Question
This is what I have for the Transaction class: public class Transaction { private String date; private double amount; private String description; public Transaction(String a,
This is what I have for the Transaction class:
public class Transaction { private String date; private double amount; private String description; public Transaction(String a, double b, String c ){ date = a; amount = b; description = c; } public String getDate(){ return date; } public double getAmount() { return amount; } public String getDescription(){ return description; } @Override public boolean equals(Object obj) { if(((Transaction) obj).getAmount()==amount&&((Transaction) obj).getDate().equals(date)&&((Transaction) obj).getDescription().equals(description)) { return true;} else{ return false; } } public Object clone(){ Transaction trans= new Transaction(date, amount, description); return trans; } public boolean earlyDate(Transaction x){ String year = x.getDate().substring(0,4); String month=x.getDate().substring(5,7); String day= x.getDate().substring(8,10); int y =Integer.parseInt(year); int m = Integer.parseInt(month); int d = Integer.parseInt(day); String year1 = this.getDate().substring(0,4); String month1 =this.getDate().substring(5,7); String day1 = this.getDate().substring(8,10); int y1 =Integer.parseInt(year1); int m1 = Integer.parseInt(month1); int d1 = Integer.parseInt(day1); if (y < y1){ return true; } else if (y == y1){ if (m < m1){ return true; } else if (m == m1){ if (d < d1){ return true; } } } return false; } }
-----------------------------------------------------------------------------------------------------
Write a fully-documented class named GeneralLedger that stores an ordered list of transaction objects within an array. The array indices determine the order the transactions are to be posted. An accountant can insert a transaction at any position within the range of the list. The GeneralLedger can record a total of 50 transactions, so use the static final variable int MAX_TRANSACTIONS = 50 (and refrain from using the number 50 within your code). Additionally, create variables of type double "totalDebitAmount" and "totalCreditAmount" to keep track of the running total of all debit and credit amounts for all transactions. These variables should be modified every time a transaction is added or deleted from the general ledger. For example, if Jason's general ledger is empty, "totalDebitAmount" and "totalCreditAmount" should both be initialized to 0. After a single transaction is added with amount value 50, meaning $50 is debited to his account, the value of "totalDebitAmount" should now be 50 and "totalCreditAmount" should now be 0.
NOTE: Make sure there are no gaps between Transactions in the list.
NOTE: Note that arrays in Java are 0 indexed, but your listing preference should start from 1.
The GeneralLedger class implements an abstract data type for a list of Transactions supporting some common operations on such lists. The GeneralLedger class will be based on the following ADT specifications: public class GeneralLedger
- public GeneralLedger
- Brief:
- Constructs an instance of the GeneralLedger with no Transaction objects in it.
- Postconditions:
- This GeneralLedger has been initialized to an empty list of Transactions.
- Brief:
- public void addTransaction(Transaction newTransaction)
- Brief:
- Adds newTransaction into this GeneralLedger if it does not already exist. Added entries should be inserted in date order. If there are multiple entries with the same date, new entries should be placed at the end of that date's boundary.
- Parameters:
- newTransaction - the new Transaction to add to the ledger
- Preconditions:
- The Transaction object has been instantiated and the number of Transaction objects in this GeneralLedger is less than MAX_TRANSACTIONS.
- Postconditions:
- The new transaction is now listed in the correct order in the list. All Transactions whose date is newer than or equal to newTransaction are moved back one position (e.g. If there are 5 transactions in a generalLedger, positioned 1-5, and the transaction you insert has a date that's newer than the transaction in position 3 but older than the transaction in position 4, the new transaction would be placed in position 4, the transaction that was in position 4 will be moved to position 5, and the transaction that was in position 5 will be moved to position 6.) (See sample output for more information).
- Throws:
- FullGeneralLedgerException - Thrown if there is no more room in this GeneralLedger to record additional transactions.
- InvalidTransactionException - Thrown if the transaction amount is 0 or if the date is invalid.
- TransactionAlreadyExistsException - Thrown if there is already a transaction in this GeneralLedger which is equivalent to newTransaction (both have the same date, amount, and description).
- NOTE: Remember that the format for inputting the date is yyyy/mm/dd.
- NOTE: Inserting an item with a newer date than any of the other items in the list is effectively the same as adding the item to the end of the list.
- Brief:
- public void removeTransaction(int position)
- Brief:
- Removes the transaction located at position from this GeneralLedger.
- Parameters:
- position - The 1-based index of the Transaction to remove
- Preconditions:
- This generalLedger has been instantiated and 1 <= position <= items_currently_in_list.
- Postconditions:
- The Transaction at the desired position has been removed. All transactions that were originally greater than or equal to position are moved backward one position (e.g. If there are 5 Transactions in the generalLedger, positioned 1-5, and you remove the Transaction in position 4, the item that was in position 5 will be moved to position 4).
- Throws:
- InvalidLedgerPositionException - Thrown if position is not valid.
- NOTE: position refers to the position in the general ledger, not the position in the array.
- Brief:
- public Transaction getTransaction(int position)
- Brief:
- Returns a reference to the Transaction object located at position.
- Parameters:
- position - The position in this GeneralLedger to retrieve.
- Preconditions:
- The GeneralLedger has been instantiated and 1 <= position <= items_currently_in_list.
- Returns:
- The Transaction at the specified position in this GeneralLedger object.
- Throws:
- InvalidLedgerPositionException - Indicates that position> is not within the valid range.
- NOTE: position refers to the position in the general ledger, not the position in the array.
- Brief:
- public static void filter(GeneralLedger generalLedger, String date)
- Brief:
- Prints all transactions that were posted on the specified date.
- Parameters:
- generalLedger - The ledger of transactions to search in.
- date - The date of the transaction(s) to search for.
- Preconditions:
- This GeneralLedger object has been instantiated.
- Postconditions:
- Displays a neatly formatted table of all Transactions that have taken place on the specified date.
- Brief:
- public Object clone()
- Brief:
- Creates a copy of this GeneralLedger. Subsequent changes to the copy will not affect the original and vice versa.
- Preconditions:
- This GeneralLedger object has been instantiated.
- Returns:
- A copy (backup) of this GeneralLedger object.
- NOTE: Remember that this is supposed to return a deep copy of the object.
- Brief:
- public boolean exists(Transaction transaction)
- Brief:
- Checks whether a certain transaction is contained in the ledger.
- Parameters:
- transaction - The Transaction to check for.
- Preconditions:
- This GeneralLedger and transaction have been instantiated.
- Returns:
- true if this GeneralLedger contains transaction, false otherwise.
- Throws:
- IllegalArgumentException - Thrown if transaction is not a valid Transaction object.
- Brief:
- public int size()
- Brief:
- Returns the number of Transactions currently in this ledger.
- Preconditions:
- This GeneralLedger has been instantiated.
- Returns:
- The number of Transactions in this ledger.
- NOTE: This method must run in O(1) time.
- Brief:
- public void printAllTransactions()
- Brief:
- Prints a neatly formatted table of each item in the list with its position number (as shown in the sample output).
- Preconditions:
- This GeneralLedger has been instantiated.
- Postconditions:
- All transactions contained within this GeneralLedger are printed in a neatly formatted table. See sample i/o for format.
- NOTE: Remember that the format for printing the date is yyyy/mm/dd.
- HINT: If your toString() method is implemented correctly, you will simply need to call it and print the results to standard output.
- Brief:
- public String toString()
- Brief:
- Returns a String representation of this GeneralLedger object, which is a neatly formatted table of each Transaction contained in this ledger on its own line with its position number (as shown in the sample output).
- Returns:
- A String representation of this GeneralLedger object.
- Brief:
- public static final int MAX_TRANSACTIONS - The maximum number of transactions a GeneralLedger can contain.
- private Transaction[] ledger - The array which will hold Transactions inserted into this GeneralLedger object.
- private double totalDebitAmount - The total debit amount for all transactions in this GeneralLedger object.
- private double totalCreditAmount - The total credit amount for all transactions in this GeneralLedger object.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
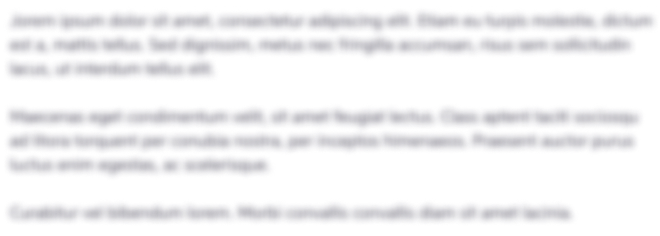
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started