Question
This is what I have so far. FullTimeEmployee Class ------------------------------------- public class FullTimeEmployee { private String name; private String idNumber; private double salary; public FullTimeEmployee(String
This is what I have so far.
FullTimeEmployee Class
-------------------------------------
public class FullTimeEmployee {
private String name;
private String idNumber;
private double salary;
public FullTimeEmployee(String n, String id, double s)
{
name = n;
idNumber = id;
salary = s;
}
public String getName()
{
return name;
}
public String getId()
{
return idNumber;
}
public double getSalary()
{
return salary;
}
public void display()
{
System.out.print(name + "\t" + idNumber + "\t$" + salary);
}
}
---------------------------------------
PartTimeEmployee Class
------------------------------------
public class PartTimeEmployee {
private String name;
private String idNumber;
private double hourlyWage;
public PartTimeEmployee(String n, String id, double hw)
{
name = n;
idNumber = id;
hourlyWage = hw;
}
public String getName()
{
return name;
}
public String getId()
{
return idNumber;
}
public double getHourlyWage()
{
return hourlyWage;
}
public void display()
{
System.out.print(name + "\t" + idNumber + "\t$" + hourlyWage);
}
}
----------------------------------------
EmployeeList class
----------------------------------------
import java.util.ArrayList;
import java.util.Iterator;
public class EmployeeList {
ArrayList
ArrayList
public EmployeeList()
{
fullTimeList = new ArrayList();
partTimeList = new ArrayList();
}
public void addFullTimeEmployee(FullTimeEmployee fte)
{
fullTimeList.add(fte);
}
public void addPartTimeEmployee(PartTimeEmployee pte)
{
partTimeList.add(pte);
}
public void removeFullTimeEmployee(String id)
{
FullTimeEmployee fte;
Iterator
boolean found = false;
while (it.hasNext() && !found)
{
fte = it.next();
if (fte.getId().equals(id))
{
it.remove();
found = true;
}
}
}
public void removePartTimeEmployee(String id)
{
PartTimeEmployee pte;
Iterator
boolean found = false;
while (it.hasNext() && !found)
{
pte = it.next();
if (pte.getId().equals(id))
{
it.remove();
found = true;
}
}
}
public void printList()
{
for (FullTimeEmployee fte: fullTimeList)
{
fte.display();
System.out.println();
}
for (PartTimeEmployee pte: partTimeList)
{
pte.display();
System.out.println();
}
}
}
-----------------------------
Driver Class
------------------------------
import java.util.Scanner;
public class Driver {
public static void main(String[] args) {
EmployeeList list = new EmployeeList();
Scanner sc = new Scanner(System.in);
boolean done = false;
int menuChoice;
String name, id, answer;
double wageInfo;
do
{
System.out.println("Enter the number of one of the following choices:");
System.out.println("1: Enter a new employee.");
System.out.println("2: Remove an employee.");
System.out.println("3: Print a list of all employees.");
System.out.println("4: Quit.");
menuChoice = sc.nextInt();
sc.nextLine();
switch (menuChoice)
{
case 1: System.out.println("Enter name");
name = sc.nextLine();
System.out.println("Enter id number");
id = sc.nextLine();
System.out.println("Is this a full time employee (Y or N)");
answer = sc.nextLine();
if (answer.equalsIgnoreCase("Y"))
{
System.out.println("Enter salary");
wageInfo = sc.nextDouble();
sc.nextLine();
list.addFullTimeEmployee(new FullTimeEmployee(name, id, wageInfo));
}
else
{
System.out.println("Enter hourly rate");
wageInfo = sc.nextDouble();
sc.nextLine();
list.addPartTimeEmployee(new PartTimeEmployee(name, id, wageInfo));
}
break;
case 2: System.out.println("Enter id number of employee:");
id = sc.nextLine();
list.removeFullTimeEmployee(id);
list.removePartTimeEmployee(id);
break;
case 3: list.printList();
break;
case 4: done = true;
System.out.println("Goodbye.");
break;
default: System.out.println("Invalid menu choice.");
}
}
while (!done);
sc.close();
}
}
In this assignment you will explore inheritance, subtyping, polymorphic variables, method overriding and dynamic method lookup. Download the Employee Project supplied with this assignment. This project contains the following: A FullTimeEmployee class. A Part TimeEmployee class. An EmployeeList class. A Driver class that does the following: O Display a menu of choices as follows: 1. Enter a new employee. 2. Remove an employee from list by ID number. 3. Print a list of all employees. 4. Quit o The user can then enter a choice (1,2,3,4) o The program will carry out that option. o The program will terminate only when the user selects choice 4. Noticing the duplication involved in the two employee classes given above, you will use inheritance as fully as possible as discussed in chapters 10 and 11. This means you will: Create a class hierarchy. Avoid code duplication. Make the project extendible. Use super call in constructors. Use super call in methods (if necessary). Use method overriding. Override the Object class toString method (instead of using a display method). Be sure to remove all duplication, including the EmployeeList and Driver classes Be sure to add ALL necessary documentation. In this assignment you will explore inheritance, subtyping, polymorphic variables, method overriding and dynamic method lookup. Download the Employee Project supplied with this assignment. This project contains the following: A FullTimeEmployee class. A Part TimeEmployee class. An EmployeeList class. A Driver class that does the following: O Display a menu of choices as follows: 1. Enter a new employee. 2. Remove an employee from list by ID number. 3. Print a list of all employees. 4. Quit o The user can then enter a choice (1,2,3,4) o The program will carry out that option. o The program will terminate only when the user selects choice 4. Noticing the duplication involved in the two employee classes given above, you will use inheritance as fully as possible as discussed in chapters 10 and 11. This means you will: Create a class hierarchy. Avoid code duplication. Make the project extendible. Use super call in constructors. Use super call in methods (if necessary). Use method overriding. Override the Object class toString method (instead of using a display method). Be sure to remove all duplication, including the EmployeeList and Driver classes Be sure to add ALL necessary documentationStep by Step Solution
There are 3 Steps involved in it
Step: 1
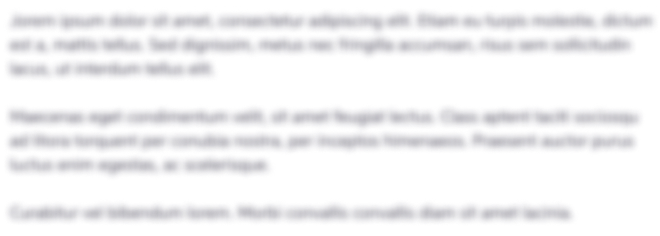
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started