reset(new GoLRandomInitializer());
}
//Reset with an arbitrary initializer public GoLBoard reset(GoLInitializer myInitializer) {
boardData = myInitializer.getCellArray(); return this; }
//Updates the board for the next round by calling the //updateCell method of the individual cells, passing a //GoLNeighborhood to them. Returns the updated board. public GoLBoard nextRound() {
GoLBoard tempBoard = this.copyBoard(); births = 0; deaths = 0;
for (int i=0; i<20; i++) {
for (int j=0; j<20; j++) {
boolean wasAlive = boardData[i][j].isAlive(); boolean nowAlive = tempBoard.getCell(i,j).updateCell(getNeighborhood(i,j)); deaths += (wasAlive && !nowAlive)?1:0; births += (!wasAlive && nowAlive)?1:0; }
}
this.boardData = tempBoard.boardData; rounds++; return this; }
//Gets a cell from the board public GoLCell getCell(int x, int y) {
return boardData[x][y];
}
//Copies the current board public GoLBoard copyBoard() {
GoLBoard tempBoard = new GoLBoard(); for (int i=0; i<20; i++) {
for (int j=0; j<20; j++) {
tempBoard.boardData[i][j] = new GoLCell(this.boardData[i][j].isAlive()); }
} return tempBoard;
}
public int getCurrentRound() { return rounds; }
public int getBirths() { return births; }
public int getDeaths() { return deaths; }
//private utility function to generate the neigborhoods private GoLNeighborhood getNeighborhood(int x, int y) {
GoLCell[][] tempArray = new GoLCell[3][3];
tempArray[0][0] = ((x>0)&&(y>0))?boardData[x-1][y-1]:(new GoLCell(false)); tempArray[1][0] = (y>0)?boardData[x][y-1]:(new GoLCell(false)); tempArray[2][0] = ((x<19)&&(y>0))?boardData[x+1][y-1]:(new GoLCell(false)); tempArray[0][1] = ((x>0))?boardData[x-1][y]:(new GoLCell(false)); tempArray[1][1] = boardData[x][y]; tempArray[2][1] = ((x<19))?boardData[x+1][y]:(new GoLCell(false)); tempArray[0][2] = ((x>0)&&(y<19))?boardData[x-1][y+1]:(new GoLCell(false)); tempArray[1][2] = (y<19)?boardData[x][y+1]:(new GoLCell(false)); tempArray[2][2] = ((x<19)&&(y<19))?boardData[x+1][y+1]:(new GoLCell(false));
return new GoLNeighborhood(tempArray);
}
}
******GameOfLifeCell**********
//Implements the business logic for an individual cell in the Game of Life
public class GoLCell {
//Private variable to hold the cell state private boolean cellState;
//Constructor: Takes the initial state of the cell as an argument public GoLCell(boolean cellState) {
//Sets initial state to value passed to constructor this.cellState = cellState;
}
//Method updates the state of the cell based on the provided GoLNeigborhood public boolean updateCell(GoLNeighborhood myNeighborhood){
//Add up the number of live neighbors int liveNeighbors = (myNeighborhood.getCell(0,0).isAlive()?1:0) + (myNeighborhood.getCell(1,0).isAlive()?1:0) + (myNeighborhood.getCell(2,0).isAlive()?1:0) + (myNeighborhood.getCell(0,1).isAlive()?1:0) + (myNeighborhood.getCell(2,1).isAlive()?1:0) + (myNeighborhood.getCell(0,2).isAlive()?1:0) + (myNeighborhood.getCell(1,2).isAlive()?1:0) + (myNeighborhood.getCell(2,2).isAlive()?1:0);
if ((liveNeighbors == 3) || ((liveNeighbors == 2) && cellState)) {
//The cell is alive cellState = true;
} else {
//The cell is dead cellState = false; }
return cellState;
}
//accessor for the state of the cell public boolean isAlive(){
return cellState; } }
******GameOfLifeDisplay*******
import java.io.*;
public class GoLDisplay {
public GoLDisplay() { }
public void displayBoard(GoLBoard myBoard) {
System.out.println("Round: " + myBoard.getCurrentRound() + " Births: " + myBoard.getBirths() + " Deaths: " + myBoard.getDeaths()); System.out.println("------------------------------------------------------");
for (int i=0; i<20; i++) {
String tempStr = "";
for (int j=0; j<20; j++) {
if (myBoard.getCell(i,j).isAlive()) {
tempStr = tempStr + "O ";
} else {
tempStr = tempStr + " "; } }
System.out.println(tempStr); }
}
}
********GameOfLifeExtRandomInitializer*******
import java.util.*;
public class GoLExtRandomInitializer implements GoLInitializer {
//Instance Variables private final int NUM_ROWS = 20; private final int NUM_COLUMNS = 20; private GoLCell[][] gameBoard = new GoLCell[NUM_ROWS][NUM_COLUMNS];
//Constructor
//Creates new GoLExtRandomInitializer with a random seed public GoLExtRandomInitializer() { Random randomLifeOrDeath = new Random(); Random randomCellType = new Random(); int cellType = -1;
//Give life to random cells in the board for (int row = 0; row < this.NUM_ROWS; row++) { for (int column = 0; column < this.NUM_COLUMNS; column++) { cellType = randomCellType.nextInt(3); if (cellType == 0) this.gameBoard[row][column] = new GoLCell(randomLifeOrDeath.nextBoolean()); if (cellType == 1) this.gameBoard[row][column] = new GoLStrongCell(randomLifeOrDeath.nextBoolean()); if (cellType == 2) this.gameBoard[row][column] = new GoLWeakCell(randomLifeOrDeath.nextBoolean()); } } }
public GoLExtRandomInitializer(long seed) { Random randomLifeOrDeath = new Random(seed); Random randomCellType = new Random(seed); int cellType = -1;
//Give life to random cells in the board for (int row = 0; row < this.NUM_ROWS; row++) { for (int column = 0; column < this.NUM_COLUMNS; column++) { cellType = randomCellType.nextInt(3); if (cellType == 0) { this.gameBoard[row][column] = new GoLCell(randomLifeOrDeath.nextBoolean()); } else if (cellType == 1) { this.gameBoard[row][column] = new GoLStrongCell(randomLifeOrDeath.nextBoolean()); } else if (cellType == 2) { this.gameBoard[row][column] = new GoLWeakCell(randomLifeOrDeath.nextBoolean()); } else { System.out.println("error!"); } } } }
@Override public GoLCell[][] getCellArray() { // TODO Auto-generated method stub return null; } }
******GameOfLifeInitializer*********
//Interface for initializing the board public interface GoLInitializer {
//Gets the initial cell array to initialize the board public GoLCell[][] getCellArray(); }
******GameOfLIfeNeighborhood*********
//Implements the concept of a cell neighborhood public class GoLNeighborhood {
//hold the neigborhood private GoLCell[][] cellArray;
//Constructor: sets the neigborhood public GoLNeighborhood(GoLCell[][] cellNeighbors) {
cellArray = cellNeighbors;
}
//retrieves a cell from the neigborhood public GoLCell getCell(int x, int y) {
return cellArray[x][y];
}
}
*******GameOfLIfeRandomInitializer********
import java.util.Random;
//Randomly initializes a board public class GoLRandomInitializer implements GoLInitializer {
//Stores the randomized contents private GoLCell[][] myBoard = new GoLCell[20][20];
//Creates a new set of board data using a random seed public GoLRandomInitializer() {
//Gets a new RNG Random myRNG = new Random();
//Loops through the array of data and randomly creates a cell for (int i = 0; i<20; i++) { for (int j = 0; j<20; j++) {
myBoard[i][j] = new GoLCell(myRNG.nextBoolean());
}
}
}
//Creates a new set of board data using a given long seed public GoLRandomInitializer(long seed) {
//Gets a new RNG Random myRNG = new Random(seed);
//Loops through the array of data and randomly creates a cell for (int i = 0; i<20; i++) { for (int j = 0; j<20; j++) {
myBoard[i][j] = new GoLCell(myRNG.nextBoolean());
}
}
}
public GoLCell[][] getCellArray() {
return myBoard; }
}
**********GameOfLifeStrong Cell**********
//GoLStrongCell.java import java.util.*;
public class GoLStrongCell extends GoLCell {
//Constructor public GoLStrongCell(boolean cellStatus) { super(cellStatus); }
//Method
//Updates cell using myNeighborhood and returns true if alive and false if dead public boolean updateCell(GoLNeighborhood myNeighborhood) { boolean stateOfCell = false; int numAlive = 0;
for (int row = 0; row < 3; row++) { for (int column = 0; column < 3; column++) { try { if (myNeighborhood.getCell(row, column).isAlive()) numAlive++; } catch (NullPointerException e) {} } }
//Check current state of cell stateOfCell = myNeighborhood.getCell(1, 1).isAlive();
//Apply rules to neighbors if (stateOfCell) { numAlive--; if (numAlive < 0 || numAlive > 5) stateOfCell = false; } else { if (numAlive == 2 || numAlive == 3) stateOfCell = true; }
return stateOfCell; } }
********GameOfLifeWeakCell*********
import java.util.*; public class GoLWeakCell extends GoLCell { // Constructor public GoLWeakCell(boolean cellStatus) { super(cellStatus); } // Method // Updates cell using myNeighborhood and returns true if alive and false if dead public boolean updateCell(GoLNeighborhood myNeighborhood) { boolean stateOfCell = false; int numAlive = 0;
for (int row = 0; row < 3; row++) { for (int column = 0; column < 3; column++) { try { if (myNeighborhood.getCell(row, column).isAlive()) numAlive++; } catch (NullPointerException e) {} } }
// Checks current state of cell stateOfCell = myNeighborhood.getCell(1, 1).isAlive();
// Apply rules to neighbors if (stateOfCell) { numAlive--; if (numAlive != 3 || numAlive != 4) stateOfCell = false; } else { if (numAlive == 4) stateOfCell = true; }
return stateOfCell; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
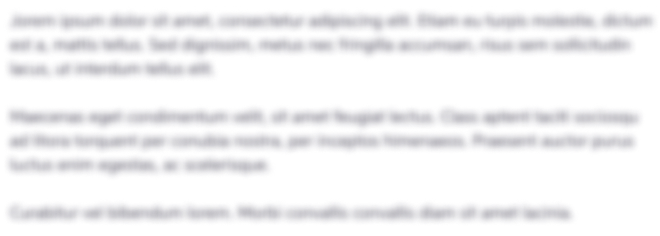
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started